How to Use Dynamic Cast in C++
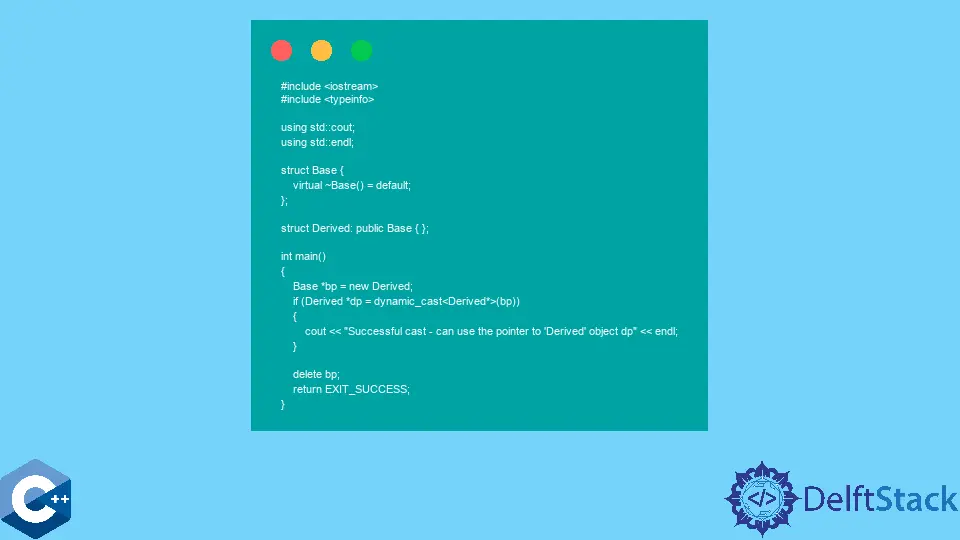
Dynamic casting in C++ is a powerful feature that allows you to safely convert pointers and references within an inheritance hierarchy.
This article introduces how to use dynamic cast in C++, explaining its importance, syntax, and practical examples. If you’re developing applications that rely on polymorphism, understanding dynamic cast is essential. It ensures that you can safely downcast or upcast between classes while maintaining type safety. In this guide, we will explore the syntax of dynamic cast, provide practical examples, and discuss when to use it effectively.
Use dynamic_cast
to Convert From Base Class Pointer to Derived One
dynamic_cast
allows the programmer to convert pointers and references to classes across the inheritance hierarchy. As an example, the base class pointer can be cast into a derived class pointer and allow the programmer to call derived class member functions.
dynamic_cast
is part of the Run-Time Type Information (RTTI) feature that provides a way to access the type of an object at run time instead of compile time.
Mind that dynamic_cast
can not be used to convert between the primitive types like int
or float
. Additionally, one should only use dynamic_cast
when the base class contains at least one virtual member function. In the following example, we declare a new Base
class pointer and cast it into the new pointer to the Derived
class. Since dynamic_cast
returns a null pointer, if the cast is unsuccessful, we can put the expression in the if
statement as a condition.
#include <iostream>
#include <typeinfo>
using std::cout;
using std::endl;
struct Base {
virtual ~Base() = default;
};
struct Derived : public Base {};
int main() {
Base *bp = new Derived;
if (Derived *dp = dynamic_cast<Derived *>(bp)) {
cout << "Successful cast - can use the pointer to 'Derived' object dp"
<< endl;
}
delete bp;
return EXIT_SUCCESS;
}
Output:
Successful cast - can use the pointer to 'Derived' object dp
The programmer can safely access the object in the if
section scope and call Derived
class member methods as needed. Note though, that since we are casting Derived
class type to Base
, on the 13th line, we specify Derived
after new
operator as the following code would yield unsuccessful cast:
#include <iostream>
#include <typeinfo>
using std::cout;
using std::endl;
struct Base {
virtual ~Base() = default;
};
struct Derived : public Base {};
int main() {
Base *bp = new Base;
if (Derived *dp = dynamic_cast<Derived *>(bp)) {
cout << "Successful cast - can use the pointer to 'Derived' object dp"
<< endl;
}
delete bp;
return EXIT_SUCCESS;
}
The other part of the RTTI feature is the typeid
operator, which returns the type of the given expression. It can be utilized to compare types of multiple expressions, as demonstrated in the next code sample. Note that, <typeinfo>
header must be included when using the typeid
operator.
#include <iostream>
#include <typeinfo>
using std::cout;
using std::endl;
struct Base {
virtual ~Base() = default;
};
struct Derived : public Base {};
int main() {
Base *bp = new Derived;
if (Derived *dp = dynamic_cast<Derived *>(bp)) {
cout << "Successful cast - can use the pointer to 'Derived' object dp"
<< endl;
if (typeid(*bp) == typeid(*dp)) {
cout << "bp and dp are of same type" << endl;
}
}
delete bp;
return EXIT_SUCCESS;
}
Output:
Successful cast - can use the pointer to 'Derived' object dp
bp and dp are of same type
Conclusion
Dynamic casting in C++ is an essential feature for developers working with polymorphic types. By utilizing dynamic_cast, you can ensure that your type conversions are safe and valid, preventing runtime errors that could arise from invalid casts. This article has covered the syntax, provided practical examples, and discussed the pros and cons of using dynamic_cast. Understanding how to effectively use dynamic_cast will enhance your programming skills and improve the robustness of your C++ applications.
FAQ
-
what is dynamic cast in C++?
Dynamic cast is a C++ operator that safely converts pointers and references in an inheritance hierarchy, providing runtime type checking. -
when should I use dynamic cast?
Use dynamic cast when you need to safely downcast or upcast between classes in a polymorphic inheritance hierarchy. -
what happens if dynamic cast fails?
If dynamic cast fails, it returns a null pointer for pointers or throws a std::bad_cast exception for references. -
how does dynamic cast differ from static cast?
Dynamic cast performs runtime checks and ensures type safety, while static cast does not perform any checks and can lead to unsafe conversions. -
can I use dynamic cast with non-polymorphic types?
No, dynamic cast can only be used with polymorphic types, meaning classes that have at least one virtual function.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook