How to Create a Dictionary in C++
- Create a Dictionary in C++ Using the Initializer List Constructor
- Create a Dictionary in C++ Using the Default Constructor
- Create a Dictionary in C++ Using the Copy Constructor
- Create a Dictionary in C++ Using Range-Based Constructor
-
Create a Dictionary in C++ Using
std::unordered_map
- Conclusion
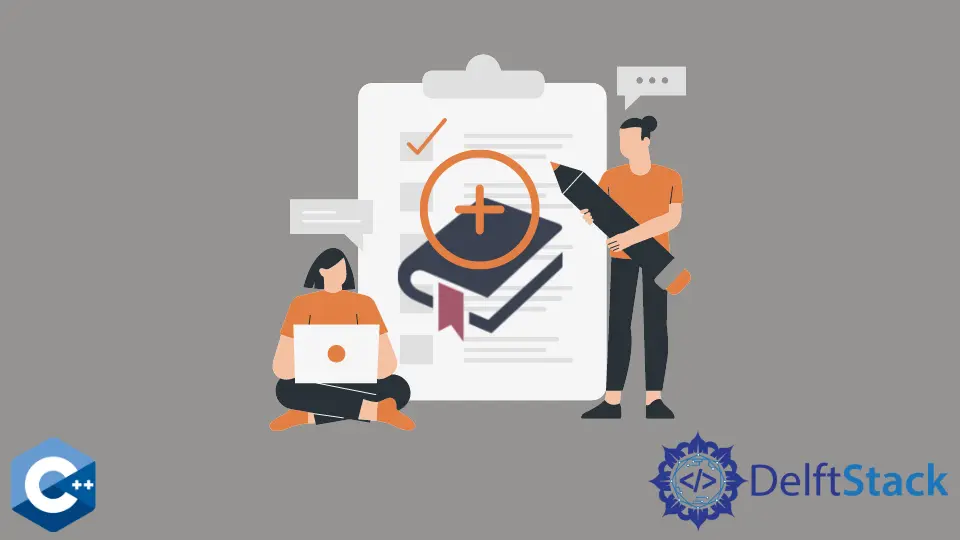
A dictionary, also known as a map or associative array, is a powerful data structure in programming that allows you to store and retrieve data in key-value pairs. In C++, dictionaries are implemented using the std::map
or std::unordered_map
containers from the Standard Template Library (STL).
In this article, we will explore how to create a dictionary in C++ using these containers.
Create a Dictionary in C++ Using the Initializer List Constructor
In C++, one of the ways to implement a dictionary is by using the std::map
container from the Standard Template Library (STL).
The initializer list constructor is a convenient feature introduced in C++11 that allows you to initialize the elements of a container using a list of values enclosed in curly braces {}
. For std::map
, this enables you to initialize key-value pairs directly when declaring the map.
The syntax is as follows:
std::map<KeyType, ValueType> myDictionary = {
{key1, value1}, {key2, value2},
// ... additional key-value pairs
};
Here, KeyType
represents the type of keys, and ValueType
represents the type of values stored in the dictionary.
Let’s take a look at an example demonstrating the creation of a dictionary using the initializer list constructor:
#include <iostream>
#include <map>
template <typename Map>
void PrintMap(Map& m) {
std::cout << "[ ";
for (const auto& item : m) {
std::cout << item.first << ":" << item.second << " ";
}
std::cout << "]\n";
}
int main() {
// Creating a dictionary using the initializer list constructor
std::map<int, std::string> myDictionary = {
{1, "Apple"}, {2, "Banana"}, {3, "Mango"},
{4, "Raspberry"}, {5, "Blackberry"}, {6, "Cocoa"}};
// Printing the dictionary
std::cout << "myDictionary - ";
PrintMap(myDictionary);
return 0;
}
In this example, we start by including the necessary headers for input/output operations and the std::map
container. The PrintMap
template function is defined to print the key-value pairs of the dictionary.
In the main
function, a std::map
named myDictionary
is created using the initializer list constructor. The key-value pairs are enclosed in curly braces, with each pair separated by a comma. The keys are integers (int
), and the values are strings (std::string
).
The PrintMap
function is then called to display the contents of the created dictionary.
Code Output:
This output demonstrates the successful creation and printing of a dictionary using the initializer list constructor. Each key-value pair is displayed within the specified map, providing a concise and efficient way to initialize a dictionary in C++.
Create a Dictionary in C++ Using the Default Constructor
While the initializer list constructor is a powerful tool for initializing dictionaries in C++, there are scenarios where using the default constructor is more suitable.
The default constructor is a special member function of a class or container that is automatically called when an object is created. In the case of the C++ Standard Template Library (STL) containers, including std::map
, the default constructor initializes an empty container.
The syntax for creating a dictionary using the default constructor involves declaring an empty std::map
object and then adding key-value pairs individually. The keys and values are assigned using the subscript ([]
) operator.
The syntax is as follows:
std::map<KeyType, ValueType> myDictionary;
myDictionary[key1] = value1;
myDictionary[key2] = value2;
// ... additional key-value pairs
Here, KeyType
represents the type of keys, and ValueType
represents the type of values stored in the dictionary.
Now, let’s delve into a practical example demonstrating the creation of a dictionary using the default constructor and subsequent addition of key-value pairs:
#include <iostream>
#include <map>
template <typename Map>
void PrintMap(Map& m) {
std::cout << "[ ";
for (const auto& item : m) {
std::cout << item.first << ":" << item.second << " ";
}
std::cout << "]\n";
}
int main() {
// Creating a dictionary using the default constructor
std::map<int, std::string> myDictionary;
// Adding key-value pairs individually
myDictionary[1] = "Banana";
myDictionary[2] = "Mango";
myDictionary[3] = "Cocoa";
myDictionary[4] = "Raspberry";
// Printing the dictionary
std::cout << "myDictionary - ";
PrintMap(myDictionary);
return 0;
}
In this example, we start by including the necessary headers for input/output operations and the std::map
container. The PrintMap
template function is defined to print the key-value pairs of the dictionary.
In the main
function, a std::map
named myDictionary
is declared using the default constructor, creating an initially empty dictionary. Subsequently, key-value pairs are added individually using the subscript ([]
) operator.
The PrintMap
function is then called to display the contents of the created dictionary.
Code Output:
This output confirms the successful creation of a dictionary using the default constructor and the addition of key-value pairs. The dictionary is printed, displaying the specified key-value pairs in the specified map, showcasing the flexibility of this approach in C++.
Create a Dictionary in C++ Using the Copy Constructor
The std::map
container offers a convenient way to create dictionaries, and one method involves using the copy constructor. Using the copy constructor is beneficial when you want to duplicate the contents of a dictionary to another instance.
The copy constructor is automatically called when a new object is created as a copy of an existing object. For C++ Standard Template Library (STL) containers, including std::map
, this constructor facilitates the creation of a new dictionary with the same key-value pairs as an existing one.
The syntax for creating a dictionary using the copy constructor involves declaring a new std::map
object and providing an existing std::map
as an argument. The key-value pairs from the existing map are duplicated to initialize the new map.
The syntax is as follows:
std::map<KeyType, ValueType> newDictionary(existingDictionary);
Here, KeyType
represents the type of keys, and ValueType
represents the type of values stored in the dictionary.
Below is an example demonstrating the creation of a dictionary using the copy constructor:
#include <iostream>
#include <map>
template <typename Map>
void PrintMap(Map& m) {
std::cout << "[ ";
for (const auto& item : m) {
std::cout << item.first << ":" << item.second << " ";
}
std::cout << "]\n";
}
int main() {
// Creating the original dictionary
std::map<int, std::string> originalDictionary = {
{1, "Apple"}, {2, "Banana"}, {3, "Mango"},
{4, "Raspberry"}, {5, "Blackberry"}, {6, "Cocoa"}};
// Creating a new dictionary using the copy constructor
std::map<int, std::string> newDictionary(originalDictionary);
// Printing the new dictionary
std::cout << "newDictionary - ";
PrintMap(newDictionary);
return 0;
}
In this example, we begin by including the necessary headers for input/output operations and the std::map
container. The PrintMap
template function is defined to print the key-value pairs of the dictionary.
In the main
function, an original std::map
named originalDictionary
is created using the initializer list constructor. Subsequently, a new std::map
named newDictionary
is created using the copy constructor, taking the original map as an argument.
The PrintMap
function is then called to display the contents of the newly created dictionary.
Code Output:
This output confirms the successful creation of a new dictionary using the copy constructor. The key-value pairs from the original dictionary are duplicated, resulting in a new map with identical contents. The displayed dictionary illustrates the efficiency and simplicity of this method in C++.
Create a Dictionary in C++ Using Range-Based Constructor
In C++, the range-based constructor is a feature that allows you to create a dictionary and initialize it with elements from a specified range, such as a pair of iterators. This constructor is particularly useful when you want to populate a dictionary using data from another container or a specific range of values.
The range-based constructor is available in C++11 and later versions. It allows you to initialize a container, such as std::map
, with elements from a specified range. For dictionaries, this range often consists of key-value pairs, making it convenient for bulk initialization.
The syntax for creating a dictionary using the range-based constructor involves declaring a new std::map
object and specifying a range from an existing std::map
. The range is defined using the begin()
and end()
iterators of the existing map.
The syntax is as follows:
std::map<KeyType, ValueType> newDictionary(existingMap.begin(),
existingMap.end());
Here, KeyType
represents the type of keys, and ValueType
represents the type of values stored in the dictionary.
Let’s delve into a practical example demonstrating the creation of a dictionary using the range-based constructor:
#include <iostream>
#include <map>
template <typename Map>
void PrintMap(Map& m) {
std::cout << "[ ";
for (const auto& item : m) {
std::cout << item.first << ":" << item.second << " ";
}
std::cout << "]\n";
}
int main() {
// Creating the original dictionary
std::map<int, std::string> originalDictionary = {
{1, "Apple"}, {2, "Banana"}, {3, "Mango"},
{4, "Raspberry"}, {5, "Blackberry"}, {6, "Cocoa"}};
// Creating a new dictionary using the range-based constructor
std::map<int, std::string> newDictionary(originalDictionary.find(2),
originalDictionary.end());
// Printing the new dictionary
std::cout << "newDictionary - ";
PrintMap(newDictionary);
return 0;
}
In this example, we include the necessary headers for input/output operations and the std::map
container. The PrintMap
template function is defined to print the key-value pairs of the dictionary.
In the main
function, an original std::map
named originalDictionary
is created using the initializer list constructor. Subsequently, a new std::map
named newDictionary
is created using the range-based constructor. The range is specified from the key-value pair with the key 2
to the end of the original map.
The PrintMap
function is then called to display the contents of the newly created dictionary.
Code Output:
This output confirms the successful creation of a new dictionary using the range-based constructor. The specified range starting from the key 2
to the end of the original dictionary is captured, resulting in a new map with the desired key-value pairs.
The displayed dictionary showcases the effectiveness of this method in C++.
Create a Dictionary in C++ Using std::unordered_map
Another powerful container for creating dictionaries is std::unordered_map
. This container provides faster average access times for individual elements compared to std::map
but does not guarantee any specific order of elements.
The syntax for creating a dictionary using std::unordered_map
is similar to std::map
. The key difference lies in the container type declaration and initialization.
The syntax is as follows:
std::unordered_map<KeyType, ValueType> myDictionary = {
{key1, value1}, {key2, value2},
// ... additional key-value pairs
};
Here, KeyType
represents the type of keys, and ValueType
represents the type of values stored in the dictionary.
Let’s delve into a practical example demonstrating the creation of a dictionary using std::unordered_map
:
#include <iostream>
#include <unordered_map>
template <typename Map>
void PrintMap(Map& m) {
std::cout << "[ ";
for (const auto& item : m) {
std::cout << item.first << ":" << item.second << " ";
}
std::cout << "]\n";
}
int main() {
// Creating an unordered dictionary using std::unordered_map
std::unordered_map<int, std::string> myDictionary = {
{1, "Apple"}, {2, "Banana"}, {3, "Mango"},
{4, "Raspberry"}, {5, "Blackberry"}, {6, "Cocoa"}};
std::cout << "myDictionary - ";
PrintMap(myDictionary);
return 0;
}
In this example, we include the necessary headers for input/output operations and the std::unordered_map
container. The PrintMap
template function is defined to print the key-value pairs of the dictionary.
In the main
function, an unordered dictionary named myDictionary
is created using std::unordered_map
with the initializer list constructor. The key-value pairs are specified within curly braces.
The PrintMap
function is then called to display the contents of the unordered dictionary.
Code Output:
This output confirms the successful creation of an unordered dictionary using std::unordered_map
.
The key advantage of using std::unordered_map
is the constant time complexity for average-case operations. This is achieved by utilizing hash functions, making lookups, insertions, and deletions more efficient compared to std::map
.
However, it’s essential to note that the order of elements in a std::unordered_map
is not guaranteed.
Conclusion
In conclusion, creating a dictionary in C++ can be accomplished through various methods, each offering distinct advantages based on specific requirements.
The use of the std::map
container, whether through the initializer list constructor, default constructor, copy constructor, or range-based constructor, provides a flexible and ordered structure for key-value pairs. Alternatively, the std::unordered_map
container offers faster access times, albeit without maintaining a specific order.
Choosing the most suitable method depends on the nature of the data and the desired trade-offs between performance and order. C++ provides developers with versatile tools to efficiently manage and manipulate dictionaries, catering to a diverse range of programming needs.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook