exit(1) in C++
- What is the exit() Function in C++?
- How to Use exit(1) in C++
- Error Handling with exit(1)
- Best Practices for Using exit(1)
- Conclusion
- FAQ
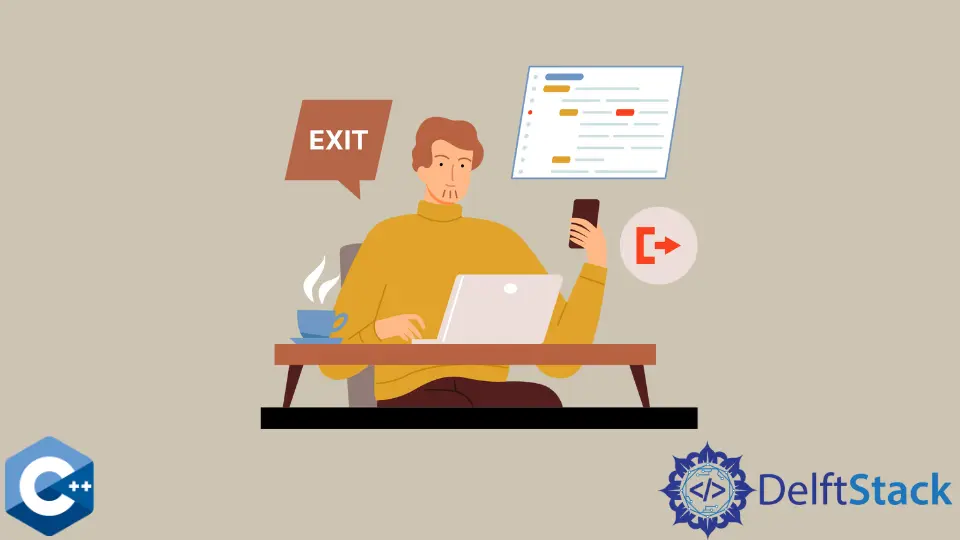
When programming in C++, managing application flow and error handling is crucial. One essential function that developers often use is exit()
. This function is particularly useful when you want to terminate a program prematurely.
In this article, we will explore the exit(1)
function in C++, focusing on its usage and implications. Whether you’re dealing with errors or simply need to exit a program, understanding how to utilize exit()
effectively can enhance your coding skills. We’ll cover practical examples to illustrate its functionality and significance in error handling. So, let’s dive into the world of C++ and see how exit(1)
can be used to manage program termination.
What is the exit() Function in C++?
The exit()
function in C++ is part of the <cstdlib>
library and is used to terminate a program immediately. When you call exit()
, you can pass an integer value as an argument, which indicates the exit status of the program. By convention, an exit status of 0
signifies successful completion, while any non-zero value indicates an error or abnormal termination.
The syntax for using the exit()
function is straightforward:
#include <cstdlib>
void exit(int status);
In this context, exit(1)
is commonly used to signal that the program has encountered an error. This can be particularly useful in scenarios such as command-line applications or scripts where it’s essential to communicate the success or failure of operations back to the operating system or calling processes.
How to Use exit(1) in C++
To demonstrate the use of exit(1)
, let’s look at a simple C++ program that checks for a division by zero error. If such an error occurs, the program will terminate with an exit status of 1
.
#include <iostream>
#include <cstdlib>
int main() {
int numerator = 10;
int denominator = 0;
if (denominator == 0) {
std::cerr << "Error: Division by zero!" << std::endl;
exit(1);
}
std::cout << "Result: " << numerator / denominator << std::endl;
return 0;
}
In this code, we first check if the denominator is zero. If it is, we output an error message to the standard error stream and call exit(1)
to terminate the program. If the denominator is not zero, we would proceed to perform the division.
Output:
Error: Division by zero!
When you run this program, it checks the condition and, upon finding a division by zero, it outputs an error message and exits with a status of 1
. This exit status can be useful for scripts or other programs that may call this application, allowing them to handle the error appropriately.
Error Handling with exit(1)
Using exit(1)
is not just about terminating the program; it’s a critical part of error handling. By specifying an exit code, you provide valuable feedback about the nature of the termination. This can be particularly useful in larger applications or systems where multiple components interact.
Consider a scenario where your program interacts with a database. If a connection fails, you might want to exit with exit(1)
to indicate that something went wrong. Here’s a simplified example:
#include <iostream>
#include <cstdlib>
bool connectToDatabase() {
// Simulated database connection failure
return false;
}
int main() {
if (!connectToDatabase()) {
std::cerr << "Error: Could not connect to the database!" << std::endl;
exit(1);
}
std::cout << "Connected to the database successfully." << std::endl;
return 0;
}
In this example, the connectToDatabase()
function simulates a database connection attempt. If the connection fails, the program outputs an error message and exits with a status of 1
.
Output:
Error: Could not connect to the database!
This approach not only helps in identifying the issue but also allows for better debugging and logging when integrated into larger systems. By using exit(1)
, you can create more robust applications that gracefully handle errors.
Best Practices for Using exit(1)
While using exit(1)
can be beneficial, it’s essential to follow best practices to ensure your program behaves as expected. Here are some tips:
-
Use Meaningful Exit Codes: While
exit(1)
is a standard way to indicate an error, consider defining specific exit codes for different types of errors. This can help in debugging and understanding the error context better. -
Clean Up Resources: Before calling
exit()
, ensure that any resources (like open files or network connections) are properly closed. This prevents resource leaks and ensures that your program leaves the system in a clean state. -
Avoid Overusing exit(): It’s generally better to return from the main function or use exception handling to manage errors rather than exiting abruptly. Reserve
exit()
for critical failures where recovery is not possible. -
Document Exit Codes: If your application will be used by others, document what each exit code means. This practice helps other developers understand how to handle different exit scenarios effectively.
By keeping these best practices in mind, you can use exit(1)
effectively while maintaining the integrity and reliability of your C++ applications.
Conclusion
Understanding how to use exit(1)
in C++ is crucial for effective error handling and program termination. By providing meaningful exit codes, you can enhance the robustness of your applications and facilitate better debugging. Whether you’re working on a simple script or a complex system, mastering the exit()
function will undoubtedly improve your programming skills. Remember to follow best practices to ensure your applications are clean, efficient, and user-friendly. Happy coding!
FAQ
-
What does exit(1) mean in C++?
exit(1) indicates that the program has terminated due to an error. An exit code of 1 is a common convention to signal abnormal termination. -
Can I use exit(0) instead of exit(1)?
Yes, exit(0) indicates successful termination of the program. Use exit(1) for errors and exit(0) for successful completion. -
Is it better to use exit() or return 0 in main?
It depends on the situation. Use return 0 for normal termination and exit() for handling critical errors where you need to terminate immediately. -
How can I check the exit status of a C++ program?
You can check the exit status in a shell by using the commandecho $?
immediately after running the program. -
Are there any alternatives to exit() in C++?
Yes, you can use exception handling to manage errors without terminating the program abruptly, allowing for more graceful error recovery.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook