如何在 C++ 中暂停程序
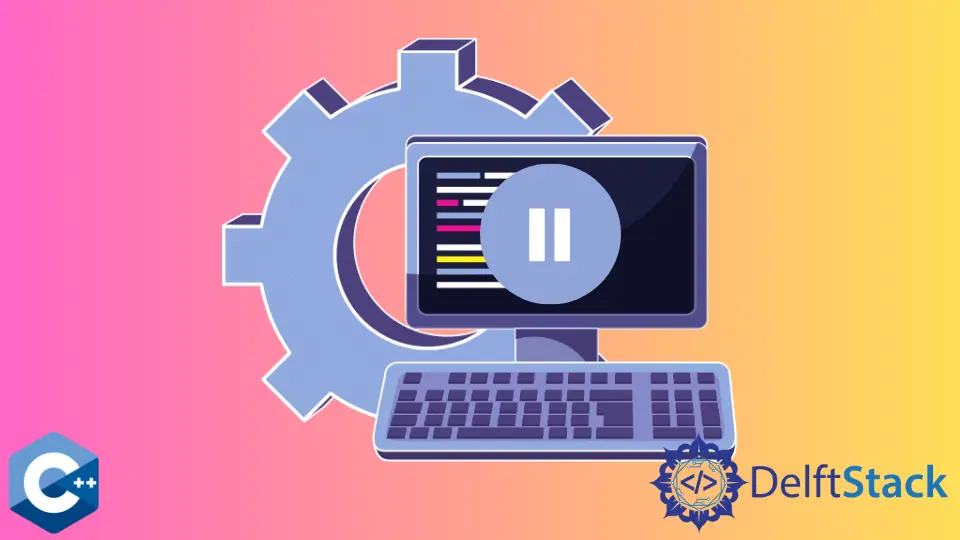
本文将介绍几种在 C++ 中暂停程序的方法。
使用 getc()
函数来暂停程序
getc()
函数来自 C 标准输入输出库,它从给定的输入流中读取下一个字符。输入流的类型为 FILE*
,该函数期望该流会被打开。在几乎所有的 Unix 系统中,在程序启动过程中,有 3 个标准的文件流被打开-即:stdin
、stdout
和 stderr
。在下面的例子中,我们传递 stdin
作为参数,它对应一个控制台输入,以等待用户继续执行程序。
#include <chrono>
#include <iostream>
#include <thread>
using std::copy;
using std::cout;
using std::endl;
using std::this_thread::sleep_for;
using namespace std::chrono_literals;
int main() {
int flag;
cout << "Program is paused !\n"
<< "Press Enter to continue\n";
// pause the program until user input
flag = getc(stdin);
cout << "\nContinuing .";
sleep_for(300ms);
cout << ".";
cout << ".";
cout << ".";
cout << "\nProgram finished with success code!";
return EXIT_SUCCESS;
}
输出:
Program is paused !
Press Enter to continue
Continuing ....
Program finished with success code!
使用 std::cin::get()
方法来暂停程序
暂停程序的另一种方法是调用 std::cin
内置方法 get
,该方法从输入流中提取参数指定的字符。在这种情况下,我们是读取一个字符,然后将控制权返回给正在执行的程序。
#include <chrono>
#include <iostream>
#include <thread>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
using std::this_thread::sleep_for;
using namespace std::chrono_literals;
int main() {
int flag;
cout << "Program is paused !\n"
<< "Press Enter to continue\n";
// pause the program until user input
flag = cin.get();
cout << "\nContinuing .";
sleep_for(300ms);
cout << ".";
cout << ".";
cout << ".";
cout << "\nProgram finished with success code!";
return EXIT_SUCCESS;
}
使用 getchar()
函数来暂停程序的运行
作为另一种方法,我们可以用 getchar
函数调用重新实现同样的功能。getchar
相当于调用 getc(stdin)
,它从控制台输入流中读取下一个字符。
请注意,这两个函数都可能返回 EOF
,表示已到达文件末尾,即没有可读的字符。程序员负责处理任何特殊的控制流和返回的错误代码。
#include <chrono>
#include <iostream>
#include <thread>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
using std::this_thread::sleep_for;
using namespace std::chrono_literals;
int main() {
int flag;
cout << "Program is paused !\n"
<< "Press Enter to continue\n";
// pause the program until user input
flag = getchar();
cout << "\nContinuing .";
sleep_for(300ms);
cout << ".";
cout << ".";
cout << ".";
cout << "\nProgram finished with success code!";
return EXIT_SUCCESS;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe