Callback in C#
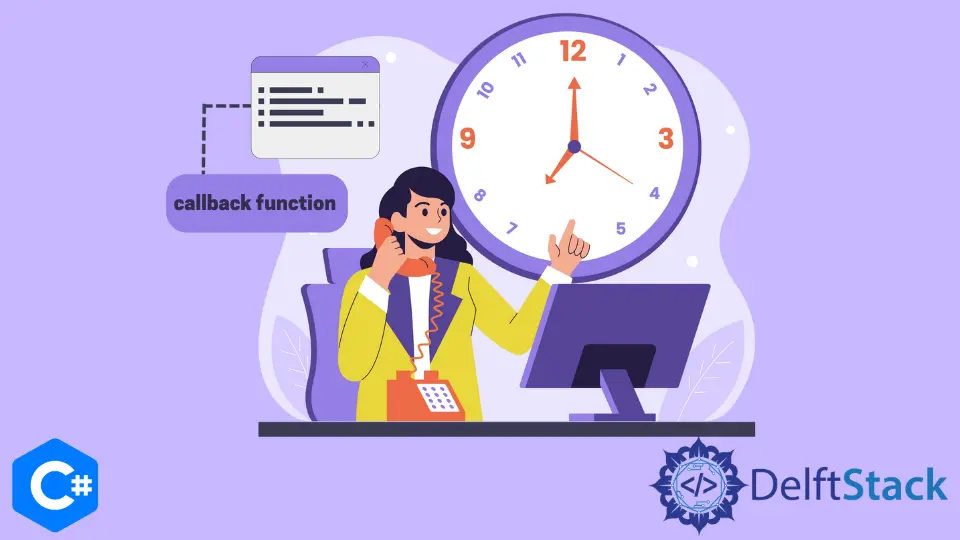
Today we will look into what a callback
is in C# and then go through some code to understand how it works.
What Is a callback
in C#
A callback
refers to some code passed as an argument to another code block that executes it later. Two of its types include; synchronous (immediate) and asynchronous (delayed) callbacks.
Synchronous callbacks work immediately, meaning they occur as soon as the function is invoked. Asynchronous (deferred) callbacks often happen once the function returns.
It, as you may have guessed, works for I/O operations that tend to invoke this function once some specific event occurs.
In C#, we use something known as delegates
, which are function pointers and can be used as callbacks
.
So you can define a method that takes a function as a parameter, and this method then tends to callback on the function with a message or something that it wants to pass back to it. Let’s take a look at an example below.
using System;
public class A {
static void Main(String[] args) {
B func = new B();
func.send_back(callbackMethod);
}
static void callbackMethod(int number) {
Console.WriteLine("The number is: " + number);
}
}
public class B {
public void send_back(Action<int> Callback) {
Callback(4);
}
}
So we have made two classes; A
and B
. A
has the Main
function defined and instantiates an object of class B
.
In B
, we have made a method that takes the function as a parameter and then invokes that function. So func
is called with the function, and B
then calls the function with the parameter 4
, which prints this number.
Output:
The number is: 4
The delegate
in the above example is the function: send_back()
. The event
is the calling of send_back()
and the callback
is the function that is invoked inside the send_back
method: callbackMethod()
.
We have already named that function callback
inside the B
method.
Use delegates
in C#
A delegate
tends to point to a method that matches its signatures and can call that function with or without the parameters defined in both. So, for example, we can say that we had the two functions, send_back()
and callbackMethod()
, and we want one to be the delegate
.
The callbackMethod()
is the delegate
. So we can write a code as follows.
using System;
public delegate void send_back(int num);
public class B {
public void send_back(Action<int> Callback) {
Callback(4);
}
}
public class Program {
static void Main(String[] args) {
B func = new B();
send_back handle = callbackMethod;
handle(4);
}
static void callbackMethod(int number) {
Console.WriteLine("The number is: " + number);
}
}
The delegate
is send_back
that is instantiated by a handle
that points to the method and then calls the method with the correct parameters. You may notice that handle(4)
is equal to callbackMethod(4)
.
This also seems very similar to Lambda
functions. The full code is shown below.
using System;
class classer {
public class A {
// DELEGATE METHOD
public delegate void send_back(int num);
static void Main(String[] args) {
B func = new B();
send_back handle = callbackMethod;
handle(4);
}
static void callbackMethod(int number) {
Console.WriteLine("The number is: " + number);
}
}
// THE CLASS B (THE FIRST CALLBACK METHOD)
public class B {
public void send_back(Action<int> Callback) {
Callback(4);
}
}
}
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub