How to Make a CURL Call in C#
-
Use
HttpWebRequest
orHttpWebResponse
to Make a CURL Call inC#
-
Use
WebClient
to Make a CURL Call inC#
-
Use
HttpClient
to Make a CURL Call inC#
- Conclusion
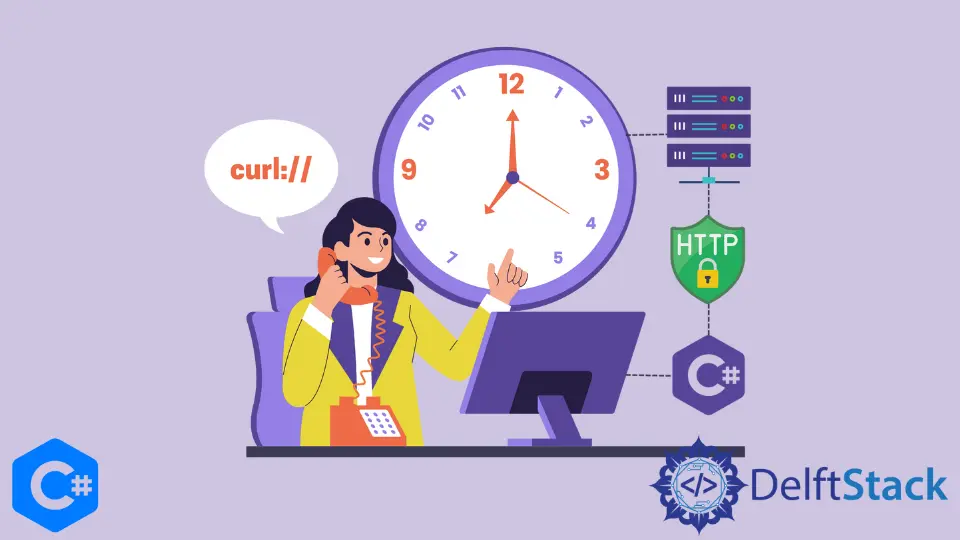
Client URL (cURL) is the de facto standard for the API docs in C#. It requires conversion from one format to another.
Hence, transforming JSON into a C# class is a reasonably popular approach. The response object from the web or host also is converted into a C# class to make it more developer-friendly.
This article will discuss three approaches to making a cURL call in C#.
Create a communication with the server by specifying the location and the data you want to send using cURL in C#. As the URL follows every cURL command, it always retrieves some data and returns the HTML source for a cURL.
cURL is highly portable and compatible with almost every OS and connected device. In C#, cURL is useful for testing endpoints and provides details of exactly what has been sent or received, which is good for error logging and debugging.
Since cURL uses libcurl
, it supports the same range of common internet protocols that libcurl
does.
Use HttpWebRequest
or HttpWebResponse
to Make a CURL Call in C#
The HttpWebRequest
class supports the methods and properties defined in WebRequest
. Its additional properties and methods enable the users to interact directly with servers using HTTP.
Use the WebRequest.Create
method to initialize new HttpWebRequest
objects. Use the GetResponse
method to make a synchronous request to the resource specified in the RequestUri
property.
It returns an HttpWebResponse
that contains the response object. In the case of a closed response object, the remaining data will be fortified.
It’s a keep-alive or pipelined request; only a small amount of data needs to be received in short intervals. In C#, the .NET framework includes a new security feature that blocks insecure cipher and hashing algorithms for connections and targets getting the more-secure behavior by default.
// create a separate class
// Include the `System.Net` and `System.IO` into your C# project and install NuGet `Newtonsoft.Json`
// package before executing the C# code.
using System.Net;
using System.IO;
using Newtonsoft.Json;
namespace cURLCall {
public class gta_allCustomersResponse {
public static void gta_AllCustomers() {
var httpWebRequest = (HttpWebRequest)WebRequest.Create(
"https://api.somewhere.com/desk/external_api/v1/customers.json");
httpWebRequest.ContentType = "application/json";
httpWebRequest.Accept = "*/*";
httpWebRequest.Method = "GET";
httpWebRequest.Headers.Add("Authorization", "Basic reallylongstring");
var httpResponse = (HttpWebResponse)httpWebRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
gta_allCustomersResponse answer =
JsonConvert.DeserializeObject<gta_allCustomersResponse>(streamReader.ReadToEnd());
// return answer;
}
}
}
}
Call the gta_AllCustomers()
method of the gta_allCustomersResponse
class into the main form to make a cURL call.
using static cURLCall.gta_allCustomersResponse;
gta_AllCustomers();
The HttpWebResponse
supports HTTP-specific users of the properties and methods of the WebResponse
class to make a cURL call in C#. Create an instance of the HttpWebResponse
class and close the response by calling Stream.Close
or HttpWebResponse.Close
methods and release the connection for reuse once the response is received successfully.
Use WebClient
to Make a CURL Call in C#
The WebClient
class provides data transformation or receiving methods over the local or internet resource identified by a URI. It uses the WebRequest
class to provide access to resources.
The WebClient
is a higher-level abstraction built on top of HttpWebRequest
to simplify the most common tasks to make a cURL call in C#.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using Newtonsoft.Json;
namespace cURLCall {
public static class StaticItems { public static string EndPoint = "http://localhost:8088/api/"; }
public class CityInfo {
public int CityID { get; set; }
public int CountryID { get; set; }
public int StateID { get; set; }
public string Name { get; set; }
public decimal Latitude { get; set; }
public decimal Longitude { get; set; }
}
public class receiveData {
public List<CityInfo> CityGet() {
try {
using (WebClient webClient = new WebClient()) {
webClient.BaseAddress = StaticItems.EndPoint;
var json = webClient.DownloadString("City/CityGetForDDL");
var list = JsonConvert.DeserializeObject<List<CityInfo>>(json);
return list.ToList();
}
} catch (WebException ex) {
throw ex;
}
}
}
public class ReturnMessageInfo {
public ReturnMessageInfo CitySave(CityInfo city) {
ReturnMessageInfo result = new ReturnMessageInfo();
try {
using (WebClient webClient = new WebClient()) {
webClient.BaseAddress = StaticItems.EndPoint;
var url = "City/CityAddUpdate";
webClient.Headers.Add(
"user-agent",
"Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.2; .NET CLR 1.0.3705;)");
webClient.Headers[HttpRequestHeader.ContentType] = "application/json";
string data = JsonConvert.SerializeObject(city);
var response = webClient.UploadString(url, data);
result = JsonConvert.DeserializeObject<ReturnMessageInfo>(response);
return result;
}
} catch (Exception ex) {
throw ex;
}
}
}
}
Classes like FileWebRequest
and FtpWebRequest
inherit from WebRequest
. In general, you can use WebRequest
to make a request and convert the return to either HttpWebRequest
, FileWebRequest
, or FtpWebRequest
, depending on your request.
It’s one of the ideal methods to make a cURL call by providing common operations to send and receive data from a resource identified by a URI.
Use HttpClient
to Make a CURL Call in C#
The HttpClient
class is engineered to be much better than any other class that offers methods to send HTTP requests and receive HTTP responses from a resource identified by a URI. Its instance is a collection of settings applied to all requests that act as a session to send HTTP requests.
In addition, every HttpClient
instance uses its connection pool for each cURL call.
In C#, HttpClient
is intended to be instantiated once and reused throughout the life of an application. As a higher-level API, HttpClient
wraps the lower-level functionality on each available platform (including platforms where it runs).
Furthermore, the behavior of this class is customizable to gain a significant performance benefit, especially for HTTP requests. Runtime configuration options in .NET can configure HttpClient
to customize its behaviour.
using System;
using System.Text;
using System.Threading.Tasks;
using System.Net.Http;
using Newtonsoft.Json;
class UserInfo {
public string UserName { get; set; }
public string Email { get; set; }
public override string ToString() {
return $"{UserName}: {Email}";
}
}
class cURLCall {
static async Task Main(string[] args) {
var user = new UserInfo();
user.UserName = "My Name";
user.Email = "myname4321@exp.com";
var json = JsonConvert.SerializeObject(person);
var data = new StringContent(json, Encoding.UTF8, "application/json");
var url = "https://httpbin.org/post";
var client = new HttpClient();
var response = await client.PostAsync(url, data);
string result = response.Content.ReadAsStringAsync().Result;
// processed user info thorugh HttpClient
Console.WriteLine(result);
}
}
The HttpClient
class has better support for handling different response types and also offers better support for asynchronous operations over the previously mentioned options.
Conclusion
This article has taught you three useful methods to make a cURL call in C#. You can now use the C# code to make cURL calls to test your endpoints and troubleshoot your C# applications.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub