C++ 中的退出(1)
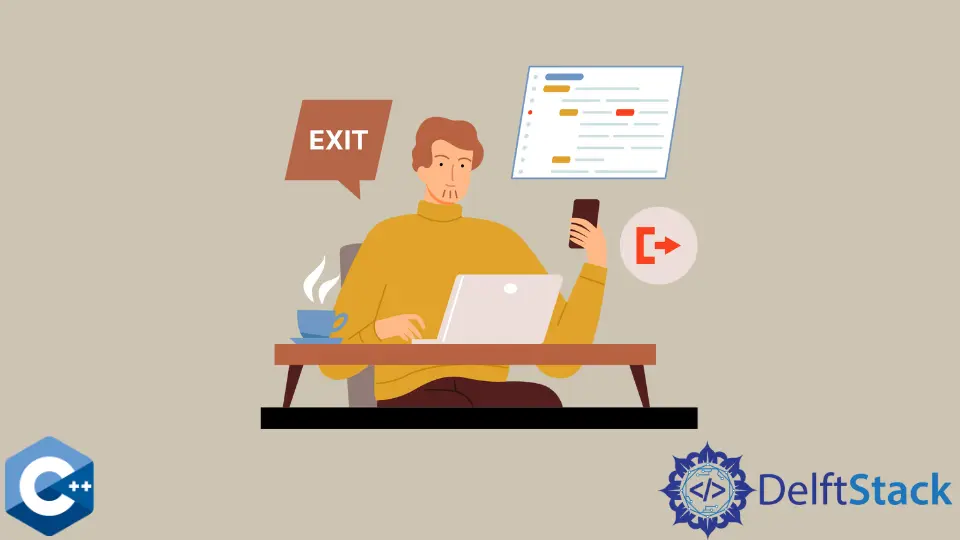
本文将解释如何在 C++ 中使用 exit()
函数。
使用 exit
函数终止带有状态码的 C++ 程序
运行中的程序在操作系统中一般称为进程,它有自己的生命周期,用不同的状态来表示。有像 web 服务器这样的长时间运行的程序,但即使是那些需要在某些情况下或通过传递用户信号来终止的程序。进程终止可以使用 exit()
函数调用,它是 C 标准的一部分并包含在 C++ 中。它需要一个整数参数来指定程序终止状态,如果子进程被等待,可以从父进程读取该状态。
成功返回状态码的常规值为 0
,除零之外的任何值都被视为错误代码,这可能对应于预定义的场景。请注意,有两个宏表达式分别称为 EXIT_SUCCESS
和 EXIT_FAILURE
来表示传递给 exit
调用的相应退出代码。通常,程序有多个文件流和临时文件打开,当调用 exit
时,它们会自动关闭或删除。
#include <unistd.h>
#include <iostream>
using std::cout;
using std::endl;
int main() {
printf("Executing the program...\n");
sleep(3);
exit(0);
// exit(EXIT_SUCCESS);
}
输出:
Executing the program...
使用 exit(1)
终止带有失败状态代码的 C++ 程序
参数值为 1
的 exit
函数用于表示失败终止。某些返回成功调用状态代码值的函数可以与验证返回值并在发生错误时退出程序的条件语句结合使用。请注意,exit(1)
与调用 exit(EXIT_FAILURE)
相同。
#include <unistd.h>
#include <iostream>
using std::cout;
using std::endl;
int main() {
if (getchar() == EOF) {
perror("getline");
exit(EXIT_FAILURE);
}
cout << "Executing the program..." << endl;
sleep(3);
exit(1);
// exit(EXIT_SUCCESS);
}
输出:
Executing the program...
exit()
函数的另一个有用功能是在程序最终终止之前执行专门注册的函数。这些函数可以定义为常规函数,要在终止时调用,它们需要使用 atexit
函数注册。atexit
是标准库的一部分,以函数指针作为唯一参数。请注意,可以使用多个 atexit
调用注册多个函数,并且一旦 exit
执行,它们中的每一个都会以相反的顺序调用。
#include <unistd.h>
#include <iostream>
using std::cout;
using std::endl;
static void at_exit_func() { cout << "at_exit_func called" << endl; }
static void at_exit_func2() { cout << "at_exit_func called" << endl; }
int main() {
if (atexit(at_exit_func) != 0) {
perror("atexit");
exit(EXIT_FAILURE);
}
if (atexit(at_exit_func2) != 0) {
perror("atexit");
exit(EXIT_FAILURE);
}
cout << "Executing the program..." << endl;
sleep(3);
exit(EXIT_SUCCESS);
}
输出:
Executing the program...
at_exit_func2 called
at_exit_func called
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe