Attendi l'input dell'utente in C++
-
Usa il metodo
cin.get()
per attendere l’input dell’utente -
Usa la funzione
getchar
per attendere l’input dell’utente -
Usa la funzione
getc
per attendere l’input dell’utente -
Evita di usare
system("pause")
per attendere l’input dell’utente
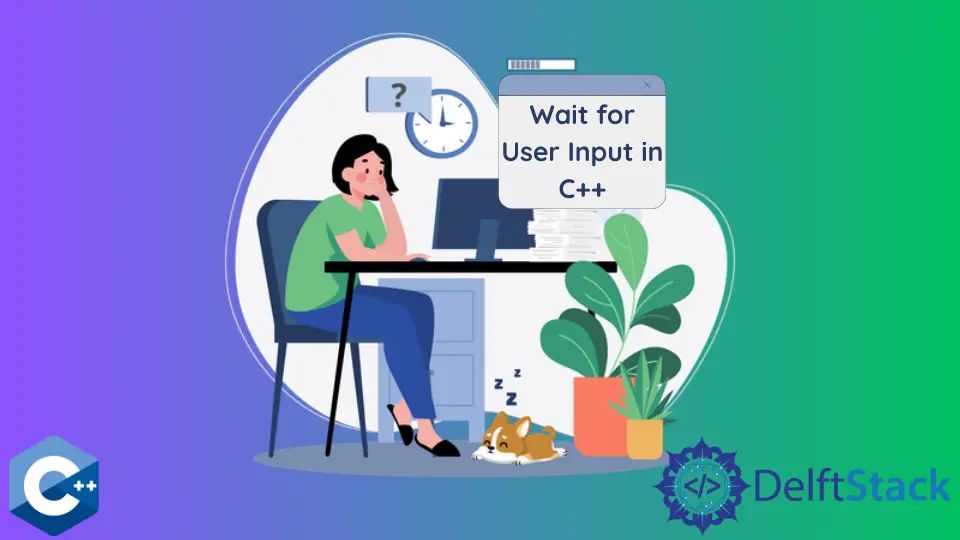
Questo articolo introdurrà i metodi C++ per attendere l’input dell’utente. Si noti che il seguente tutorial presuppone che i contenuti dell’input dell’utente siano irrilevanti per l’esecuzione del programma.
Usa il metodo cin.get()
per attendere l’input dell’utente
get()
è la funzione membro std:cin
, che funziona quasi come un operatore di input >>
che estrae i caratteri dallo stream. In questo caso, quando non siamo interessati a elaborare l’input dell’utente e abbiamo solo bisogno di implementare la funzionalità wait
, possiamo chiamare la funzione get()
senza argomenti. Si noti, tuttavia, che questa funzione ritorna quando viene premuto il tasto Enter.
#include <iostream>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> arr = {'w', 'x', 'y', 'z'};
int flag;
flag = cin.get();
for (auto const& value : arr) cout << value << "; ";
cout << "\nDone !" << endl;
return EXIT_SUCCESS;
}
Produzione:
w; x; y; z;
Done !
Usa la funzione getchar
per attendere l’input dell’utente
La funzione getchar
è la funzione della libreria standard C per leggere un singolo carattere dal flusso di input (stdin
). Come il precedente, questo metodo si aspetta che venga restituito un nuovo carattere di linea (cioè il tasto Enter). getchar
restituisce eof
in caso di errore o quando si incontra la fine del flusso.
#include <iostream>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> arr = {'w', 'x', 'y', 'z'};
int flag;
flag = getchar();
for (auto const& value : arr) cout << value << "; ";
cout << "\nDone !" << endl;
return EXIT_SUCCESS;
}
Usa la funzione getc
per attendere l’input dell’utente
In alternativa, potremmo sostituire l’esempio precedente con la funzione getc
. getc
viene passato all’argomento FILE *stream
per leggere da un dato flusso di input, ma in questo caso stiamo passando stdin
, che è il flusso di input standard comunemente associato all’input del terminale. Questa funzione ritorna anche quando si preme il tasto Enter.
#include <iostream>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> arr = {'w', 'x', 'y', 'z'};
int flag;
flag = getchar();
for (auto const& value : arr) cout << value << "; ";
cout << "\nDone !" << endl;
return EXIT_SUCCESS;
}
Evita di usare system("pause")
per attendere l’input dell’utente
La funzione system
viene utilizzata per eseguire i comandi della shell e il nome del comando viene passato come stringa letterale. Quindi, se una pause
viene passata come argomento, tenta di eseguire il comando corrispondente, che è disponibile solo su piattaforme Windows. È meglio implementare una funzione di attesa personalizzata con i metodi sopra elencati piuttosto che utilizzare un modo non portabile di system("pause")
.
#include <iostream>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> arr = {'w', 'x', 'y', 'z'};
int flag;
system("pause");
for (auto const& value : arr) cout << value << "; ";
cout << "\nDone !" << endl;
return EXIT_SUCCESS;
}
Produzione:
sh: 1: pause: not found
w; x; y; z;
Done !
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook