How to Print System Time in C++
-
Use
std::chrono::system_clock
andstd::ctime
to Print System Time in C++ -
Use
time
,localtime
andstrftime
to Print System Time in C++
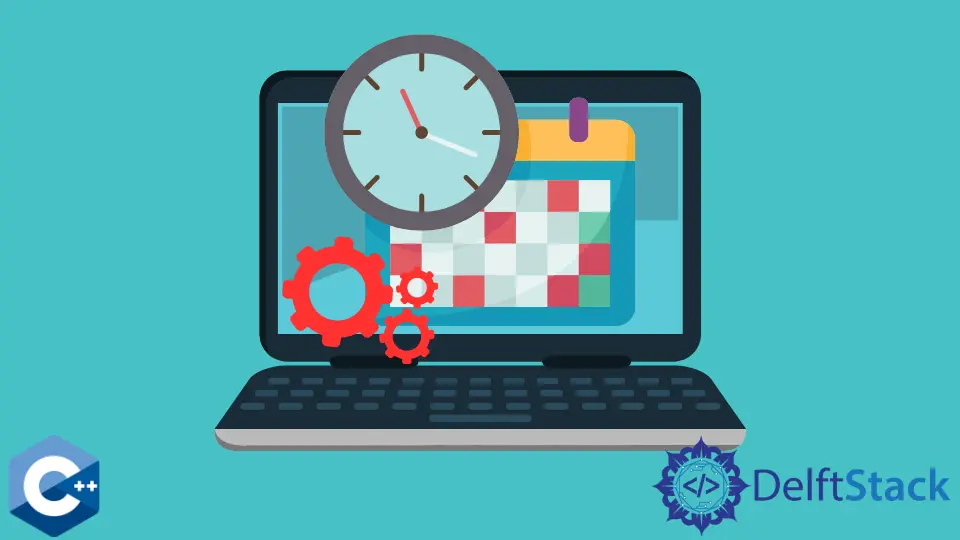
This article will explain several methods of how to print system time in C++.
Use std::chrono::system_clock
and std::ctime
to Print System Time in C++
std::chrono::system_clock
represents the system-wide wall clock and it provides two functions to convert to/from std::time_t
type. The latter object we can process using the ctime
function and return a null-terminated string of the form - Wed Jun 30 21:49:08 1993\n
. In this case, we construct a separate function to encapsulate both calls and return the string
value to the caller. Notice that we also remove the new line character to return value in a more flexible form. Also, system_clock::now
is used to retrieve the current timepoint.
#include <sys/time.h>
#include <chrono>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
std::string timeToString(std::chrono::system_clock::time_point &t) {
std::time_t time = std::chrono::system_clock::to_time_t(t);
std::string time_str = std::ctime(&time);
time_str.resize(time_str.size() - 1);
return time_str;
}
int main() {
auto time_p = std::chrono::system_clock::now();
cout << "Current time: " << timeToString(time_p) << endl;
return EXIT_SUCCESS;
}
Output:
Current time: Fri Apr 1 01:34:20 2021
One can also utilize the same method to display Epoch value in a similar form, as demonstrated in the following example. Note that, Epoch is usually January 1 of 1970 for POSIX/UNIX systems, but it’s not required to be the same value for different clocks provided in the chrono
library.
#include <sys/time.h>
#include <chrono>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
std::string timeToString(std::chrono::system_clock::time_point &t) {
std::time_t time = std::chrono::system_clock::to_time_t(t);
std::string time_str = std::ctime(&time);
time_str.resize(time_str.size() - 1);
return time_str;
}
int main() {
std::chrono::system_clock::time_point time_p2;
cout << "Epoch: " << timeToString(time_p2) << endl;
return EXIT_SUCCESS;
}
Output:
Epoch: Thu Jan 1 00:00:00 1970
Use time
, localtime
and strftime
to Print System Time in C++
Alternatively, we can use POSIX specific function - time
and directly retrieve the time_t
structure. time_t
is essentially an integer that stores the number of seconds since the Epoch. Similarly to the previous method, we can use ctime
to convert to the string of predefined form or call the localtime
function. The localtime
function converts the time_t
object to the tm
structure, which is the broken-down time format that can be utilized to format the output string as we wish with special specifiers. Formatting is done by the strftime
function that takes four parameters, the last of which is the pointer to struct tm
. The first argument specifies the memory address where the character string will be stored, and the next two arguments are the maximum size of the string and the format specifier. The detailed overview of format specification can be seen on this page.
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
using std::string;
int main() {
char tt[100];
time_t now = time(nullptr);
auto tm_info = localtime(&now);
strftime(tt, 100, "%Y-%m-%d %H:%M:%S", tm_info);
puts(tt);
return EXIT_SUCCESS;
}
Output:
2021-04-02 05:42:46
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook