How to Get Mouse Position in C++
- Using the Windows API
- Using SDL (Simple DirectMedia Layer)
- Using SFML (Simple and Fast Multimedia Library)
- Conclusion
- FAQ
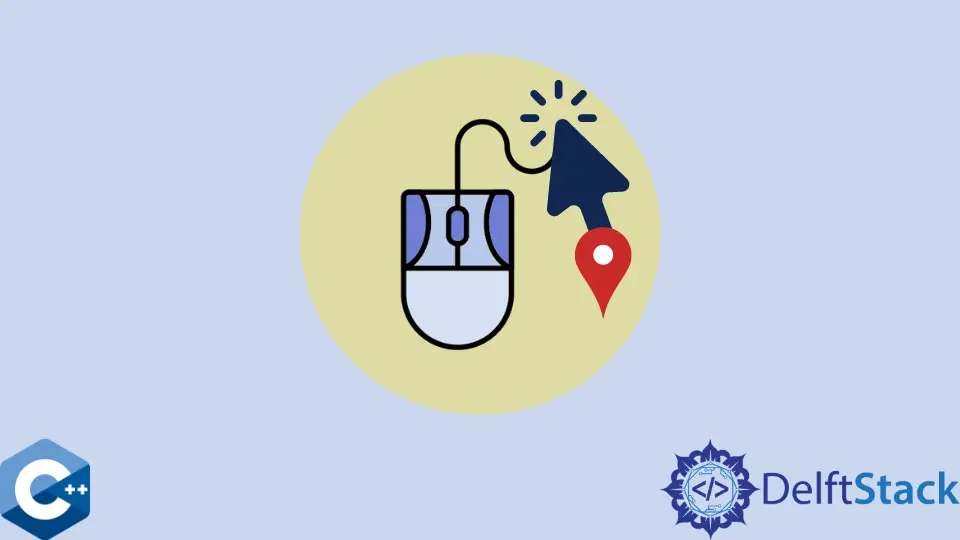
In the world of programming, understanding how to interact with user input is crucial, especially when it comes to graphical applications. One common task is retrieving the mouse position in C++. Whether you’re developing a game, a user interface, or any application that requires mouse tracking, knowing how to obtain the mouse coordinates can significantly enhance user experience.
In this tutorial, we will explore various methods to get the mouse position in C++ using different libraries and techniques. By the end of this guide, you will have a solid understanding of how to retrieve mouse coordinates effectively.
Using the Windows API
If you are developing on a Windows platform, one of the simplest ways to get the mouse position is by using the Windows API. This approach allows you to access the current position of the mouse cursor directly.
Here’s a straightforward example of how to do this:
#include <windows.h>
#include <iostream>
int main() {
POINT mousePos;
if (GetCursorPos(&mousePos)) {
std::cout << "Mouse Position: (" << mousePos.x << ", " << mousePos.y << ")\n";
}
return 0;
}
Output:
Mouse Position: (x, y)
In this code snippet, we include the windows.h
header to access the necessary Windows API functions. The GetCursorPos
function retrieves the current position of the mouse cursor and stores it in a POINT
structure, which contains x
and y
coordinates. If the function call is successful, we print the mouse position to the console. This method is efficient and works seamlessly on Windows systems, making it a preferred choice for many developers.
Using SDL (Simple DirectMedia Layer)
SDL is a popular library for multimedia applications, especially games. It provides a cross-platform way to handle graphics, sound, and user input, including mouse position tracking.
Here’s how to get the mouse position using SDL:
#include <SDL.h>
#include <iostream>
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("Mouse Position", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 640, 480, SDL_WINDOW_SHOWN);
SDL_Event event;
while (true) {
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
if (event.type == SDL_MOUSEMOTION) {
int x, y;
SDL_GetMouseState(&x, &y);
std::cout << "Mouse Position: (" << x << ", " << y << ")\n";
}
}
}
return 0;
}
Output:
Mouse Position: (x, y)
In this example, we initialize SDL and create a window. We enter a loop where we continuously check for events. When a mouse motion event is detected, we call SDL_GetMouseState
, which retrieves the current mouse position. This method is advantageous because it works across multiple platforms, making your application more versatile.
Using SFML (Simple and Fast Multimedia Library)
SFML is another excellent library for handling graphics and input in C++. It provides a simple interface for managing windows, graphics, and user input, including mouse tracking.
Here’s how to get the mouse position with SFML:
#include <SFML/Graphics.hpp>
#include <iostream>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Mouse Position");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
if (event.type == sf::Event::MouseMoved) {
std::cout << "Mouse Position: (" << event.mouseMove.x << ", " << event.mouseMove.y << ")\n";
}
}
window.clear();
window.display();
}
return 0;
}
Output:
Mouse Position: (x, y)
In this SFML example, we create a render window and enter a loop to process events. When the mouse is moved, we capture its position directly from the event
object. This method is user-friendly and integrates well with graphics, making it an excellent choice for game development.
Conclusion
Retrieving the mouse position in C++ is a fundamental skill for developers working on interactive applications. Whether you choose to use the Windows API, SDL, or SFML, each method has its advantages and is suited for different types of projects. Understanding these techniques will empower you to create more engaging and responsive applications. As you experiment with these libraries, consider how mouse input can enhance your user experience and open up new possibilities in your software development journey.
FAQ
- How do I get the mouse position in a console application?
You can use the Windows API to get the mouse position in a console application by calling theGetCursorPos
function.
-
Is SDL cross-platform?
Yes, SDL is designed to be cross-platform, allowing you to develop applications for Windows, macOS, and Linux. -
Can I use SFML for game development?
Absolutely! SFML is widely used for game development due to its simplicity and ease of use in handling graphics and input. -
Do I need to install additional libraries for these methods?
Yes, for SDL and SFML, you will need to install the respective libraries and link them to your project. -
What is the best method to track mouse movement in a game?
Using SDL or SFML is recommended for game development, as they provide robust event handling and graphics capabilities.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook