async and await in C#
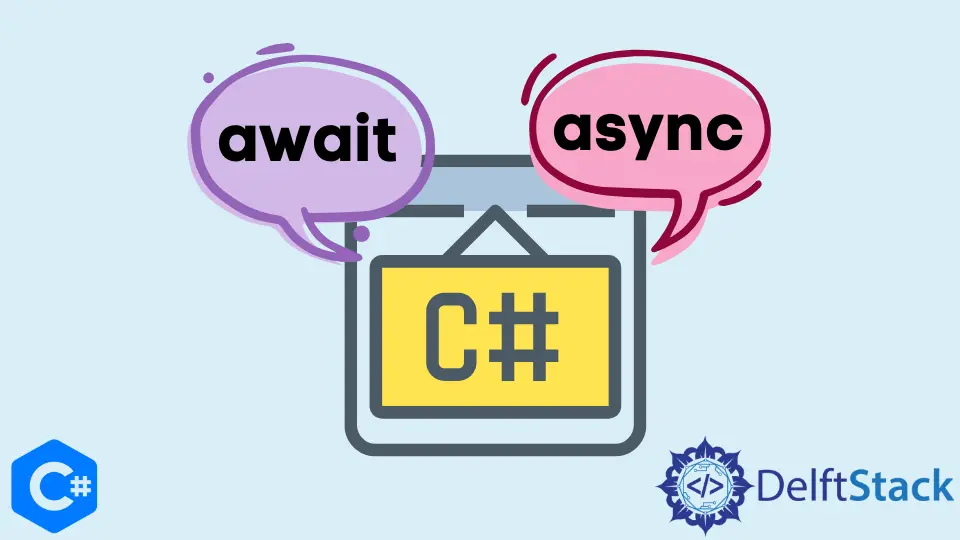
This tutorial will discuss Asynchronous programming in C#.
Asynchronous Programming in C#
If any process is blocked in a synchronous application, the whole application gets blocked and stops responding until that particular process gets completed. We can use asynchronous programming in this scenario. With asynchronous programming, our application can continue working in the background on some independent tasks until this particular process executes. In asynchronous programming, our whole application does not simply depend upon a single time-consuming process. Asynchronous programming is used with I/O bound or CPU bound tasks. The main use of asynchronous programming is to create a responsive User-Interface that does not get stuck while waiting for an I/O bound or CPU bound process to complete its execution. The await
and async
keywords are used for asynchronous programming in C#. The await
keyword gives control back to the calling function. The await
keyword is the main keyword that enables asynchronous programming in C#. The async
keyword enables the await
keyword. A function utilizing the await
keyword must have the async
keyword and return a Task
object in C#. The await
keyword cannot be used without the async
keyword in C#. The following code example demonstrates synchronous programming in C#.
using System;
using System.Threading.Tasks;
namespace async_and_await {
class Program {
public static void process1() {
for (int i = 0; i < 5; i++) {
Console.WriteLine("Process 1");
Task.Delay(100).Wait();
}
}
public static void process2() {
for (int i = 0; i < 5; i++) {
Console.WriteLine("Process 2");
Task.Delay(100).Wait();
}
}
static void Main(string[] args) {
process1();
process2();
}
}
}
Output:
Process 1
Process 1
Process 1
Process 1
Process 1
Process 2
Process 2
Process 2
Process 2
Process 2
In the above code, the functions process1()
and process2()
are independent processes but the process2()
function has to wait for the completion of the process1()
function. This simple synchronous programming code can be converted to asynchronous programming with async
and await
keywords in C#.
using System;
using System.Threading.Tasks;
namespace async_and_await {
class Program {
public static async Task process1() {
await Task.Run(() => {
for (int i = 0; i < 5; i++) {
Console.WriteLine("Process 1");
Task.Delay(100).Wait();
}
});
}
public static void process2() {
for (int i = 0; i < 5; i++) {
Console.WriteLine("Process 2");
Task.Delay(100).Wait();
}
}
static void Main(string[] args) {
process1();
process2();
}
}
}
Output:
Process 2
Process 1
Process 1
Process 2
Process 2
Process 1
Process 1
Process 2
Process 2
Process 1
In the above code, we used the async
and await
keywords to convert the previous example to asynchronous programming. The output clearly shows that the process2()
function does not wait to complete the process1()
function. In the definition of the process1()
function, we used the async
keyword to suggest that this is an Asynchronously executing function. The await
keyword in the process1()
function gives the control back to the main function, which then calls the process2()
function. The control keeps shifting between the process1()
and process2()
functions until the completion of process1()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn