How to Wait for User Input in C++
-
Wait for User Input in C++ Using the
cin.get()
Method -
Wait for User Input in C++ Using the
getchar
Function -
Wait for User Input in C++ Using the
getc
Function -
Wait for User Input in C++ Using the
system
Function - Conclusion
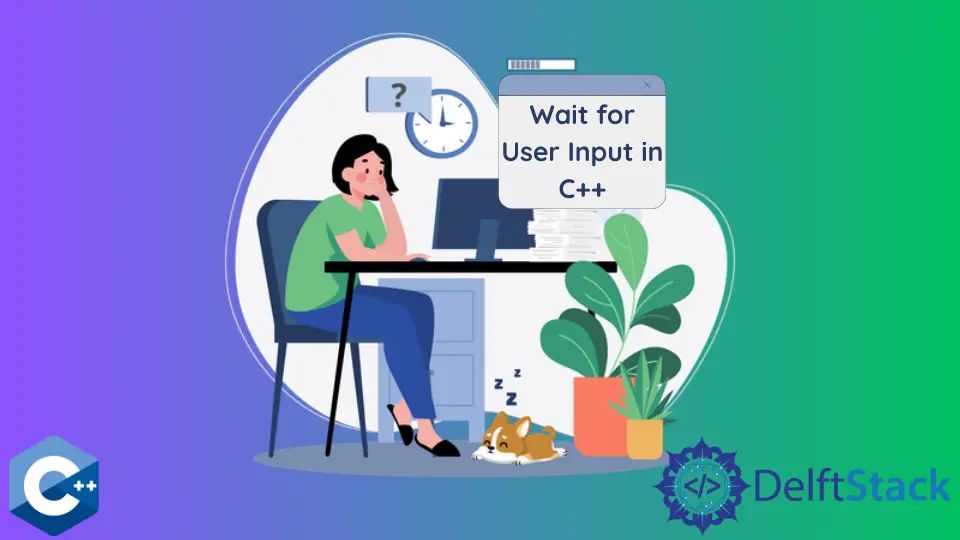
User input can come from various sources, such as the keyboard, mouse, or other input devices, depending on the nature of the application. Whether you’re expecting a single keypress, a sequence of characters, or a numeric input, the ability to wait for and capture user input is important for creating interactive and dynamic programs.
In this article, we will explore various methods to wait for user input in C++. Each method has its nuances, advantages, and use cases, empowering you with the flexibility to choose the most suitable approach for your specific programming scenario.
Wait for User Input in C++ Using the cin.get()
Method
In C++, one method to wait for user input is by using the cin.get()
method.
The cin.get()
method is a member function of the cin
stream in C++. It is used to read a single character from the standard input (keyboard).
Unlike the >>
operator, which can leave whitespace characters in the input buffer, cin.get()
reads the next character, including whitespace like spaces and newline characters.
When cin.get()
is called without any arguments, it reads a single character from the standard input and returns its ASCII value. It effectively waits for the user to press a key, and the program proceeds once a key is pressed, including the Enter key.
Let’s dive into a practical example demonstrating the use of cin.get()
for waiting for user input:
#include <iostream>
int main() {
char userInput;
std::cout << "Press any key to continue: ";
userInput = std::cin.get();
std::cout << "You pressed: " << userInput << std::endl;
return 0;
}
In this C++ code snippet, we begin by including the necessary header <iostream>
, which is essential for input/output operations in C++. Inside the main()
function, we declare a character variable named userInput
to store the character entered by the user.
Next, we prompt the user with a message using std::cout
. The message instructs the user to Press any key to continue
. This acts as a visual cue to inform the user about the expected interaction.
The key aspect of this code lies in the line userInput = std::cin.get();
. Here, we use the std::cin.get()
method to wait for the user to input a character.
Unlike other input methods, std::cin.get()
captures the first character entered by the user, including whitespace characters like spaces and newline characters. This effectively puts the program in a waiting state until the user presses a key, and the entered character is stored in the userInput
variable.
Finally, the program prints the result using std::cout
. The message You pressed:
is concatenated with the actual character the user pressed (userInput
), and a newline character (std::endl
) is added for better formatting.
Code Output:
In this example, the user pressed the A
key, and the program responded accordingly. The cin.get()
method effectively waits for the user input, allowing the program to pause until a key is pressed.
Wait for User Input in C++ Using the getchar
Function
Another method to wait for user input is by using the getchar
function. This function belongs to the C standard library and reads a single character from the standard input stream (stdin
).
The getchar
function is a simple yet effective way to capture user input. It reads a single character from the standard input and returns its ASCII value.
Similar to other input methods, getchar
waits for the user to press a key, and the program proceeds once a key is pressed, including the Enter key. If an error occurs or the end of the input stream is reached, getchar
returns EOF
(End of File).
Let’s explore a practical example demonstrating the use of the getchar
function for waiting for user input:
#include <cstdio>
int main() {
char userInput;
// Prompting the user
printf("Press any key to continue: ");
// Waiting for user input using getchar()
userInput = getchar();
// Displaying the result
printf("You pressed: %c\n", userInput);
return 0;
}
In the example above, we start by including the <cstdio>
header, which is necessary for input/output operations in C++.
Within the main()
function, a character variable named userInput
is declared. This variable will store the character entered by the user.
Following that, we use the printf
function to prompt the user with a message. The message Press any key to continue:
is displayed on the console, serving as an instruction for the user. This acts as a visual cue, indicating the expected interaction.
The key functionality of the code lies in the line userInput = getchar();
.
Here, the getchar()
function is employed to wait for the user to input a character. The entered character is then assigned to the userInput
variable. getchar
effectively pauses the program until a key is pressed, including the Enter key.
Finally, the program prints the result using printf
. The message You pressed:
is concatenated with the actual character the user pressed (userInput
).
The %c
format specifier is used in the format string to correctly display the character.
Code Output:
In this example, the user pressed the X
key, and the program responded by displaying the pressed key. The getchar
function effectively waits for the user to input a character, allowing the program to pause until a key is pressed.
Wait for User Input in C++ Using the getc
Function
In C++, another method for waiting for user input is the getc
function. This function, similar to getchar
, is part of the C standard library and reads a single character from the input stream.
The getc
function is a versatile input function that reads a character from a given input stream. It takes a FILE *stream
as an argument, representing the input stream from which it reads.
In our case, we pass stdin
, which is the standard input stream typically associated with keyboard input. Like other input functions, getc
waits for the user to press a key, and the program proceeds once a key is pressed, including the Enter key.
If an error occurs or the end of the input stream is reached, getc
returns EOF
(End of File).
Let’s explore a practical example demonstrating the use of the getc
function for waiting for user input:
#include <cstdio>
int main() {
char userInput;
// Prompting the user
printf("Press any key to continue: ");
// Waiting for user input using getc()
userInput = getc(stdin);
// Displaying the result
printf("You pressed: %c\n", userInput);
return 0;
}
This C++ program begins by including the <cstdio>
header, which is essential for input/output operations in C++. Inside the main()
function, a character variable named userInput
is declared to store the character entered by the user.
Similar to the previous code example, we use the printf
function to prompt the user with a message.
The message Press any key to continue:
is displayed on the console, acting as an instruction for the user. This serves as a visual cue, indicating the expected interaction.
The core functionality of the code resides in the line userInput = getc(stdin);
.
Here, the getc(stdin)
function is employed to wait for the user to input a character. The entered character is then assigned to the userInput
variable. getc
effectively pauses the program until a key is pressed, including the Enter key.
Finally, the program prints the result using printf
. The message You pressed:
is concatenated with the actual character the user pressed (userInput
). The %c
format specifier is used in the format string to correctly display the character.
Code Output:
In this example, the user pressed the K
key, and the program responded by displaying the pressed key.
The getc
function effectively waits for the user to input a character, allowing the program to pause until a key is pressed. This example illustrates the usage of the getc
function for input handling in C++.
Wait for User Input in C++ Using the system
Function
In C++, another method often used to wait for user input is the system
function.
The system
function is part of the C standard library and is primarily designed for executing shell commands. When used with the argument pause
, it tries to execute the corresponding command, which typically pauses the program execution until the user presses a key.
However, it’s important to note that using system("pause")
for this purpose is considered non-portable and may not work across all platforms. This approach relies on the execution of a shell command to pause the program, making it specific to systems that support the pause
command.
Let’s explore a practical example demonstrating the use of the system
function for waiting for user input:
#include <cstdlib>
int main() {
// Prompting the user
system("echo Press any key to continue...");
// Waiting for user input using system("pause")
system("pause");
return 0;
}
Here, we begin by including the <cstdlib>
header, which is necessary for functions related to the C standard library, including the system
function. Inside the main()
function, no variables are declared; the focus is on using system commands for user interaction.
The line system("echo Press any key to continue...");
employs the system
function to execute the echo
command, displaying the message Press any key to continue..
on the console. This serves as a prompt or instruction for the user, providing a visual cue about the expected interaction.
Following that, the line system("pause");
utilizes the system
function to execute the pause
command. In many Windows environments, the pause
command traditionally pauses the program execution until the user presses a key.
It’s important to emphasize that using system("pause")
is not considered good practice in C++ programming. This method introduces platform-specific behavior and might not work on all systems.
Instead, it’s recommended to utilize more portable and reliable approaches like the ones involving cin.get()
, getchar()
, or getc()
.
Code Output:
Press any key to continue...
Despite its simplicity and convenience, it is strongly recommended to avoid relying on system("pause")
for waiting for user input in C++. Instead, consider using more portable and secure methods discussed earlier in this article.
Conclusion
Waiting for user input is a fundamental aspect of programming, and in C++, we have several methods at their disposal. In this article, we’ve delved into various techniques for accomplishing this task.
From the standard input stream with cin.get()
to character-specific functions like getchar
and getc
, each method has its strengths and use cases.
It’s essential to choose the right approach based on the specific requirements of your program. While cin.get()
is suitable for general input and supports various data types, getchar
and getc
are more character-centric, making them valuable for specific scenarios.
However, developers should be cautious when considering the non-portable option of system("pause")
, as it introduces security risks and may not behave consistently across different platforms.
In C++, the key lies in understanding the trade-offs between simplicity, portability, and security when implementing user input waiting mechanisms. Considerations such as code readability, cross-platform compatibility, and adherence to best practices play a pivotal role in making informed decisions.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook