How to Pause a Program in C++
-
Use the
getc()
Function to Pause a C++ Program -
Use the
std::cin::get()
Method to Pause a C++ Program -
Use the
getchar()
Function to Pause a C++ Program - Use System-Specific Commands to Pause a C++ Program
-
Use the
sleep()
Function to Pause a C++ Program - Conclusion
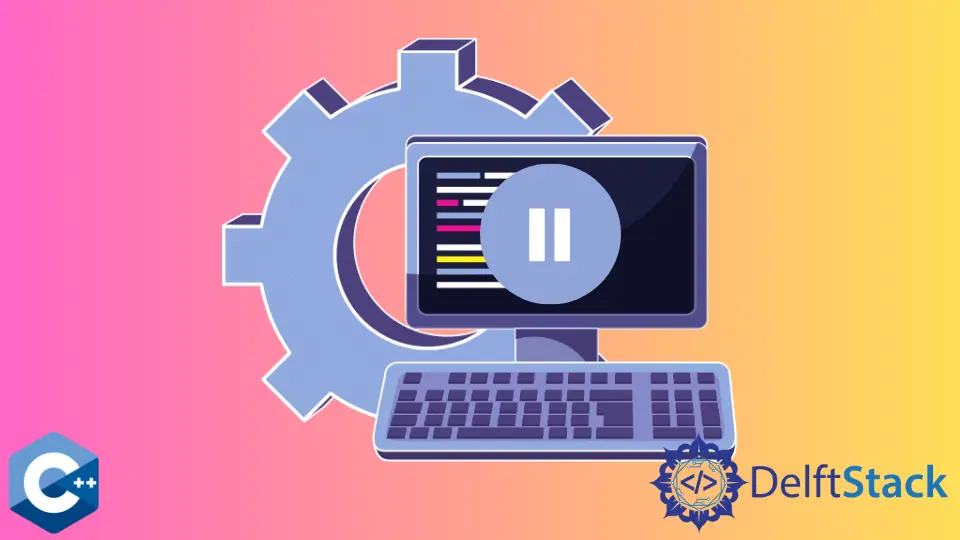
In C++, introducing pauses in a program is a common requirement for diverse reasons – waiting for user input, creating animations, or providing a momentary delay between operations. This article explores various methods to pause a program in C++, showcasing traditional and modern techniques that cater to different scenarios.
Use the getc()
Function to Pause a C++ Program
One effective approach for pausing a C++ program involves utilizing the getc()
function from the C standard input-output library. This function reads the next character from a specified input stream, making it a straightforward choice for pausing the program until user input is received.
The syntax for the getc()
function is as follows:
int getc(FILE* stream);
Here, stream
is a pointer to a FILE
object, representing the input stream from which the function reads the next character. In the context of pausing a program, stdin
(standard input) is commonly used as the input stream, as it corresponds to console input.
The getc()
function returns the integer representation of the character read or EOF
(End-of-File) if the end of the file is reached or an error occurs.
Let’s delve into a complete example demonstrating how to use the getc()
function to pause a C++ program:
#include <chrono>
#include <iostream>
#include <thread>
int main() {
int flag;
std::cout << "Program is paused!\nPress Enter to continue\n";
flag = getc(stdin);
std::cout << "\nContinuing .";
std::this_thread::sleep_for(std::chrono::milliseconds(300));
std::cout << "...\nProgram finished with success code!";
return EXIT_SUCCESS;
}
In this example, we start by including necessary header files, such as <iostream>
for input and output operations and <chrono>
and <thread>
for introducing a delay. The program then declares an integer variable flag
to store the result of the getc()
function.
Next, a message is displayed to inform the user that the program is paused, prompting them to press Enter to continue. The getc(stdin)
line is crucial—it captures a single character of input from the console, effectively pausing the program until the user presses Enter.
Following the user input, a simple animation of dots is displayed to simulate a brief delay in program execution. Finally, a message indicates the successful completion of the program.
The output of this program would look like the following:
Program is paused!
Press Enter to continue
Continuing ....
Program finished with success code!
In this output, the program pauses at the user prompt, waits for input, and then continues with the specified animation before concluding successfully.
Use the std::cin::get()
Method to Pause a C++ Program
Another effective method for pausing a program involves leveraging the std::cin::get()
method. This method of the std::cin
stream extracts characters from the input stream, making it a simple way to pause the program until user input is received.
The syntax for the std::cin::get()
method is as follows:
int_type get();
Here, get()
is a member function of the std::cin
stream, and it reads a single character from the input stream. The return value, int_type
, represents the integral value of the character read.
This method, when used for pausing a program, excels at simplicity by extracting a single character, effectively causing the program to wait until the user provides input.
Let’s explore a complete example demonstrating how to use the std::cin::get()
method to pause a C++ program:
#include <chrono>
#include <iostream>
#include <thread>
int main() {
int flag;
std::cout << "Program is paused!\nPress Enter to continue\n";
flag = std::cin.get();
std::cout << "\nContinuing .";
std::this_thread::sleep_for(std::chrono::milliseconds(300));
std::cout << "...\nProgram finished with success code!";
return EXIT_SUCCESS;
}
In this example, we begin by including necessary header files, such as <iostream>
for input and output operations and <chrono>
and <thread>
for introducing a delay. The program then declares an integer variable flag
to store the result of the std::cin::get()
method.
The user is prompted with a message indicating that the program is paused, with a request to press Enter to continue. The crucial line is flag = std::cin.get();
, which pauses the program until the user provides input.
Subsequently, an animation of dots is displayed to simulate a brief delay in program execution. Finally, a message indicates the successful completion of the program.
The output of this program would appear as follows:
Program is paused!
Press Enter to continue
Continuing ....
Program finished with success code!
In this output, the program pauses at the user prompt, waiting for the user to press Enter. After receiving input, it proceeds with the specified animation before concluding successfully.
Use the getchar()
Function to Pause a C++ Program
The getchar()
function offers yet another way to pause a program effectively. This function is akin to getc(stdin)
and reads the next character from the console input stream.
Using getchar()
provides a concise and widely supported way to introduce a pause in the program until user input is received.
The syntax for the getchar()
function is as follows:
int getchar();
Here, getchar()
is a standard library function that reads a single character from the standard input (stdin
). The function returns the integral representation of the character read, or EOF
(End-of-File), if the end of the file is reached or an error occurs.
In the context of pausing a program, this function is convenient as it reads a character, effectively causing the program to wait until the user provides input.
Let’s dive into a complete example demonstrating how to use the getchar()
function to pause a C++ program:
#include <chrono>
#include <iostream>
#include <thread>
int main() {
int flag;
std::cout << "Program is paused!\nPress Enter to continue\n";
flag = getchar();
std::cout << "\nContinuing .";
std::this_thread::sleep_for(std::chrono::milliseconds(300));
std::cout << "...\nProgram finished with success code!";
return EXIT_SUCCESS;
}
In this example, the necessary header files, including <iostream>
for input and output operations and <chrono>
and <thread>
for introducing a delay, are included. The program declares an integer variable flag
to store the result of the getchar()
function.
The user is presented with a message indicating that the program is paused and a prompt to press Enter to continue. The pivotal line, flag = getchar();
, effectively halts the program until the user provides input.
Following user input, a simple animation of dots is displayed, simulating a brief delay in program execution. Finally, a message indicates the successful completion of the program.
The output of this program would be as follows:
Program is paused!
Press Enter to continue
Continuing ....
Program finished with success code!
In this output, the program halts at the user prompt, waiting for input. After the user presses Enter, the program proceeds with the specified animation before concluding successfully.
Use System-Specific Commands to Pause a C++ Program
Another approach to pausing a program involves using system-specific commands, although it’s worth noting that this method may be less portable across different operating systems.
Here, we’ll explore an example using the std::system("pause")
command, commonly employed on Windows systems. This command initiates a pause, allowing the user to interact with the console window before proceeding.
The syntax for using system-specific commands in C++ is as follows:
int system(const char* command);
Here, command
is a null-terminated string containing the system command to be executed.
In the case of pausing a program on Windows, the command "pause"
is often used. However, it’s important to note that the availability and behavior of system commands can vary across different platforms.
Let’s delve into an example demonstrating how to use system-specific commands to pause a C++ program, specifically using the "pause"
command on Windows:
#include <chrono>
#include <cstdlib>
#include <iostream>
#include <thread>
int main() {
std::cout << "Program is paused!\nPress Enter to continue\n";
std::system("pause");
std::cout << "\nContinuing .";
std::this_thread::sleep_for(std::chrono::milliseconds(300));
std::cout << "...\nProgram finished with success code!";
return EXIT_SUCCESS;
}
In this example, the required header files are included, such as <iostream>
for input and output operations, <cstdlib>
for the std::system
function, and <chrono>
and <thread>
for introducing a delay. The program does not require a variable to store the result, as the system command is used directly.
The user is presented with a message indicating that the program is paused, along with a prompt to press Enter to continue. The necessary line, std::system("pause");
, invokes the system command to pause the program on Windows, allowing the user to interact with the console.
After the system command, a simple animation of dots is displayed, simulating a brief delay in program execution. Finally, a message indicates the successful completion of the program.
The output of this program on a Windows system would look like the following:
Program is paused!
Press Enter to continue
Continuing ....
Program finished with success code!
In this program, the user is prompted to press a key to invoke the system-specific pause command, and then the program proceeds with the specified animation before successfully concluding.
Use the sleep()
Function to Pause a C++ Program
The sleep()
function provides a simple and portable method to introduce a pause in a program by delaying its execution for a specified duration. This approach is versatile and can be particularly useful when a precise time delay is desired between program segments.
The syntax for the sleep()
function is as follows:
#include <chrono>
#include <thread>
void sleep_for(const std::chrono::duration<Rep, Period>& rel_time);
Here, rel_time
represents the relative time duration for which the program execution should be paused. It’s important to include the necessary header files: <thread>
for threading-related functions and <chrono>
for representing time durations.
Let’s explore an example demonstrating how to use the sleep()
function to pause a C++ program.
#include <chrono>
#include <iostream>
#include <thread>
int main() {
std::cout << "Program is paused!\n";
std::this_thread::sleep_for(std::chrono::seconds(5));
std::cout << "Continuing .";
std::this_thread::sleep_for(std::chrono::milliseconds(300));
std::cout << "...\nProgram finished with success code!";
return EXIT_SUCCESS;
}
In this example, the necessary header files, including <iostream>
, <chrono>
, and <thread>
, are included. The program directly specifies the desired pause duration using std::this_thread::sleep_for(std::chrono::seconds(5));
, which pauses the program for 5 seconds.
After the initial pause, a simple animation of dots is displayed, simulating a brief delay in program execution. Finally, a message indicates the successful completion of the program.
The output of this program would look like the following:
Program is paused!
Continuing ...
Program finished with success code!
The program informs the user that it is paused before initiating a 5-second delay using the sleep()
function. After the pause, the program proceeds with the specified animation before concluding successfully.
Conclusion
Controlling program flow through pauses is an art in C++ development, and the methods showcased here offer a spectrum of possibilities. Whether capturing user input, leveraging system commands, or introducing precise delays, developers can choose the approach that aligns with their application’s needs.
Armed with these techniques, learning how to implement program pauses in C++ becomes a key skill for creating responsive and user-friendly applications.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook