Reflection in C++
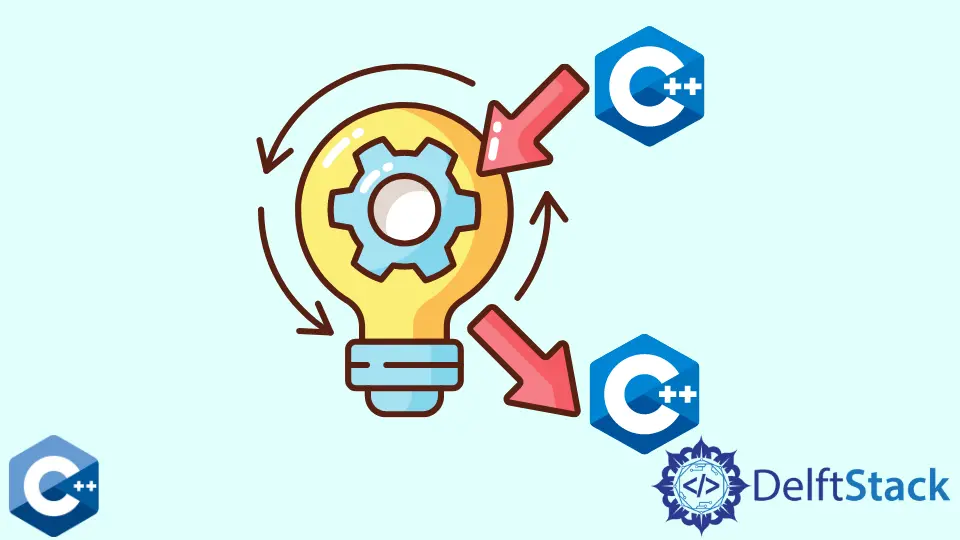
This article will discuss Reflections
, purpose, and implementation in C++. We will further look into the pros and cons of using reflections.
Reflection in C++
Reflection
is a programming mechanism that allows you to write generic code that works with any object type. It helps to detect the format of class objects at runtime and invoke their methods or access their data.
The reflections are useful in invoicing remote methods where a descriptor for that class is returned (containing information about the class, variables, and methods). These descriptors make it simple to call instance methods and access their variables.
Reflection has been implemented to C++ later using RTTI; however, it only has a few restrictions.
Below is the code snippet showing the syntax of Reflection
.
class demo {
public:
int a;
char* p;
double d;
protected:
long my_array[10];
int** p1;
public:
RTTI_DESCRIBE_STRUCT((RTTI_FIELD(a, RTTI_FLD_PUBLIC),
RTTI_PTR(p, RTTI_FLD_PUBLIC),
RTTI_FIELD(d, RTTI_FLD_PUBLIC),
RTTI_ARRAY(my_array, RTTI_FLD_PROTECTED),
RTTI_PTR_TO_PTR(p1, RTTI_FLD_PROTECTED)));
};
The above reflection
syntax describes a class descriptor using various flags. As can be seen, two types of macros are employed.
RTTI DESCRIBE STRUCT
- This helps in defining the class components. It is specified in the declaration of the class.
The macros below can be used to describe a class.
RTTI_FIELD
- This field specifies if the scalar or structure is a scalar or a structure.RTTI_PTR
- This field describes the pointer to the mentioned scalar or structure.RTTI_PTR_PTR
- The macroPTR TO PTR
is a double pointer to theRTTI_FIELD
macro.RTTI_ARRAY
- This macro creates one-dimensional scalar, class, or structure arrays.
The class’ second parameter asks for flags or qualifiers for those fields. Some of the flags used in the above macros are listed below.
RTTI_FLD_INSTANCE
RTTI_FLD_STATIC
RTTI_FLD_CONST
RTTI_FLD_PUBLIC
RTTI_FLD_PROTECTED
RTTI_FLD_PRIVATE
RTTI_FLD_VIRTUAL
RTTI_FLD_VOLATILE
RTTI_FLD_TRANSIENT
How Reflections Work in C++
When using reflection in C++, one can quickly determine whether or not the expression used in the application is valid. Moreover, it further helps in determining whether the object contains the indicated member variable/method or not.
The reflection API collects all of the information while the program is running and creates a class descriptor containing all of the information about the class’s member variables and methods.
The compiler uses this class descriptor to determine whether variables and methods belong to that specific class and whether the given expression is legitimate.
There are several methods in C++ to determine the format of an object during runtime, including:
- Examine the debugging data.
- Using custom preprocessors that parse C++ sources to generate class descriptors.
- The programmer has to do it manually.
Pros and Cons of Reflection
- Debugging information extraction - Using the
reflection
API, it becomes easier to extract all relevant information about the types of objects utilized in the application. When using this functionality, one must be careful not to modify the application. - No additional steps are necessary - When employing reflection features to obtain information about the object’s format type, no additional procedures are required to produce runtime type information.
- Efficient code generation - An application’s
Reflection
API aids in generating efficient code for reflection methods. - Access variables and instance methods - Using the descriptor returned by the
reflection
API in a class, one can easily access the class variables and activate the class instance methods.
A reflection is a useful tool for determining the type of an object during runtime. This information is useful for activities like an invocation, debugging, remote methods, serialization, and so on.
This is also useful for finding an item in an application by its name or if you need to iterate over all the components.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn