How to Add Days to Date in C#
-
Use the
DateTime.AddDays()
Function to Add Days to Date inC#
-
Add Days to Date Using User Input in
C#
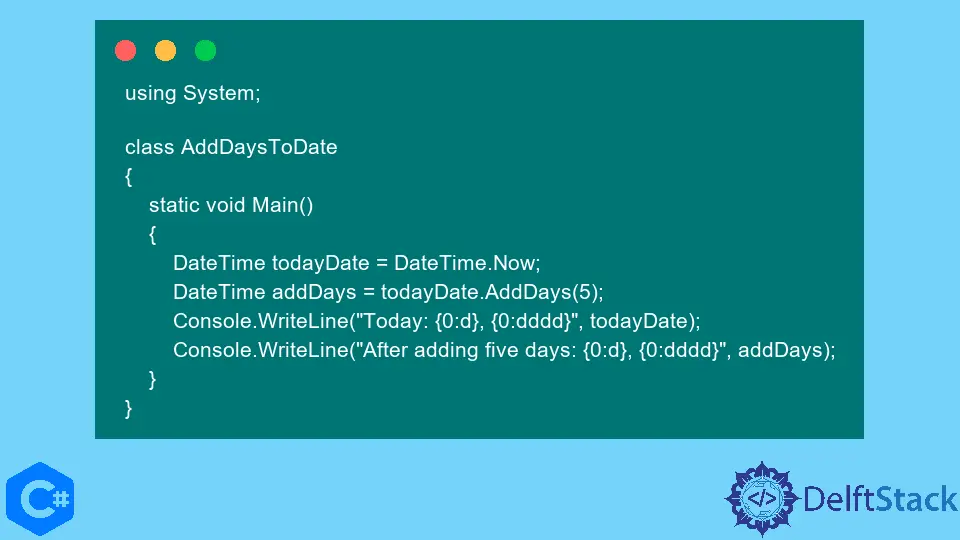
This article demonstrates how to add days to date using C# programming language.
Use the DateTime.AddDays()
Function to Add Days to Date in C#
Several built-in methods and attributes are available in the C# programming language that may be used to handle DateTime
objects. DateTime.AddDays()
is a method that, when called, returns a new DateTime
that modifies the value of this DateTime
object instance by adding the provided number of days.
You need to pass an argument while using this function. The name of this option is value
, and its data type is double.
This value
parameter is an integer that may be either a complete day or a fraction of a day. A positive or negative number may be used for this value
parameter.
The DateTime.AddDays()
function gives a System.DateTime
as its return value. The returned DateTime
object is the sum of the DateTime instance supplied together with the number of days that have passed as specified by the argument.
This method does not change the DateTime
object being supplied but returns the result as a brand new DateTime
object.
Let’s look at an example that takes the date of today as an input, adds 5 days to it, and then displays the resultant date along with the day.
Source Code:
using System;
class AddDaysToDate {
static void Main() {
DateTime todayDate = DateTime.Now;
DateTime addDays = todayDate.AddDays(5);
Console.WriteLine("Today: {0:d}, {0:dddd}", todayDate);
Console.WriteLine("After adding five days: {0:d}, {0:dddd}", addDays);
}
}
Output:
Today: 11-Oct-2022, Tuesday
After adding five days: 16-Oct-2022, Sunday
Add Days to Date Using User Input in C#
In this method, we will ask the user to provide the date in a particular format, and then we will ask the user to add days to the date. After that, we will display the day and the date with the appended user’s chosen number of additional days.
Source Code:
using System;
class Program {
static void Main(string[] args) {
Console.WriteLine("Enter the year in the format dd-mm-yyyy");
DateTime dateTime = Convert.ToDateTime(Console.ReadLine());
Console.WriteLine("Enter the days to add:");
int addDays = Convert.ToInt32(Console.ReadLine());
DateTime newDate = dateTime.AddDays(addDays);
Console.WriteLine("The new day and date after adding days: " + newDate.ToLongDateString());
}
}
Output:
Enter the year in the format dd-mm-yyyy
13-04-2000
Enter the days to add:
8
The new day and date after adding days: Friday, 21 April 2000
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn