Sign Extend a 9-Bit Integer in C
- What is Sign Extend
- Sign Extend a 9-Bit Number in C
-
Method 1: Arithmetic Right Shift and Bitwise
OR
- Method 2: Left Shift and Arithmetic Right Shift
- Method 3: Bitwise Operations
- Method 4: Conditional Operations
- Method 5: Bitwise Manipulation with Shifts
- Conclusion
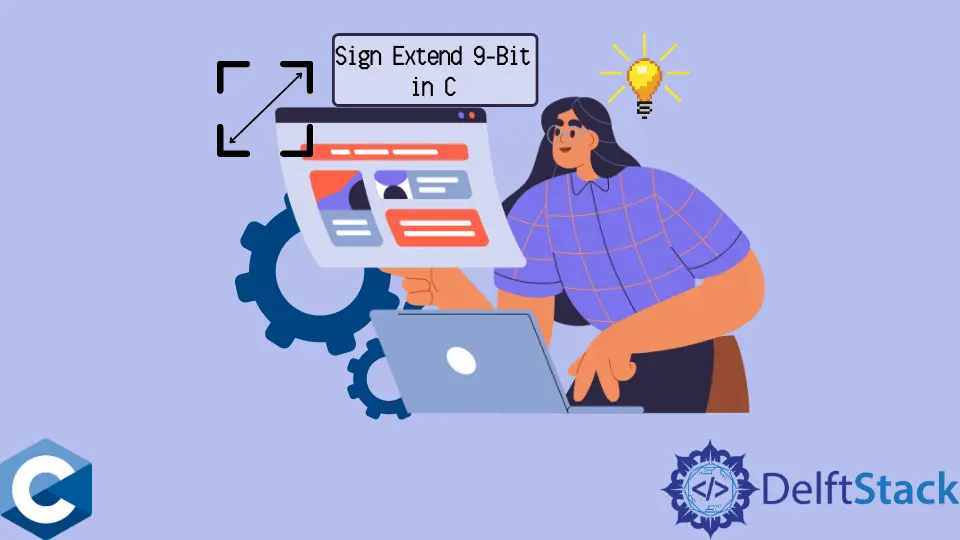
When working with integers in programming, it’s essential to maintain their signedness when extending their bit width. Sign extension ensures that the sign of the number remains consistent when increasing its bit size.
In the context of a nine-bit integer in C, sign extension involves expanding it to a larger bit width while preserving its signed representation.
In this article, we’ll explore several methods to perform sign extension on a nine-bit number in C. Each method aims to preserve the sign bit (the most significant bit) while extending the integer to a larger size.
By employing bitwise operations, logical manipulations, and arithmetic shifts, these methods provide diverse approaches to achieve sign extension efficiently.
What is Sign Extend
Sign extension is a process used in computer science and digital arithmetic to expand the bit width of a binary number while preserving its signedness. It involves replicating the most significant bit (often the sign bit) of the original number across the additional bits to maintain the number’s signed value.
For instance, if you have a signed binary number like "1101"
representing -3
in a four-bit system (where the leftmost bit indicates the sign), and you want to extend it to an eight-bit system, sign extension would replicate the leftmost bit (1) to fill the additional bits, resulting in "11111101"
in an eight-bit representation.
In essence, sign extension ensures that the original signed value of the number remains consistent when expanded to a larger bit width. This process is essential for maintaining the integrity of signed numbers during arithmetic operations, memory storage, and data manipulation within computer systems.
Sign Extend a 9-Bit Number in C
Some bitwise operators in the C programming language operate at the hardware level. However, to utilize these operators, it is necessary to understand the architecture of the memory and how C saves data in the memory.
Structure:
- The memory is divided into 1 byte for each character of the
char
type. - The memory structure of an
int
type consists of 4 bytes. - A
short
type memory location consists of 2 bytes.
The information regarding the sign can be found in the portion farthest to the left. The number 1 indicates a negative number, whereas the number 0 represents a positive number.
Let’s look at the following example to better comprehend what is being discussed.
To begin, in the main()
class, we will create a variable of the short
type and give it the name val
. We will then assign the variable the same way as below.
short val = 234;
After that, we will provide the short
value we made together with a 9-bit number to the variable called res
.
int res = val & 0b0000000111111111;
An if
check is applied. This checks if the sign bit, which is the highest bit that you are keeping (0x100)
, is set, and if it is, it puts all the bits that come after it.
if (val & 0x100) {
res = res | 0xFE00;
}
We made a val
variable, a small integer with 16 bits in the memory. The &
operator was then used to mask the most recent 9 bits.
It is the AND
operator used in bitwise operations, and its process is as described below.
X Y res
0 0 0
0 1 0
1 0 0
1 1 1
-
The
res
represents the result, whileX
andY
represent the many input options. The calculation is done bit by bit.It will only produce a 1 if both bits with the same significant value are 1.
-
Therefore, we may separate the value’s final 9 bits of the bitwise
AND
operator, and the remainder of the bits will be set to 0. The value0b0000000111111111
corresponds to the binary representation.This demonstrates that just the most recent 9 bits contain 1, while the remainder contains a 0. The
res
variable will be updated with the result. -
After that, we used an
if
check to verify the first bit. If it was 1, we added it to the result. Otherwise, we left it at 0.
For that purpose, we again used the bitwise AND
operator, and, this time, we extracted the first bit only. If the answer was 1, then we used the |
operator, which is the bitwise OR
operator and works as follows.
X Y res
0 0 0
0 1 1
1 0 1
1 1 1
-
The
res
is the output, andX
andY
are the input possibilities. It is calculated bit by bit.It only outputs 0 if both the same significant bit is 0. In the
if
statement, the result of this operator is assessed.If
true
, the bitwiseOR
operator is used to insert 1 in the result’s leftmost bit. -
0xFE00
is the hexadecimal representation of the binary number, and it is used to set the bits above it if there is a sign bit present.
By utilizing the bitwise operator in C, we can perform a variety of other things. There are many more bitwise operators in C than bitwise AND
and bitwise OR
.
There is bitwise XOR
represented as a ^
symbol. The ~
symbol represents a bitwise NOT
operator.
The >>
sign denotes a left-shift operator. You may do the right shift using the <<
symbol.
You may do this or any other desired job in a variety of ways with the assistance of these operators.
Complete Source Code:
#include <stdio.h>
int main() {
short val = 234;
int res = val & 0b0000000111111111;
if (val & 0x100) {
res = res | 0xFE00;
}
return 0;
}
This code snippet takes a 9-bit value stored in a short
variable (val
), extracts these 9 bits into an int
variable (res
), and then checks the sign bit to perform sign extension by replicating the sign bit across the higher bits of res
.
Method 1: Arithmetic Right Shift and Bitwise OR
This method involves using an arithmetic right shift and a bitwise OR
operation.
int sign_extend_arithmetic(int x) {
const int sign_bit = (x & 0x100) != 0; // Extract sign bit (bit 8)
return (x & 0xFF) | (sign_bit ? 0xFF00 : 0); // Sign extension
}
The code defines a function, sign_extend_arithmetic
, that performs sign extension on a nine-bit integer x
. It isolates the sign bit, determining whether the number is positive or negative, and extends the nine-bit value to a larger size while preserving the sign.
Combining the original nine bits with additional bits based on the sign bit ensures the proper representation of the signed integer in the extended format.
Method 2: Left Shift and Arithmetic Right Shift
This method involves left-shifting the nine-bit integer to a larger size and then performing an arithmetic right shift.
int sign_extend_shift(int x) {
return (x << 23) >> 23; // Shift left to 32-bit, then right to sign extend
}
The code defines a function sign_extend_shift
that extends a nine-bit integer (x
) to a 32-bit signed integer while preserving its sign. It accomplishes this by first left-shifting x
to occupy the lower bits of a 32-bit integer.
Then, it performs an arithmetic right shift to propagate the sign bit (bit 8 in the nine-bit integer) to fill the higher bits, resulting in the sign-extended 32-bit representation of the original nine-bit value.
Method 3: Bitwise Operations
This method directly manipulates the bits to perform sign extension.
int sign_extend_bitwise(int x) {
return (x ^ 0x100) - 0x100; // Toggle sign bit, subtract to extend sign
}
The code defines a function sign_extend_bitwise
that extends a 9-bit integer x
to a larger size while preserving its sign. It achieves this by flipping the sign bit using bitwise XOR
(x ^ 0x100
) and then adjusting the value by subtracting 256
(0x100
in decimal).
This process maintains the signed representation of the integer during the extension, effectively concisely performing sign extension using bitwise operations.
Method 4: Conditional Operations
Using conditional operations to extend the nine-bit integer to a larger size while considering the sign bit.
int sign_extend_conditional(int x) {
return (x & 0x100) ? (x | 0xFF00)
: x; // If sign bit set, OR with sign extension
}
The sign_extend_conditional
function performs sign extension on a 9-bit integer x
. It checks if the 9th bit (sign bit) is set; if true (indicating a negative number), it extends the sign by setting higher bits using a bitwise OR
operation with 0xFF00
.
If the sign bit is not set (indicating a positive number), it returns the original value of x
unchanged. This concise function effectively extends the sign of a 9-bit integer when needed, ensuring consistent handling of signed values.
Method 5: Bitwise Manipulation with Shifts
Another approach uses bitwise operations and shifts to sign extend a nine-bit integer.
int sign_extend_shifts(int x) {
return ((x & 0x100) ? (x | 0xFE00)
: (x & 0x1FF)); // Sign extension using shifts
}
The code defines a function, sign_extend_shifts
, handling sign extension for a nine-bit integer x
. It checks if the 9th bit (sign bit) is set by using bitwise operations.
If the sign bit is 1 (indicating a negative number), the function extends the sign by setting the higher bits to 1 through a bitwise OR
operation with 0xFE00
.
For positive numbers (when the sign bit is 0), it returns x
with only the original nine bits preserved using a bitwise AND
operation with 0x1FF
. This function efficiently performs sign extension on a nine-bit integer using bitwise operations and conditional checks.
Conclusion
This article delves into the importance of maintaining the signedness of integers when extending their bit width, focusing on sign extension techniques for a nine-bit integer in C. It explores various methods utilizing bitwise operations, logical manipulations, and shifts to extend the integer while preserving its signed representation.
The examined methods, including arithmetic shifts with bitwise OR
operations, conditional checks, direct bitwise manipulations, and logical operations, showcase diverse strategies for sign extension. Each method aims to retain the significance of the sign bit when expanding the integer to a larger size.
Understanding bitwise operations in C and their impact on memory architecture is crucial for effectively manipulating and extending integer representations. These techniques highlight the versatility of bitwise operations in ensuring accurate representation and interpretation of numerical values, especially in preserving the signedness of integers during bit width extension.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn