C++ Escape Characters
- What are Escape Characters in C++?
- Using Escape Characters in C++
- Special Characters and Their Uses
- Multi-line Strings with Escape Characters
- Best Practices for Using Escape Characters
- Conclusion
- FAQ
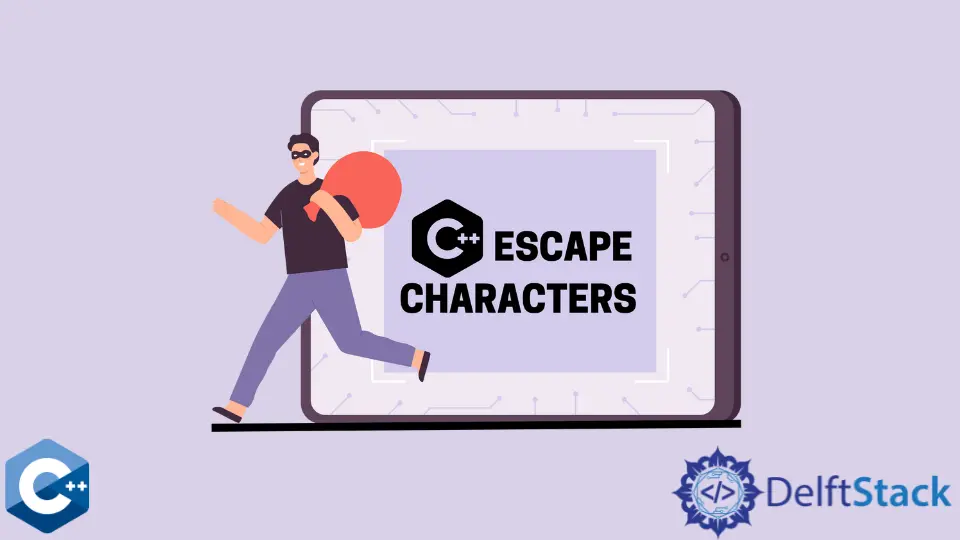
In the world of programming, understanding the nuances of string manipulation is crucial, especially in C++. One of the essential aspects of working with strings in C++ is the use of escape characters. These special characters allow you to include characters in strings that would otherwise be difficult or impossible to represent. Whether you want to include a newline, a tab, or even a quotation mark in your strings, knowing how to use escape characters effectively can save you a lot of time and frustration.
In this article, we will explore the rules for escape characters in C++, providing clear examples and explanations to help you master this fundamental concept.
What are Escape Characters in C++?
Escape characters are special sequences in string literals that begin with a backslash (). They enable you to represent characters that have specific meanings in C++ or that are otherwise difficult to include directly in strings. For example, if you want to include a quotation mark in your string, you can’t just type it out because it would terminate the string. Instead, you use the escape sequence ".
Here are some common escape characters used in C++:
- \n: Newline
- \t: Tab
- ": Double quotation mark
- \: Backslash
- \r: Carriage return
- \a: Alert (bell)
Understanding these escape characters allows you to format strings in a way that enhances readability and functionality.
Using Escape Characters in C++
When you use escape characters in C++, they alter how the string is interpreted by the compiler. Let’s take a look at a basic example to illustrate this point.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!\n";
cout << "This is a tab:\tTabbed text.\n";
cout << "This is a quote: \"Quoted text.\"\n";
return 0;
}
Output:
Hello, World!
This is a tab: Tabbed text.
This is a quote: "Quoted text."
In this example, we see several escape characters in action. The \n creates a new line after “Hello, World!”, while \t adds a tab space before “Tabbed text.” Finally, the " allows us to include quotation marks within the string without ending it prematurely.
Using escape characters in this way makes your output more organized and readable, which is especially useful in larger applications where clarity is key.
Special Characters and Their Uses
C++ escape characters are not just limited to formatting text; they also include some special characters that can be useful in various programming scenarios. For instance, the backslash itself can be included in a string using the escape sequence \.
Here’s how you can use some of these special characters in a C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "File path: C:\\Program Files\\MyApp\n";
cout << "Alert sound will play now:\a\n";
cout << "Carriage return example: Hello\rWorld\n";
return 0;
}
Output:
File path: C:\Program Files\MyApp
Alert sound will play now:
Carriage return example: Hello
World
In this code, we demonstrate the use of \ to represent a backslash in a file path. The \a triggers an alert sound (if your system supports it), and \r moves the cursor back to the beginning of the line, allowing “World” to overwrite “Hello.” These examples show how escape characters can be used creatively to manipulate output.
Multi-line Strings with Escape Characters
Escape characters can also be beneficial when dealing with multi-line strings. Although C++ does not have built-in support for multi-line string literals like some other languages, you can achieve similar results using escape characters for newlines.
Here’s an example of how to create a multi-line string using escape characters:
#include <iostream>
using namespace std;
int main() {
cout << "This is a multi-line string:\n"
<< "Line 1\n"
<< "Line 2\n"
<< "Line 3\n";
return 0;
}
Output:
This is a multi-line string:
Line 1
Line 2
Line 3
In this example, we concatenate multiple strings using the newline escape character \n. This method allows you to create a visually structured output without cluttering your code with multiple cout statements.
Best Practices for Using Escape Characters
While escape characters are powerful tools, it’s essential to use them judiciously to maintain code readability. Here are some best practices to consider:
- Keep It Simple: Avoid overusing escape characters in a single string. If your string becomes too complex, consider breaking it into smaller parts.
- Comment Wisely: When using escape characters, consider adding comments to clarify their purpose, especially if the meaning isn’t immediately obvious.
- Consistent Formatting: Use consistent formatting for your strings. For example, if you start using escape characters for newlines, try to stick with that approach throughout your code.
By following these best practices, you can ensure that your code remains clean and maintainable while effectively utilizing escape characters.
Conclusion
Escape characters are an essential part of working with strings in C++. Understanding how to use them effectively can greatly enhance your programming skills and improve the readability of your code. From formatting text with newlines and tabs to including special characters, mastering escape characters allows you to create more dynamic and user-friendly applications. As you continue to explore C++, keep these rules in mind, and you’ll find that your ability to manipulate strings will become a powerful asset in your programming toolkit.
FAQ
-
What are escape characters in C++?
Escape characters are special sequences that begin with a backslash () and allow you to represent characters in strings that would otherwise be difficult to include. -
How do I include a quotation mark in a C++ string?
You can include a quotation mark in a string by using the escape sequence ". -
What does the escape sequence \n do?
The escape sequence \n creates a new line in the output. -
Can I use escape characters in multi-line strings?
Yes, you can use escape characters like \n to create multi-line strings by concatenating them in your output statements. -
What is the purpose of the escape sequence \?
The escape sequence \ is used to include a backslash character in a string.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn