How to Use a Bitmask in C#
- Use a Bitmask in C#
-
Bitwise
AND
(&
) Operator -
Bitwise
OR
(|
) Operator -
Bitwise
NOT
(~
) Operator -
Bitwise
XOR
(^
) Operator -
Bit Shifting (
<<
and>>
) Operators - Conclusion
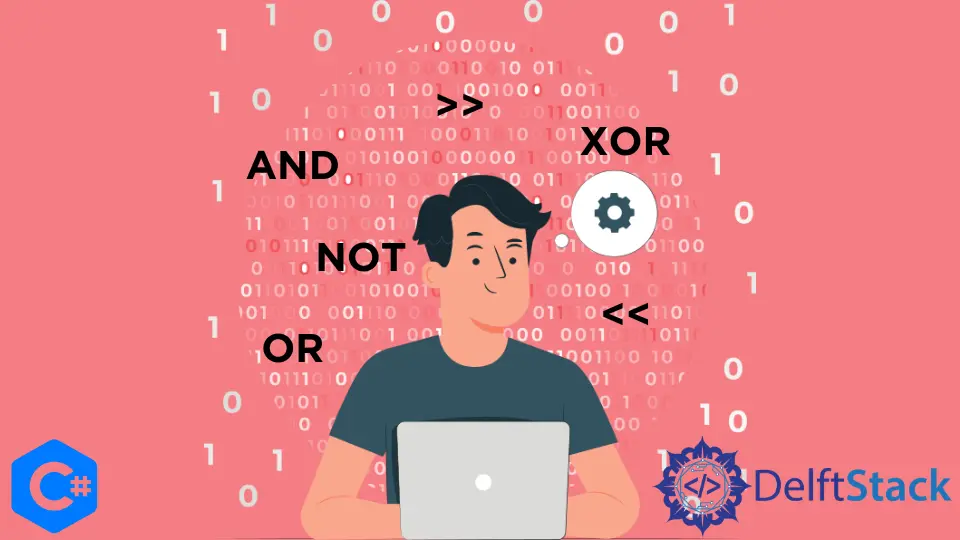
Bitmasks are a fundamental concept in computer science that involves using individual bits within a binary number to represent states, settings, or flags. In C#, bitmasks are commonly employed for bitwise operations, such as setting, clearing, toggling, or checking individual bits in an integer or other data types.
This post will look at how bitmask may be used in C#. The most important thing is that you understand the general idea behind what you are doing, even if there are a lot of various methods to do this assignment.
You must be aware that you will be working with the bits in the memory to utilize the bitmask.
Use a Bitmask in C#
C# has a variety of operators, most of which are referred to as bitwise operators. These operators carry out operations on the bit level in memory.
The bitwise operators with their symbols are as follows.
SIGN | OPERATOR |
---|---|
& |
AND |
` | ` |
~ |
NOT |
^ |
XOR |
<< |
left shift |
>> |
right shift AND |
Bitwise AND
(&
) Operator
The bitwise AND
operator is used to manipulate bits by performing an AND
operation between two values. This method allows checking the state of a particular bit within an integer.
The working of the AND
operator is as follows.
X Y Res
0 0 0
0 1 0
1 0 0
1 1 1
Res
is the output, and X
and Y
are the input possibilities.
The most common operator used for bitmask is the AND
operator; it is used to see which bits are one and which are zero because its output is one when both inputs are 1
.
Below is the code evaluating the last 4 bits masked.
using System;
namespace HelloWorld {
class Program {
static void Main(string[] args) {
char x = '2';
int y = 0b00001111;
int z = x & y;
Console.WriteLine(z);
}
}
}
Output:
2
For it to have 1 byte of space in the memory, we constructed the char
type variable x
and the int
type variable y
. The binary version of the corresponding decimal value, in which the final four bits are 1
, is written as 0b00001111
.
The line that reads int z= x&y;
computes the bitwise and of the bits and saves the result in the variable named z
. Since the z
variable is of the int
data type, we can see the result expressed in decimal form.
The operation is as follows:
x = 01111101
y = 00001111
z = x & y = 00001101
Because this is visible, we know that the x
variable was stripped of all except its most recent four bits. To do it, we employed the AND
operator.
Similarly, we may combine the bits using the OR
operator. You should now be able to see that if you have a solid knowledge of the bits, it is now straightforward to play with them and generate your ciphers.
Bitwise OR
(|
) Operator
The bitwise OR
operator is used to set or turn on specific bits within an integer by performing an OR
operation.
The working of the OR
operator is as follows:
X Y Res
0 0 0
0 1 1
1 0 1
1 1 1
Res
is the output, and X
and Y
are the input possibilities.
Example:
using System;
namespace HelloWorld {
class Program {
static void Main(string[] args) {
// Bitmask creation and setting a bit using bitwise OR operator
int bitmask = 0b101010; // Example bitmask
int flag = 0b001000; // Bit to set
bitmask |= flag; // Set the bit
Console.WriteLine(
Convert.ToString(bitmask, 2)); // Output: 101010 | 001000 = 101010 (Bit set)
}
}
}
Output:
101010
The code creates a bitmask and sets a specific bit using bitwise operations. It initializes a bitmask
and a flag
representing a bit to set.
The bitwise OR
operation (|=
) is then used to modify the bitmask
, setting the bit represented by the flag
. The resulting bitmask
is displayed in its binary representation, confirming the successful bit setting operation.
Bitwise NOT
(~
) Operator
The bitwise NOT
operator is a unary operator used to invert bits in an integer, effectively performing a one’s complement operation.
The working of the NOT
operator is as follows:
X Y
1 0
0 1
Y
is the output, and X
is the possibilities of input.
Example:
using System;
namespace HelloWorld {
class Program {
static void Main(string[] args) {
int bitmask = 0b101010; // Example bitmask
// Invert the bits and keep only the last 8 bits
int invertedMask = ~bitmask & 0xFF;
// Convert the result to a binary string and pad it to 8 bits
string binaryString = Convert.ToString(invertedMask, 2).PadLeft(8, '0');
Console.WriteLine(binaryString); // Output: 11010101 (8-bit representation)
}
}
}
Output:
11010101
This code focuses on bitwise operations to invert bits in a bitmask. It initializes a bitmask
with the value 0b101010
(binary representation).
The bitwise NOT
operator (~
) is applied to the bitmask
, which inverts all its bits, generating a new value invertedMask
. The resulting invertedMask
is displayed in binary format using Convert.ToString()
to demonstrate the inversion of bits, resulting in 11010101
.
Bitwise XOR
(^
) Operator
The bitwise XOR
operator performs an exclusive OR
operation between corresponding bits, toggling or flipping bits.
The working of the XOR
operator is as follows:
X Y Res
0 0 0
0 1 1
1 0 1
1 1 0
Res
is the output, and X
and Y
are the input possibilities.
Example:
using System;
namespace HelloWorld {
class Program {
static void Main(string[] args) {
// Bitmask creation and toggling a bit using the bitwise XOR operator
int bitmask = 0b101010; // Example bitmask
int flag = 0b001000; // Bit to toggle
bitmask ^= flag; // Toggle the bit
Console.WriteLine(
Convert.ToString(bitmask, 2)); // Output: 101010 ^ 001000 = 100010 (Bit toggled)
}
}
}
Output:
100010
This code demonstrates the use of the bitwise XOR
operator (^
) to toggle a specific bit in a bitmask. Initially, it sets up a bitmask
with the value 0b101010
and a flag
with the value 0b001000
, representing the bit to be toggled.
By performing a bitwise XOR
operation between the bitmask
and the flag
(bitmask ^= flag
), the designated bit is toggled: if the bit in the bitmask
and the flag
is different, it becomes 1
; if they are the same, it becomes 0
.
The resulting value of bitmask
after the toggle operation is 0b100010
, as demonstrated by converting it to a binary string using Convert.ToString()
. This result reflects the toggled bit (101010 ^ 001000 = 100010
).
Bit Shifting (<<
and >>
) Operators
Bit shifting operators (left shift <<
and right shift >>
) move bits left or right within an integer, effectively multiplying or dividing by powers of 2
.
The working of the left-shift
operator is as follows:
x = 010110011
If we give it two left shifts, the bits will move left 2-bit spaces.
x << 2
Now the x
will be:
x = 011001100
And on the right side, two new 0
bits will be added.
The working of the right shift operator is as follows:
x = 010110011
If we give it two right shifts, the bits will move left 2-bit spaces.
x >> 2
Now the x
will be:
x = 000101100
And on the left side, two new 0
bits will be added. Now, as we know the working of the bitwise operator, we can apply bitmask to our inputs.
Example:
using System;
namespace HelloWorld {
class Program {
static void Main(string[] args) {
// Bitmask creation and shifting bits using bit shift operators
int bitmask = 0b101010; // Example bitmask
int shiftedLeft = bitmask << 2; // Left shift by 2 bits
int shiftedRight = bitmask >> 1; // Right shift by 1 bit
Console.WriteLine(
Convert.ToString(shiftedLeft, 2)); // Output: 101010 << 2 = 10101000 (Bits shifted left)
Console.WriteLine(
Convert.ToString(shiftedRight, 2)); // Output: 101010 >> 1 = 10101 (Bits shifted right)
}
}
}
Output:
10101000
10101
The code demonstrates the utilization of bitwise shift operators (<<
and >>
) to manipulate a bitmask. It initializes a bitmask with the value 0b101010
, showcasing various bit-shifting operations:
- Left Shifting:
bitmask << 2
shifts all bits in thebitmask
2 positions to the left, displaying10101000
in binary. - Right Shifting:
bitmask >> 1
shifts all bits in thebitmask
1 position to the right, resulting in10101
in binary.
These operations exhibit how bitwise shift operators alter the positions of bits within the bitmask, facilitating the movement of bits either left or right based on the specified shift count. The code showcases the binary representations of the shifted integers using Convert.ToString()
and outputs the results using Console.WriteLine()
.
Conclusion
Bitmasks are versatile tools in C# for manipulating individual bits within integers, enabling efficient storage and retrieval of flags or settings. Using various bitwise operators and bit shifting, programmers can effectively set, clear, toggle, or check specific bits within integers to represent various states or options in their applications.
These methods provide powerful tools for bitwise operations, enhancing the capabilities of C# programs when dealing with bit-level manipulation.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn