C++ Getters and Setters
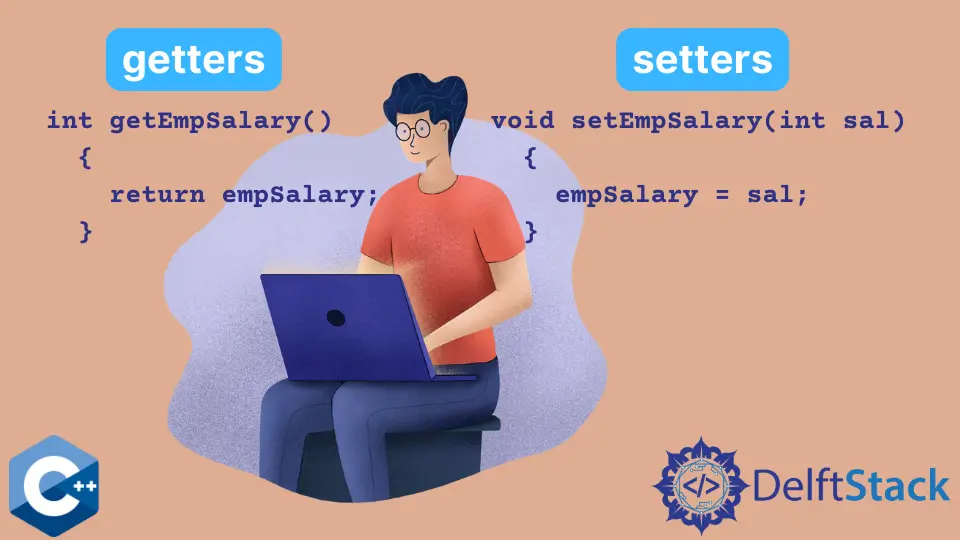
This trivial guide will first briefly introduce the concept of encapsulation and data hiding in object-oriented programming. Then will move on to the use of getters and setters in C++.
What is Encapsulation
Encapsulation is the concept of binding all relevant things together. A class in C++ can combine all the related data into a single capsule.
For example, assume we have an object or entity Rectangle
with some attributes and properties. We can combine all its attributes and properties in a single class.
Therefore, encapsulation is known to combine related data and methods in a single class. It is often confused with the concept of data hiding.
Data Hiding is the concept that the attributes belonging to a class should not be accessible to everyone outside the class. This is because it violates data security.
If data members of a class are made private, any user outside the class cannot access it, and therefore we can achieve data security.
By doing this, although we cannot set the value or get the value of data members from outside the class, we can create methods that can be used to access the members, and these methods ensure that some unwanted data is not placed in the data members.
Such methods for setting or getting the value of data members are called getters
and setters
.
Getters and Setters in C++
This is a good programming practice to make data members of a class private so that invalid data cannot be assigned to the data members of the class. With this, you can make checks on the data coming in the data members before storing that in the data members.
For example, we have a class, Shape
. The length attribute of any shape can never be negative.
Therefore, while setting the value of length, we will check whether or not the provided value is greater than zero.
Similarly, if we have an Employee
class, that has an attribute of salary
. This attribute has neither a negative nor a very large positive value.
Hence, a validator must be placed before setting the value of salary
. Let’s look at the example below.
class Emp {
private:
int empSalary;
public:
void setEmpSalary(int sal) {
if (sal > 0 and sal < 10000000) empSalary = sal;
}
int getEmpSalary() { return empSalary; }
};
In this code snippet, we have data member salary
, which is private to achieve data hiding. Moreover, we have provided a getter and setter for this data member so that the value can be set and retrieved when required.
In the setter function, we also created a check to control the invalid value entered in the salary
data member. This is how to create setters and getters for any data member you require.
Let’s look at the complete code for the above example.
#include <iostream>
#include <string>
using namespace std;
class Emp {
private:
int empSalary;
string empName;
public:
Emp(string n, int s) {
setEmpName(n);
setEmpSalary(s);
}
void setEmpName(string s) { empName = s; }
string getEmpName() { return empName; }
void setEmpSalary(int sal) {
if (sal > 0 and sal < 10000000) empSalary = sal;
}
int getEmpSalary() { return empSalary; }
};
int main() {
Emp e("John", 50000);
cout << "Name: " << e.getEmpName() << endl;
cout << "Salary: " << e.getEmpSalary() << endl;
}
Output:
Name: John
Salary: 50000
Conclusion
The addition of getter and setter methods to the class interface is critical. Because a class’ member variables will be declared private, your class’ users will require a way to retrieve and set their values.
Because setter methods can include data validation code to ensure that member variables are set to legitimate values, getter and setter functions enable this access securely.
Of course, there are times when you don’t want to offer getter, setter, or both methods for all of a class’ member variables. Read-only member variables are variables that can be obtained but not changed.
One such example can be some static data members, or there can be some read-only data members; for such members, we need a getter function but not a setter function.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn