Difference Between Const Int and Int Const in C++
-
Difference Between
const int
andint const
in C++ -
C++
const
Keyword -
const int
andint const
With Variables -
const int
andint const
With Pointers -
Use
int * const
in C++ -
Use
const int *
in C++ - Conclusion
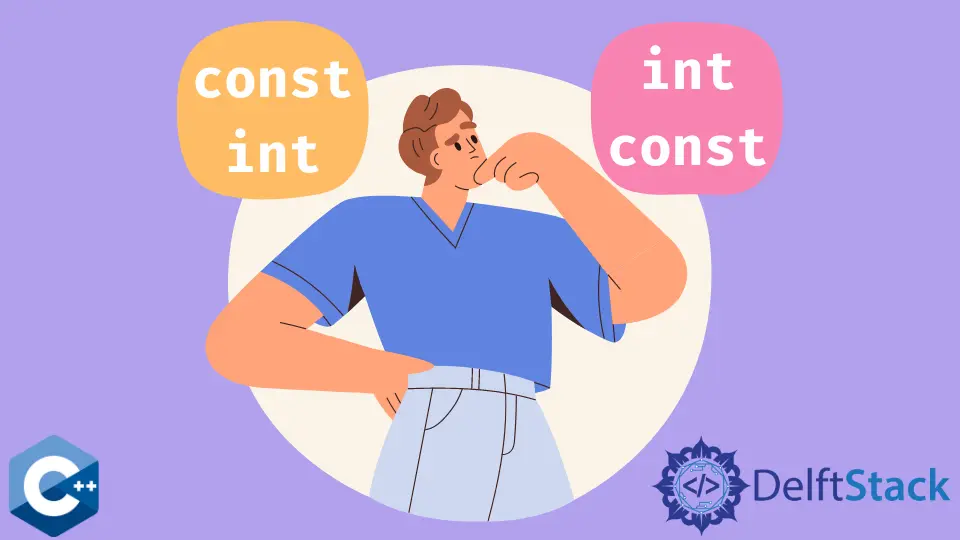
The most loved and tricky questions of any programming language are the ones where little changes make a lot of difference in how the program will run and the output it will give.
In this article, we’ll discuss whether const int
is the same as int const
in C++ or not.
Difference Between const int
and int const
in C++
Code:
int const a = 1000;
const int a = 1000;
Here are two lines of code that look almost the same. Now, are these two lines of code the same?
Before we answer that question, let’s revisit the basics of the const
keyword of C++.
C++ const
Keyword
The const
keyword of C++ helps keep certain things constant. This means that if you put the const
keyword before any variable, pointer, or method, then the value of the data items of these variables, pointers, and methods can’t be changed while the program is under execution.
Suppose we try to change the value of any variable, pointer, or method that is initialized with a const
keyword, which gives us an error.
Code:
#include <iostream>
using namespace std;
int main() {
const int demo = 1; // constant variable
cout << demo;
demo = 2 // changing the value of constant variable
cout
<< demo;
return 0;
}
Output:
In function 'int main()': error: assignment of read-only variable 'demo'
9 | demo = 2
| ~~~~~^~~
In the above example, we declare a const
variable demo
and assign the value 1
to it. Then, we assign a different value, 2
, to the same constant variable.
This results in an error, as you can see in the output.
Code:
#include <iostream>
using namespace std;
int main() {
int demo = 1;
cout << "The initial value is: " << demo << endl;
// changing the value of the variable
demo = 2;
cout << "The changed value is: " << demo << endl;
return 0;
}
Output:
The initial value is: 1
The changed value is: 2
Now this time, we do not initialize the variable demo
with the keyword const
. Therefore, we can change the value of this variable without getting any errors.
This is essentially how the const
keyword works. Refer to this link to know more about the const
keyword.
Let’s go back to the question we had to answer.
const int
and int const
With Variables
The standard way to attach const
to a variable in C++ is to put this keyword before the variable’s data type. However, if we place it before the variable itself, it serves the same purpose and works similarly.
This means that the following lines of code are equivalent and correct.
const int demo = 1000;
int const demo = 1000;
When you read such declarations, the trick is to go from right to left. Therefore, the first line of code would be read as - demo is a constant integer.
While the second line is read as - demo is a constant integer. Doubtless, to say, both these statements mean the same.
We can verify this through a working example. In this following example, we place the keyword const
before the data type, i.e. int
and then print the variable demo
.
Code:
#include <iostream>
using namespace std;
int main() {
const int demo = 1000; // const keyword before the data type
cout << demo;
}
Output:
1000
Let’s try the same with the keyword const
placed before the variable, demo
itself.
#include <iostream>
using namespace std;
int main() {
int const demo = 1000; // const keyword before the variable itself
cout << demo;
}
Output:
1000
See how these statements work the same way without resulting in any kind of error. We can use either of these statements when we have to declare a constant variable.
Thus, we can conclude that int const
is the same as const int
, but there is a catch. This is true for variables until pointers don’t come in.
For pointers, the meaning of the keyword const
changes as per its position. Let us discuss how the keyword const
works with pointers.
const int
and int const
With Pointers
Using the const
keyword with pointers is very simple. Either we can make the pointer immutable or the content pointing to immutable.
Look at the below line of code. The pointer demo
is a constant pointer whose value can’t be changed.
This means that it cannot be modified to point to a different value or variable. We read this from right to left as - demo is a constant pointer to an integer.
int* const demo = &anyvalue;
However, in this second piece of code given below, the data that the pointer demo
points to can’t be changed. We read this as - demo is a pointer to an integer that is constant.
const int *demo = &anyvalue;
Let us understand both these lines of code with examples.
Use int * const
in C++
As mentioned above, placing the keyword const
before the pointer variable means we can not change the pointer to point to a different value or variable. This can be seen more profoundly through an example.
The following points summarize what’s happening in the code given below.
- Here, we first assign the value of the variable
one
to the pointer variable*demo
. This means that the pointer*demo
points to the variableone
. - On printing the value of variable
one
, we get the output as1
, while on printingdemo
, we get the address it is pointing to. This is the address of variableone
. - Later, we assign another variable,
two
, to the same pointer variable,demo
and print the values. This time the pointer returns a different address.
Code:
#include <iostream>
using namespace std;
int main() {
int one = 1;
int two = 2;
int *demo = &one; // demo pointer points to variable one
cout << one << endl;
cout << demo << endl;
demo = &two; // assigning the variable two to demo variable
cout << two << endl;
cout << demo << endl;
return 0;
}
Output:
1
0x7ffc22e802a8
2
0x7ffc22e802ac
You can see how we can assign different variables to the same pointer variable. This change is impossible if you attach the const
keyword before the pointer variable.
Code:
#include <iostream>
using namespace std;
int main() {
int one = 1;
int two = 2;
int* const demo = &one; // attach const keyword before the pointer variable
cout << one << endl;
cout << demo << endl;
demo = &two; // this assignment will now give an error
cout << two << endl;
cout << demo << endl;
return 0;
}
Output:
In function 'int main()': error: assignment of read-only variable 'demo'
13 | demo = &two;
| ~~~~~^~~~~~
Note that this time, since the declared pointer is constant, we cannot assign a different variable to it. Thus we get an error.
This is what int const
means when used with a pointer.
Use const int *
in C++
We already know that we can use a pointer to change the value of a variable. This is shown in the example below.
The following points summarize what’s happening in this code.
- We first assign the value,
1
to the variableone
, and the pointer*demo
points to the variableone
. - Then, we print the values of the variable
one
and the pointer*demo
. Both these give the output as1
. - Later, we assign the value
2
to the pointer variable,*demo
. - Lastly, we print the values of the variable
one
and the pointerdemo
, but this time they both give the output as2
.
Code:
#include <iostream>
using namespace std;
int main() {
int one = 1; // variable one holds the value 1
int *demo = &one;
cout << one << endl;
cout << *demo << endl;
*demo = 2; // assign the value 2 to variable demo
cout << one << endl;
cout << *demo << endl;
}
Output:
1
1
2
2
See how the value of the variable changes with the help of the pointer to which the variable was assigned. Now, if you use the const
keyword before the data type of the pointer, this kind of mutability won’t be possible.
Code:
#include <iostream>
using namespace std;
int main() {
int one = 1;
const int *demo = &one; // attach const keyword before data type
cout << one << endl;
cout << *demo << endl;
*demo = 2; // this assignment will give an error now
cout << one << endl;
cout << *demo << endl;
}
Output:
In function 'int main()': error: assignment of read-only location '* demo'
12 | *demo = 2;
| ~~~~~~^~~
Note that we get an error if we try changing the value of the variable that the pointer points to. This is what const int
means when used with a pointer.
Conclusion
In this article, we learned about how const int
and int const
are different from each other. Moreover, we saw how they work in the context of variables and pointers.
We also learned that both these formats mean the same for simple variables but pointers meaning changes significantly. We understood this through various working examples and also went over the basics of the const
keyword of C++.