The %p Format Specifier in C
- Introduction to Format Specifiers in C
-
Functionality of the
%p
Format Specifier in C -
Use Cases of
%p
in C -
Handling Pointers With
%p
- Conclusion
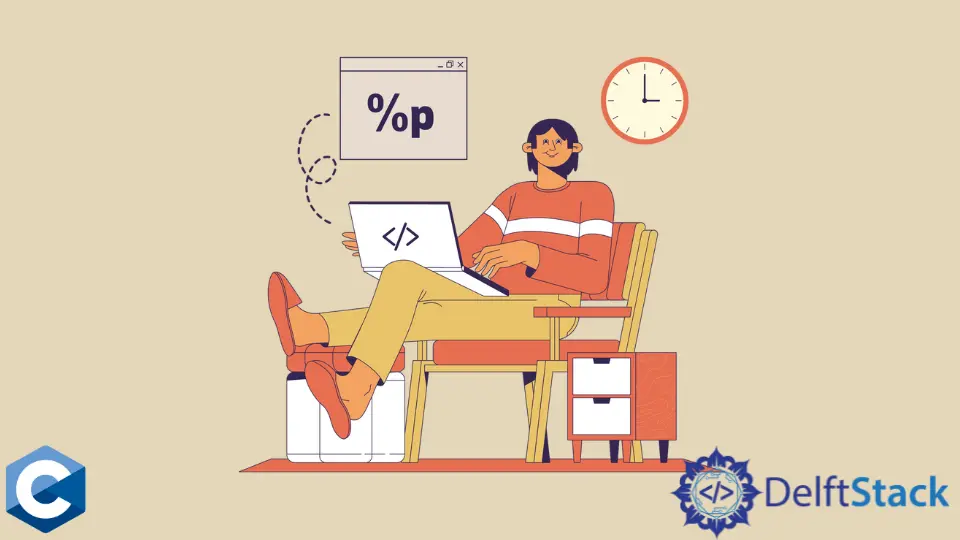
In the world of C programming, format specifiers play a crucial role in controlling the way data is displayed or interpreted when using functions like printf
and scanf
. Among these specifiers, %p
holds a unique place, providing a means to work with pointers.
In this article, we will delve into the intricacies of the %p
format specifier in C, exploring its functionality, use cases, and the nuances of working with pointers.
Introduction to Format Specifiers in C
Format specifiers in C are placeholders used in format strings to specify the type and format of the input or output.
They are primarily associated with functions like printf
and scanf
from the standard input/output library (stdio.h
). The %p
format specifier is specifically designed to handle pointers.
Functionality of the %p
Format Specifier in C
The %p
format specifier in C is used for displaying pointers in a human-readable format. When used with printf
, it takes a pointer as an argument and prints its value as a hexadecimal number.
For example:
#include <stdio.h>
int main() {
int value = 42;
int *ptr = &value;
printf("Address of 'value': %p\n", (void *)ptr);
return 0;
}
Output:
Address of 'value': 0x7ffee706736c
In this example, (void *)ptr
is used to cast the pointer to the void *
type, which is necessary because %p
expects a void *
argument. The output will display the memory address of the value
variable in hexadecimal format.
Use Cases of %p
in C
Let’s explore some practical scenarios where the %p
format specifier proves to be valuable:
1. Debugging and Output Formatting
When developing C programs, especially those dealing with dynamic memory allocation, debugging becomes crucial. The %p
specifier helps developers print the memory addresses of pointers, aiding in identifying potential issues related to memory management.
#include <stdio.h>
#include <stdlib.h>
int main() {
int *dynamicArray = malloc(5 * sizeof(int));
if (dynamicArray != NULL) {
printf("Memory address of dynamicArray: %p\n", (void *)dynamicArray);
free(dynamicArray);
}
return 0;
}
Output:
Memory address of dynamicArray: 0x558b2a83a2a0
2. Pointers in Structures
In data structures where pointers are used as members, %p
can be employed to display the memory addresses contained within those structures.
#include <stdio.h>
struct Node {
int data;
struct Node *next;
};
int main() {
struct Node node;
struct Node *ptr = &node;
printf("Address of 'next' in Node struct: %p\n", (void *)&ptr->next);
return 0;
}
Output:
Address of 'next' in Node struct: 0x7ffd71db5a58
3. Dynamic Memory Allocation
When working with dynamic memory allocation using functions like malloc
or calloc
, %p
helps in printing the addresses returned by these functions.
#include <stdio.h>
#include <stdlib.h>
int main() {
int *dynamicVar = malloc(sizeof(int));
if (dynamicVar != NULL) {
printf("Memory address of dynamicVar: %p\n", (void *)dynamicVar);
free(dynamicVar);
}
return 0;
}
Output:
Memory address of dynamicVar: 0x55bc661662a0
4. Portability and Consistency
The %p
format specifier is designed to ensure portability across different platforms. It abstracts away the details of pointer representation, providing a consistent and platform-independent way to print pointer addresses.
Handling Pointers With %p
While %p
is a powerful tool for working with pointers in C, it comes with certain responsibilities. Here are some considerations when using %p
:
1. Cast to void *
As demonstrated in the examples, it is essential to cast the pointer to void *
when using %p
to print the address. This ensures compatibility with the expected argument type.
2. Output Interpretation
Remember that the output of %p
is in hexadecimal format. Understanding hexadecimal notation is crucial for interpreting and analyzing the output correctly.
3. NULL Pointers
When dealing with potentially null pointers, ensure that proper checks are in place before using %p
to print their addresses. Attempting to print the address of a null pointer can lead to undefined behavior.
Conclusion
The %p
format specifier in C is a valuable tool for working with pointers, offering a concise and standardized way to print memory addresses. Whether debugging dynamic memory allocation, analyzing data structures, or ensuring portability across platforms, %p
provides a versatile solution for displaying pointer addresses in a readable format.
By understanding the functionality and use cases of %p
, C programmers can enhance their ability to work with pointers effectively. However, it is crucial to handle pointers responsibly, considering issues like type casting and null pointer checks to ensure the reliability and correctness of the code.
As C remains a foundational language in system programming and embedded systems, mastering features like %p
contributes to writing robust and efficient code.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn