How to Comment a Block of Text in C#
-
Single-Line and Multi-Line Comments for a Block of Text in
C#
- Comment a Block of Text in C# with Visual Studio’s Box Selection Feature
-
Comment a Block of Text with Space or Indent in
C#
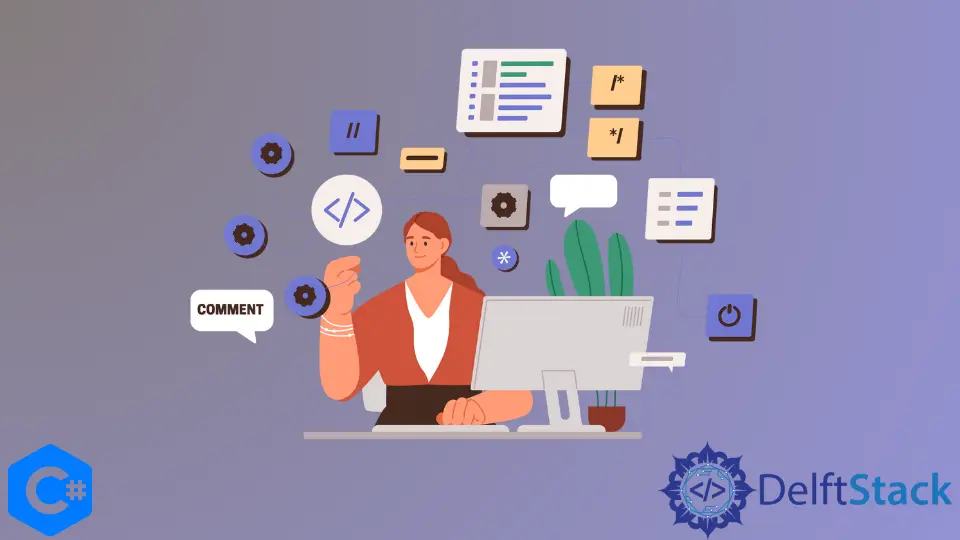
Comments as annotations are at a higher level of abstraction and are ignored by compilers when compiling your C# code. In this guide, you will learn different methods, syntax, and keyboard shortcuts to comment on a block of text or code in C#.
Single-Line and Multi-Line Comments for a Block of Text in C#
Single-line is the most common type of comment in C#. C# compiler ignores everything after double forward-slash or //
(which represents a single-line comment) until the end of the line.
Multi-line comments start with /*
and end with */
, and each line of text between them will act as a comment block.
// This is a single-line comment
// This is another line of comment
using System;
public class SingleLine {
public static void Main(string[] args) {
/*
This will be a comment line.
This feature is useful for commenting on multiple lines of code at once in C#
*/
Console.WriteLine("Hello World!");
}
}
Output:
Hello World!
Technically, you can write multi-line comments by using many single-line comments. If you have multiple lines of text to be commented in C#, use Ctrl+E+C to comment out these multiple lines.
As a shortcut, you can use Ctrl+K to comment and Ctrl+C to uncomment selected lines of text in C#. Alternatively, Ctrl+K can comment, and Ctrl+U can uncomment selected lines of C# code.
Comment a Block of Text in C# with Visual Studio’s Box Selection Feature
Visual Studio has a Box Selection
feature when performing multi-line editing in C#. Typing with box selection allows you to insert new text into each line of the comment or code.
You can select a rectangular text region with a mouse or keyboard and press Alt to activate the Box Selection
feature.
Place a mouse pointer on the text and hold the Alt key. Select a rectangular region with the left mouse key or from the keyboard’s arrow keys while holding the Alt key.
Release the left mouse button and the Alt key to insert text in each line of the selected comment block at once.
Before:
int First;
int Second;
int Third;
Use Ctrl+E+C to comment multiple lines of code at once and type Line of Code
after selecting int
from each line using the Box Selection
feature.
After:
// Line of Code First
// Line of Code Second
// Line of Code Third
The Box Selection
feature enables developers to add text to multiple lines of a comment block at once. Box selection
feature helps make a vertical selection zero characters wide to create a multi-line insert point for the new or copied text.
Comment a Block of Text with Space or Indent in C#
When you comment a block of text using Ctrl+E+C after selecting some lines of code using Ctrl+K, C, you get:
// First line
// Second line
// Third line
To insert a space or indent between //
and the text of a comment, move the cursor to the first line after //
. Then press Alt+Shift and use the up and down arrow keys on the keyboard to select a block of text.
Once the selection is complete, press the space bar to enter a space (indent) in a comment block, and you will get:
// First line
// Second line
// Third line
These keyboard shortcuts are introduced as a part of Visual Studio 2010 and are valid for later versions of VS, including Visual Studio 2022 IDE.
Alternatively, multi-line comment /*
before the first line of a block of text and */
after the last line of a block of text eliminates the need for any space or indent.
/*
First line
Second line
Third line
*/
In this tutorial, you have learned every method to comment on a block of text or code in C#. Each method offers some unique benefits for different scenarios.
Use your judgment and experience to decide which method will serve you well.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub