How to Measure Execution Time of a Function in C++ STL
-
Use
std::chrono::high_resolution_clock::now
andstd::chrono::duration_cast<std::chrono::seconds>
to Measure Execution Time of a Function -
Use
std::chrono::high_resolution_clock::now
andstd::chrono::duration<double, std::milli>
to Measure Execution Time of a Function
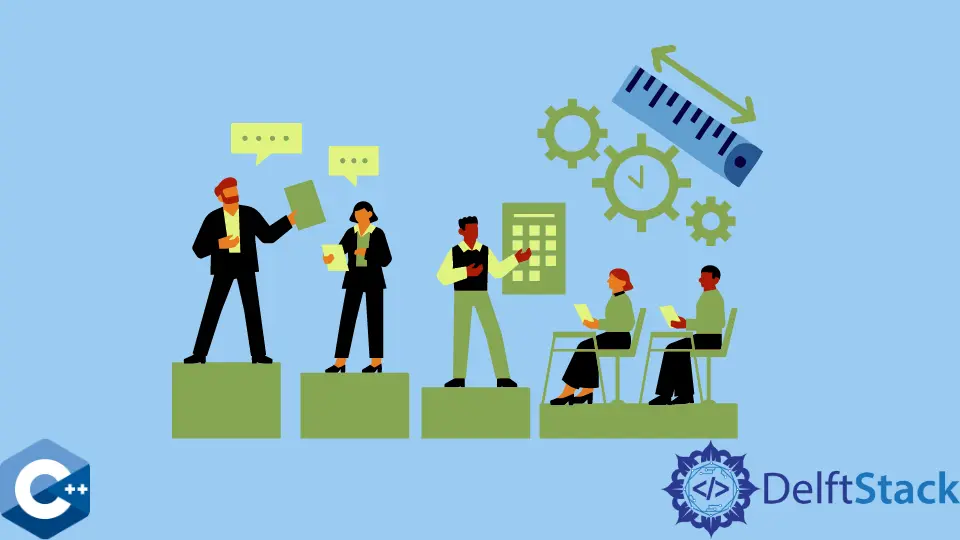
This article will demonstrate multiple methods about how to measure the execution time of a function in C++.
Use std::chrono::high_resolution_clock::now
and std::chrono::duration_cast<std::chrono::seconds>
to Measure Execution Time of a Function
std::chrono
namespace consolidates all date and time utilities provided by the C++ STL library. The latter offers multiple clock implementations, one of which is std::chrono::high_resolution_clock
that corresponds to the clock with the smallest tick period. Note though, this clock is hardware platform-dependent, and even multiple standard library implementations differ, so it’s best to read the compiler documentation and make sure this one is suited for the problem requirements. The idea for measuring the execution time of a function is to retrieve the current time from the given clock twice: before the function call and after, and calculate the difference between the values. The current time is retrieved using the now
built-in method. Once the difference is calculated, it should be interpreted in a certain unit of time, which is done using the std::chrono::duration_cast
utility. In the following example, we cast the result in std::chrono::seconds
units and output the value with the count
built-in function. Notice that the funcSleep
in the code example suspends the program’s execution for 3 seconds and then returns control to the main
function.
#include <chrono>
#include <iostream>
#include <thread>
using std::cout;
using std::endl;
void funcSleep() { std::this_thread::sleep_for(std::chrono::seconds(3)); }
int main() {
auto start = std::chrono::high_resolution_clock::now();
funcSleep();
auto end = std::chrono::high_resolution_clock::now();
auto int_s = std::chrono::duration_cast<std::chrono::seconds>(end - start);
std::cout << "funcSleep() elapsed time is " << int_s.count() << " seconds )"
<< std::endl;
return EXIT_SUCCESS;
}
Output:
funcSleep() elapsed time is 3 seconds
Use std::chrono::high_resolution_clock::now
and std::chrono::duration<double, std::milli>
to Measure Execution Time of a Function
In contrast with the previous code, where the unit of time was saved in an integer value, the next example stores the interval value as a floating-point number in the std::chrono::duration<double, std::milli>
type object. std::chrono::duration
is a general class template for representing the time interval. Finally, the interval value is retrieved using the count
function, at which point it can be printed to the cout
stream.
#include <chrono>
#include <iostream>
#include <thread>
using std::cout;
using std::endl;
void funcSleep() { std::this_thread::sleep_for(std::chrono::seconds(3)); }
int main() {
auto start = std::chrono::high_resolution_clock::now();
funcSleep();
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double, std::milli> float_ms = end - start;
std::cout << "funcSleep() elapsed time is " << float_ms.count()
<< " milliseconds" << std::endl;
return EXIT_SUCCESS;
}
Output:
funcSleep() elapsed time is 3000.27 milliseconds
The past two examples measured a function that mostly takes constant time, but a similar approach can be used to calculate the given code block’s execution time. As an example, the following snippet demonstrates the random integer generation function that takes more variable intervals to execute. Notice that, this time, we used both methods to display time with milliseconds precision and seconds.
#include <chrono>
#include <iostream>
#include <thread>
using std::cout;
using std::endl;
constexpr int WIDTH = 1000000;
void generateNumbers(int arr[]) {
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
}
int main() {
int *arr = new int[WIDTH];
auto start = std::chrono::high_resolution_clock::now();
generateNumbers(arr);
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double, std::milli> float_ms = end - start;
auto int_ms =
std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
std::chrono::duration<long, std::micro> int_usec = int_ms;
std::cout << "generateNumbers() elapsed time is " << float_ms.count()
<< " ms "
<< "( " << int_ms.count() << " milliseconds )" << std::endl;
delete[] arr;
return EXIT_SUCCESS;
}
Output:
generateNumbers() elapsed time is 30.7443 ms ( 30 milliseconds )
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook