Virtual Variable in C++
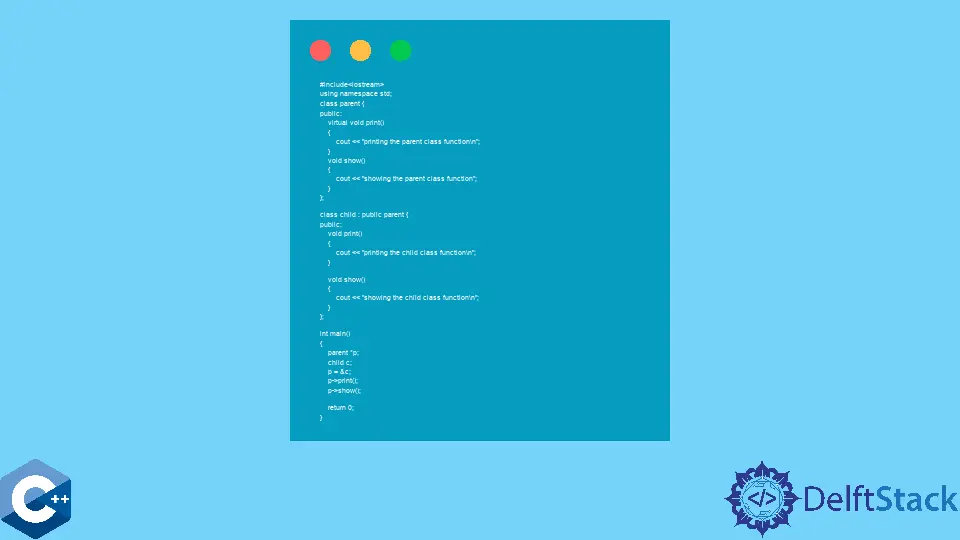
Polymorphism is one of the pillars of Object Oriented Programming in C++ that can display a certain message in more than one form. We have two types of Polymorphism - Compile time and runtime- achieved by function overloading and function overriding, respectively.
However, function overriding involves the usage of the virtual
keyword in C++. This article discusses the virtual variables in C++.
Virtual Variable in C++
Polymorphism can be described as something that has many forms. A real-world example of polymorphism can be a woman who is a mother, daughter, sister, and daughter-in-law, at the same time.
However, from a programming point of view, polymorphism can be achieved by using a member function with the same or different type more than once for different purposes.
There are two types of polymorphism - Compile time, also known as early binding, is achieved by function overloading or operator overloading. In simple terms, it’s the ability to use a function with the same name but with a different number or type of argument.
On the other hand, runtime polymorphism is achieved by function overriding.
Function overriding is the ability to use a function with the same name, type, and several arguments. It is achieved using the virtual
keyword in C++.
For implementing function overriding, we make virtual functions in C++; however, no such concept named virtual variables exists in C++.
Virtual functions are the ones that permit polymorphism and not virtual variables. A virtual function is a member function declared in the base class and overridden in the derived class.
Whenever we refer to a child class object using a parent class pointer, we can call a virtual function for that object and execute the child class version (derived class version) of that function.
In C++, virtual functions let two classes of different types be treated as the same by the calling code, with the differences in the internal behavior of the classes being encapsulated in the virtual functions. However, in the case of virtual variables, behavior is encapsulated by accessing a variable.
However, you can perform polymorphism by simply using the virtual function in C++. Let us see the code for the same.
#include <iostream>
using namespace std;
class parent {
public:
virtual void print() { cout << "printing the parent class function\n"; }
void show() { cout << "showing the parent class function"; }
};
class child : public parent {
public:
void print() { cout << "printing the child class function\n"; }
void show() { cout << "showing the child class function\n"; }
};
int main() {
parent *p;
child c;
p = &c;
p->print();
p->show();
return 0;
}
Output:
printing the child class function
showing the parent class function
In the above code example, since we are referencing the child class using the parent class pointer, we can call the virtual function for that object and execute the child class version of the function.
Therefore, we have made the print
function of the parent class virtual, after which the function that is executed by calling the print()
function is the one that is in the child class, whereas the show()
function, which is not virtual, still prints the parent version of the function.
Conclusion
In this article, we have discussed polymorphism, virtual functions, and virtual variables in C++. Polymorphism is described in many forms and has two types - Runtime and Compile time.
However, virtual functions are the ones that are used to implement runtime polymorphism in C++ by making a function virtual. But there is nothing called virtual variables in C++ as there is no behavior to encapsulate by accessing a variable.