C++에서 동적 주물 사용
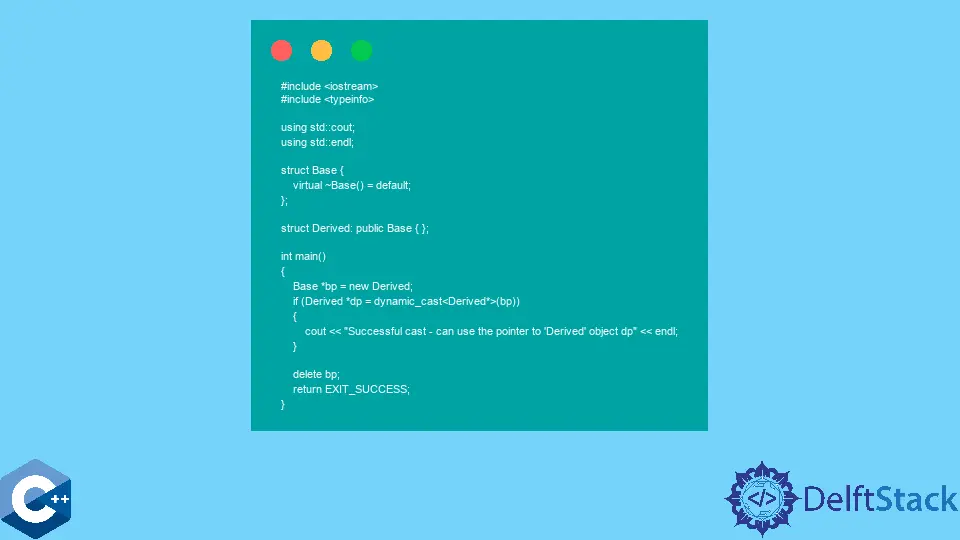
이 기사에서는 C++에서 동적 캐스트를 사용하는 방법에 대한 여러 방법을 보여줍니다.
dynamic_cast
를 사용하여 기본 클래스 포인터에서 파생 된 포인터로 변환
dynamic_cast
를 사용하면 프로그래머가 상속 계층 구조에서 클래스에 대한 포인터와 참조를 변환 할 수 있습니다. 예를 들어, 기본 클래스 포인터를 파생 클래스 포인터로 캐스팅하고 프로그래머가 파생 클래스 멤버 함수를 호출 할 수 있습니다.
dynamic_cast
는 컴파일 타임이 아닌 런타임에 객체 유형에 액세스하는 방법을 제공하는 RTTI
(Run-Time Type Information) 기능의 일부입니다.
dynamic_cast
는int
또는float
와 같은 기본 유형간에 변환하는 데 사용할 수 없습니다. 또한 기본 클래스에 가상 멤버 함수가 하나 이상 포함되어있는 경우에만 dynamic_cast
를 사용해야합니다. 다음 예제에서는 새로운Base
클래스 포인터를 선언하고Derived
클래스에 대한 새 포인터로 캐스팅합니다. dynamic_cast
는 null
포인터를 반환하므로 캐스트가 실패하면 표현식을if
문에 조건으로 넣을 수 있습니다.
#include <iostream>
#include <typeinfo>
using std::cout;
using std::endl;
struct Base {
virtual ~Base() = default;
};
struct Derived : public Base {};
int main() {
Base *bp = new Derived;
if (Derived *dp = dynamic_cast<Derived *>(bp)) {
cout << "Successful cast - can use the pointer to 'Derived' object dp"
<< endl;
}
delete bp;
return EXIT_SUCCESS;
}
출력:
Successful cast - can use the pointer to 'Derived' object dp
프로그래머는if
섹션 범위의 객체에 안전하게 액세스하고 필요에 따라Derived
클래스 멤버 메서드를 호출 할 수 있습니다. 단, 우리는Derived
클래스 유형을Base
로 캐스팅하고 있기 때문에 13 번째 줄에서new
연산자 뒤에Derived
를 지정합니다. 다음 코드는 실패한 캐스팅을 생성합니다.
#include <iostream>
#include <typeinfo>
using std::cout;
using std::endl;
struct Base {
virtual ~Base() = default;
};
struct Derived : public Base {};
int main() {
Base *bp = new Base;
if (Derived *dp = dynamic_cast<Derived *>(bp)) {
cout << "Successful cast - can use the pointer to 'Derived' object dp"
<< endl;
}
delete bp;
return EXIT_SUCCESS;
}
RTTI 기능의 다른 부분은 주어진 표현식의 유형을 반환하는typeid
연산자입니다. 다음 코드 샘플에서 설명하는 것처럼 여러 표현식의 유형을 비교하는 데 사용할 수 있습니다. typeid
연산자를 사용할 때는<typeinfo>
헤더를 포함해야합니다.
#include <iostream>
#include <typeinfo>
using std::cout;
using std::endl;
struct Base {
virtual ~Base() = default;
};
struct Derived : public Base {};
int main() {
Base *bp = new Derived;
if (Derived *dp = dynamic_cast<Derived *>(bp)) {
cout << "Successful cast - can use the pointer to 'Derived' object dp"
<< endl;
if (typeid(*bp) == typeid(*dp)) {
cout << "bp and dp are of same type" << endl;
}
}
delete bp;
return EXIT_SUCCESS;
}
출력:
Successful cast - can use the pointer to 'Derived' object dp
bp and dp are of same type
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook