How to Close Form in C#
-
Use the
Close()
Method to Close Form in C# -
Set the
DialogResult
Property in Windows Forms to Close Form in C# -
Use the
Application.Exit
Method to Exit the Application in C# -
Use the
Environment.Exit
Method to Exit the Application in C# -
Use the
Form.Hide()
Method to Hide Form in C# -
Use the
Form.Dispose()
Method to Dispose Form in C# - Conclusion
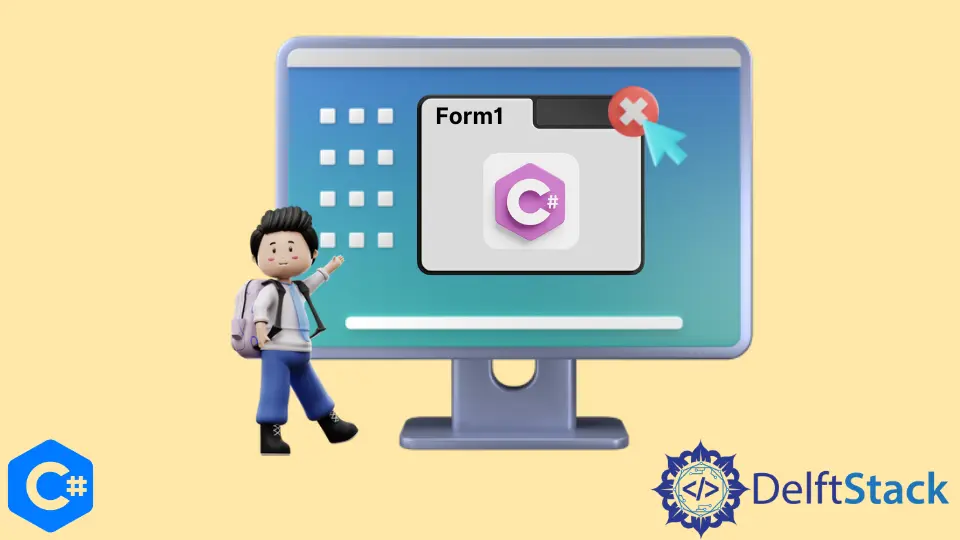
Closing a form is a fundamental operation in C# application development. Whether you’re building a Windows Forms Application or a Windows Presentation Foundation (WPF) Application, understanding how to close a form correctly is crucial for maintaining your application’s integrity and user experience.
This tutorial will explore different methods for closing a form in C# and provide step-by-step explanations and working code examples for each method.
Use the Close()
Method to Close Form in C#
The most straightforward way to close a form in C# is by using the Close()
method. This method is inherited from the System.Windows.Forms.Form
class and is available for Windows Forms and WPF applications.
Inside Form1
, we have a button (closeButton
) that, when clicked, calls this.Close()
to close the form.
using System;
using System.Windows.Forms;
namespace close_form {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void closeButton_Click(object sender, EventArgs e) {
this.Close();
}
}
}
In the above code, we closed the form in our Windows Forms application that only consists of one form with the Close()
method in C#.
This method only closes a single form in our application. It can also close a single form in an application that consists of multiple forms.
Set the DialogResult
Property in Windows Forms to Close Form in C#
In Windows Forms applications, you can use the DialogResult
property to close a form and indicate a specific result to the caller. This is often used when the form acts as a dialog box or a modal form.
using System;
using System.Windows.Forms;
namespace DialogResultDemo {
public partial class MainForm : Form {
public MainForm() {
InitializeComponent();
}
private void okButton_Click(object sender, EventArgs e) {
this.DialogResult = DialogResult.OK; // Set the DialogResult
this.Close(); // Close the form
}
private void cancelButton_Click(object sender, EventArgs e) {
this.DialogResult = DialogResult.Cancel; // Set the DialogResult
this.Close(); // Close the form
}
}
static class Program {
[STAThread]
static void Main() {
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
// Show the MainForm as a dialog
if (new MainForm().ShowDialog() == DialogResult.OK) {
MessageBox.Show("OK button clicked.");
} else {
MessageBox.Show("Cancel button clicked or form closed.");
}
}
}
}
In the above code, we create a Windows Forms application with a form named MainForm
containing two buttons (okButton
and cancelButton
). When the OK
or Cancel
button is clicked, we set the DialogResult
property accordingly and then call this.Close()
to close the form.
Use the Application.Exit
Method to Exit the Application in C#
The Application.Exit()
method is used to close the entire application in C#. This method informs all message loops to terminate execution and closes the application after all the message loops have terminated.
We can also use this method to close a form in a Windows Forms application if our application only consists of one form.
However, use this method with caution. It’s essential to make sure that it’s appropriate to exit the entire application at that point, as it can lead to unexpected behavior if other forms or processes need to be closed or cleaned up.
using System;
using System.Windows.Forms;
namespace close_form {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void exitButton_Click(object sender, EventArgs e) {
Application.Exit();
}
}
}
In the above code, when the Exit
button is clicked, we call Application.Exit()
to exit the entire application.
The drawback with this method is that the Application.Exit()
method exits the whole application. So, if the application contains more than one form, all the forms will be closed.
Use the Environment.Exit
Method to Exit the Application in C#
While it’s possible to close an application by calling Environment.Exit(0)
, it is generally discouraged.
This method forcefully terminates the application without allowing it to clean up resources or execute finalizers. It should only be used as a last resort when dealing with unhandled exceptions or critical issues.
using System;
using System.Windows.Forms;
namespace EnvironmentExitDemo {
public partial class MainForm : Form {
public MainForm() {
InitializeComponent();
}
private void exitButton_Click(object sender, EventArgs e) {
Environment.Exit(0); // Forcefully exit the entire application
}
}
}
In the above code, when the Exit
button is clicked, we call Environment.Exit(0)
to forcefully exit the entire application. This should be used sparingly, as it does not allow proper cleanup.
Application.Exit()
is specific to Windows Forms applications and provides a controlled, graceful exit, while Environment.Exit()
is a general-purpose method that forcibly terminates the application without any cleanup or event handling.
Use the Form.Hide()
Method to Hide Form in C#
Sometimes, you want to hide the form instead of closing it; you can use the Form.Hide()
method to do this. The form remains in memory and can be shown again using this.Show()
or this.Visible = true;
.
using System;
using System.Windows.Forms;
namespace FormHideDemo {
public partial class MainForm : Form {
public MainForm() {
InitializeComponent();
}
private void hideButton_Click(object sender, EventArgs e) {
this.Hide(); // Hide the form
}
private void showButton_Click(object sender, EventArgs e) {
this.Show(); // Show the hidden form
}
}
}
In the above code, we create a Windows Forms application with a form named MainForm
containing two buttons (hideButton
and showButton
).
When clicking the Hide
button, we call this.Hide()
to hide the form. When clicking the Show
button, we call this.Show()
to show the hidden form.
Use the Form.Dispose()
Method to Dispose Form in C#
You can explicitly dispose of a form and release its resources using the Form.Dispose()
method. It should be used when you explicitly want to release resources associated with the form, but it does not close its window.
using System;
using System.Windows.Forms;
namespace FormDisposeDemo {
public partial class MainForm : Form {
public MainForm() {
InitializeComponent();
}
private void disposeButton_Click(object sender, EventArgs e) {
this.Dispose(); // Dispose of the form
}
}
}
In the above code, when clicking the Dispose
button, we call this.Dispose()
to explicitly dispose of the form. This should be used when you want to release resources associated with the form.
Conclusion
Closing a form in C# involves several methods and considerations, depending on your application’s requirements. We have explored various methods, including using the Close()
method, setting the DialogResult
property, exiting the application, hiding the form, and disposing of the form.
Choosing the right method depends on your specific use case. Understanding when and how to use each method is crucial to ensure your application behaves as expected and provides a seamless user experience.
By mastering form closure techniques in C#, you can develop robust and user-friendly applications that meet the needs of your users while maintaining code integrity and resource management.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn