How to Implement Fizz Buzz Solution in C++
- Use Iterative Method with Literal Values to Implement Fizz Buzz Solution in C++
- Use Custom Class to Implement Fizz Buzz Solution in C++
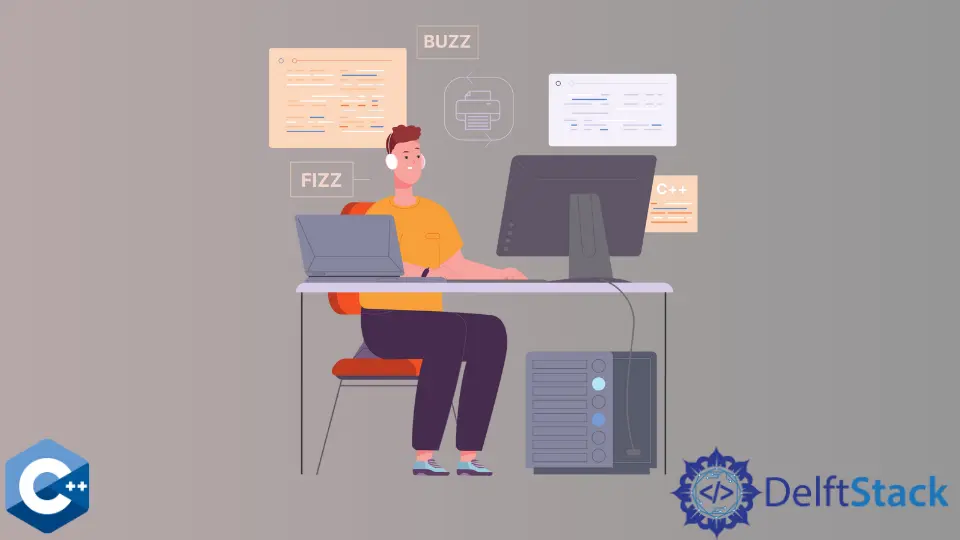
This article will introduce how to implement the Fizz Buzz solution in C++.
Use Iterative Method with Literal Values to Implement Fizz Buzz Solution in C++
Fizz Buzz is a trivial problem used as the programming exercise on training sites or even interviews sometimes. It essentially boils down to printing the numbers from 1
to 100
to the console, except that the multiples of 3
and 5
should be substituted with Fizz
and Buzz
strings, respectively. Additionally, there is a rule for multiples of 15
, which should be printed as FizzBuzz
. The task can be solved with a straightforward loop statement that iterates through the 100-integer range and has if
conditions for 4
different cases in it. Note that, to check if the number is multiple of the given integer, one should use a modulus operator - %
.
#include <iostream>
using std::cout;
using std::endl;
constexpr int COUNT = 100;
int main() {
for (int i = 1; i <= COUNT; ++i) {
if (i % 3 == 0)
cout << "Fizz, ";
else if (i % 5 == 0)
cout << "Buzz, ";
else if (i % 15 == 0)
cout << "FizzBuzz, ";
else
cout << i << ", ";
}
return EXIT_SUCCESS;
}
Output:
1, 2, Fizz, 4, Buzz, Fizz, 7, 8, Fizz, Buzz, 11, Fizz, 13, 14, Fizz, 16, 17, Fizz, 19, Buzz, Fizz, 22, 23, Fizz, Buzz, 26, Fizz, 28, 29, Fizz, 31, 32, Fizz, 34, Buzz, Fizz, 37, 38, Fizz, Buzz, 41, Fizz, 43, 44, Fizz, 46, 47, Fizz, 49, Buzz, Fizz, 52, 53, Fizz, Buzz, 56, Fizz, 58, 59, Fizz, 61, 62, Fizz, 64, Buzz, Fizz, 67, 68, Fizz, Buzz, 71, Fizz, 73, 74, Fizz, 76, 77, Fizz, 79, Buzz, Fizz, 82, 83, Fizz, Buzz, 86, Fizz, 88, 89, Fizz, 91, 92, Fizz, 94, Buzz, Fizz, 97, 98, Fizz, Buzz,
Use Custom Class to Implement Fizz Buzz Solution in C++
Alternatively, we can implement a class that stores the given map of integers and corresponding strings and then call the built-in function to print the values to the console. Note that this method is more generic and can be used to expand the problem definition. FizzBuzz
class has one data member of type map<int, string>
and a constructor that takes a reference to a map. checkFizzBuzz
takes no parameters and executes two nested loops to check for each number in the range of 1-100
.
#include <iostream>
#include <map>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
constexpr int COUNT = 100;
class FizzBuzz {
map<int, string> table;
public:
explicit FizzBuzz(map<int, string> &init) : table(std::move(init)) {}
void checkFizzBuzz() {
for (int i = 1; i <= COUNT; ++i) {
for (const auto &item : table) {
i % item.first == 0 ? cout << item.second << ", " : cout << i << ", ";
break;
}
}
}
};
int main() {
map<int, string> init = {{3, "Fizz"}, {5, "Buzz"}, {15, "FizzBuzz"}};
FizzBuzz fii(init);
fii.checkFizzBuzz();
return EXIT_SUCCESS;
}
Another way to redesign the FizzBuzz
class from the previous example code is to define a constructor that takes an initializer list of integer/string pairs like map
itself. The remaining part of the class stays unchanged. One could also add COUNT
data member to the FizzBuzz
class itself and initialize it using the constructor to allow the user to pass the different numbers as needed.
#include <initializer_list>
#include <iostream>
#include <map>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
constexpr int COUNT = 100;
class FizzBuzz {
map<int, string> table;
public:
FizzBuzz(std::initializer_list<std::pair<int, string>> init) {
for (const auto &item : init) {
table.insert(item);
}
}
void checkFizzBuzz() {
for (int i = 1; i <= COUNT; ++i) {
for (const auto &item : table) {
i % item.first == 0 ? cout << item.second << ", " : cout << i << ", ";
break;
}
}
}
};
int main() {
FizzBuzz fii = {{3, "Fizz"}, {5, "Buzz"}, {15, "FizzBuzz"}};
fii.checkFizzBuzz();
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook