How to Check if Process Is Running in C#
-
Check if Process Is Running With the
Process.GetProcessByName()
Function inC#
-
Check if Process Is Running With the
Process.GetProcessById()
Function inC#
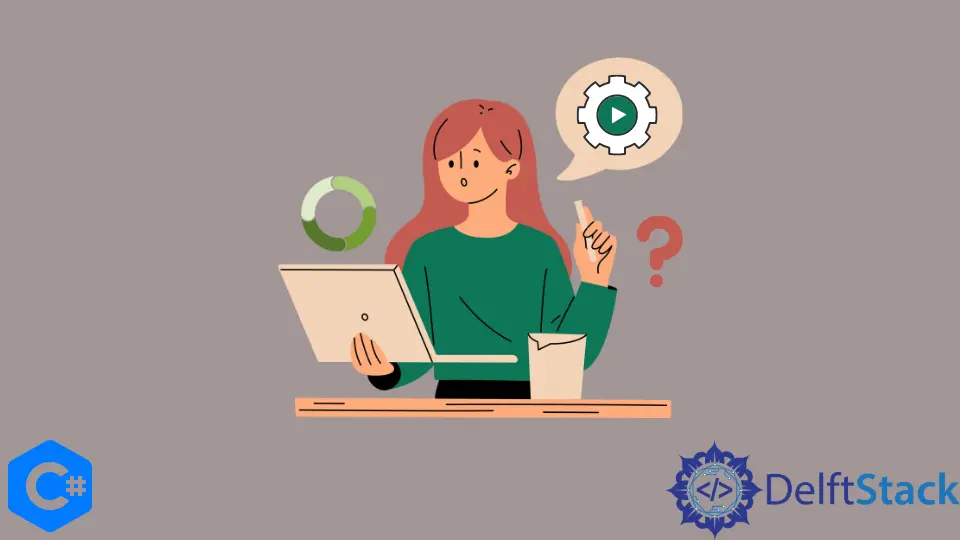
This tutorial will introduce the methods to check if a process is running in C#.
Check if Process Is Running With the Process.GetProcessByName()
Function in C#
The Process.GetProcessByName()
function gets all the running processes of the same name in C#. The Process.GetProcessByName()
function takes the name of the process as an input and returns an array of all the processes running by the same name. We can use this method to check if a process is running or not in our system. See the following code example.
using System;
using System.Diagnostics;
namespace check_if_process_is_running {
class Program {
static void Main(string[] args) {
Process[] processes = Process.GetProcessesByName("notepad");
if (processes.Length == 0) {
Console.WriteLine("Not running");
} else {
Console.WriteLine("Running");
}
}
}
}
Output:
Running
In the above code, we checked whether there were any processes with the name notepad
running on our system or not. We stored the result of the Process.GetProcessByName("notepad")
function in an array of processes. If the array’s length is zero, which means that the array is empty, then the process is not running. If there is even a single element inside the array, which means that the array is not empty, then the process with the specified name is running on our system.
Check if Process Is Running With the Process.GetProcessById()
Function in C#
The Process.GetProcessById()
function gets a process running in our system with the specified process id in C#. The Process.GetProcessById()
function takes the process id as a parameter and returns an instance of the Process
class with the specified id. This method can also be used to check if a process is running on our system or not. The following code example shows us how we can check if a process is running in our system or not with the Process.GetProcessById()
function in C#.
using System;
using System.Diagnostics;
namespace check_if_process_is_running {
class Program {
static bool isRunning(int id) {
try {
Process.GetProcessById(id);
} catch (InvalidOperationException) {
return false;
} catch (ArgumentException) {
return false;
}
return true;
}
static void Main(string[] args) {
bool running = isRunning(15);
if (running) {
Console.WriteLine("Running");
} else {
Console.WriteLine("Not Running");
}
}
}
}
Output:
Not Running
In the above code, we created a function isRunning()
that checks if a process is running or not. The isRunning()
function takes the process id as an argument and returns true
if the process is running and returns false
if it is not running.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn