How to Use the gettimeofday Function in C
-
Calculate Elapsed Time in a Code Block in C Using the
gettimeofday
Function -
Calculate Elapsed Time in a Code Block in C Using the
clock_gettime
Function -
Calculate Elapsed Time in a Code Block in C Using the
clock
Function - Conclusion
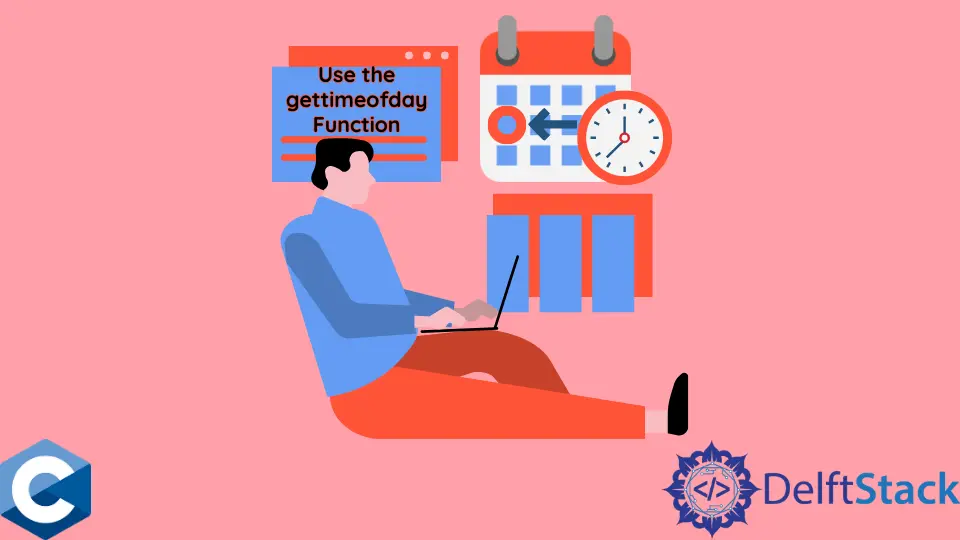
Time plays a significant role in programming, and sometimes, it’s essential to measure the elapsed time between certain operations or events in your code. This can be particularly useful for performance analysis, benchmarking, or simply tracking how long a specific task takes to execute.
In C, you can achieve this by using various functions provided by the standard library. In this article, we’ll explore how to calculate elapsed time in a code block using C.
Calculate Elapsed Time in a Code Block in C Using the gettimeofday
Function
In C programming, the gettimeofday
function is a powerful tool for obtaining accurate time information.
The gettimeofday
function is commonly used in Unix-like operating systems to retrieve the current time with microsecond precision. It is declared in the <sys/time.h>
header file.
Its syntax is as follows:
int gettimeofday(struct timeval *tv, struct timezone *tz);
Here,
struct timeval
: Holds the time information that holds the seconds and microseconds since the Epoch (January 1, 1970).struct timezone
: This is a structure used to represent the local timezone information (which is now obsolete), so you should passNULL
instead.
To calculate elapsed time, you typically follow these steps:
-
Record the current time before the code block starts using
gettimeofday
. -
Execute the code block you want to measure.
-
Record the current time again after the code block finishes.
-
Calculate the time difference between the two recorded times.
To calculate the elapsed time of a code block, we need to record the current time before and after the execution of the block and then find the difference between these two timestamps.
Here’s a simple example:
#include <stdio.h>
#include <stdlib.h>
#include <sys/time.h>
#define NUM 1000000
#define NUM2 10000000
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
void loopFunc(size_t num) {
int tmp = 0;
for (int i = 0; i < num; ++i) {
tmp += 1;
}
}
int main() {
struct timeval start, end;
// Measure time for code block 1
gettimeofday(&start, NULL);
loopFunc(NUM);
gettimeofday(&end, NULL);
printf("loopFunc(%d) time spent: %0.8f sec\n", NUM, time_diff(&start, &end));
// Measure time for code block 2
gettimeofday(&start, NULL);
loopFunc(NUM2);
gettimeofday(&end, NULL);
printf("loopFunc(%d) time spent: %0.8f sec\n", NUM2, time_diff(&start, &end));
exit(EXIT_SUCCESS);
}
The time_diff
function takes two struct timeval
pointers, representing the start and end times, and calculates the time difference in seconds.
The loopFunc
is a simple function used for demonstration purposes, and you can replace it with the code block you want to measure.
In the main
function, we declare two struct timeval
variables, start
and end
, to store the time before and after each code block execution. The gettimeofday
function is used to record the current time.
After executing the code block (loopFunc
in this case), we record the end time again. The time_diff
function calculates the elapsed time, and the result is printed to the console.
Output:
loopFunc(1000000) time spent: 0.00221000 sec
loopFunc(10000000) time spent: 0.02184500 sec
The output displays the elapsed time for executing the specified code blocks, providing valuable insights into the performance characteristics of your C program.
Calculate Elapsed Time in a Code Block in C Using the clock_gettime
Function
In addition to the gettimeofday
function, another powerful tool for measuring elapsed time in C programming is the clock_gettime
function. This function provides a higher resolution and more consistent interface for obtaining time information.
The clock_gettime
function is part of the <time.h>
header in C, and it allows you to retrieve the current time with nanosecond precision. Its syntax is as follows:
int clock_gettime(clockid_t clk_id, struct timespec *tp);
Here,
clockid_t
: Specifies the clock to be used, withCLOCK_REALTIME
being a common choice for wall-clock time.struct timespec
: Holds the time information, including seconds and nanoseconds.
Similar to the approach with gettimeofday
, to measure elapsed time using clock_gettime
, you typically:
-
Record the current time before the code block starts.
-
Execute the code block.
-
Record the current time again after the code block finishes.
-
Calculate the time difference between the two recorded times.
Now, let’s explore an example to illustrate these concepts.
#include <stdio.h>
#include <stdlib.h>
#include <sys/time.h>
#include <time.h>
#define NUM 1000000
#define NUM2 10000000
float time_diff2(struct timespec *start, struct timespec *end) {
return (end->tv_sec - start->tv_sec) + 1e-9 * (end->tv_nsec - start->tv_nsec);
}
void loopFunc(size_t num) {
int tmp = 0;
for (int i = 0; i < num; ++i) {
tmp += 1;
}
}
int main() {
struct timespec start2, end2;
// Measure time for code block 1
clock_gettime(CLOCK_REALTIME, &start2);
loopFunc(NUM);
clock_gettime(CLOCK_REALTIME, &end2);
printf("loopFunc(%d) time spent: %0.8f sec\n", NUM,
time_diff2(&start2, &end2));
// Measure time for code block 2
clock_gettime(CLOCK_REALTIME, &start2);
loopFunc(NUM2);
clock_gettime(CLOCK_REALTIME, &end2);
printf("loopFunc(%d) time spent: %0.8f sec\n", NUM2,
time_diff2(&start2, &end2));
exit(EXIT_SUCCESS);
}
The time_diff2
function calculates the time difference between two struct timespec
pointers, representing the start and end times in seconds.
The loopFunc
is a simple function for demonstration purposes. Replace it with the code block you want to measure.
In the main
function, we declare two struct timespec
variables, start2
and end2
, to store the time before and after each code block execution. The clock_gettime
function is used to record the current time.
After executing the code block, we record the end time again. The time_diff2
function calculates the elapsed time, and the result is printed to the console.
Output:
loopFunc(1000000) time spent: 0.00221000 sec
loopFunc(10000000) time spent: 0.02184500 sec
The output provides the elapsed time for executing the specified code blocks, aiding in the assessment of your C program’s performance characteristics.
Calculate Elapsed Time in a Code Block in C Using the clock
Function
In C programming, the <time.h>
header provides the clock
function, which is a straightforward way to measure elapsed time within a code block. Unlike gettimeofday
and clock_gettime
, clock
returns the processor time used by the program, which can be useful for understanding the CPU time consumed.
The clock
function in C returns the processor time used by the program since the start of its execution. The returned value is in clock ticks, and to convert it to seconds, you can use the CLOCKS_PER_SEC
constant.
The clock
function’s syntax is straightforward:
clock_t clock(void);
It returns the number of clock ticks (an implementation-dependent unit representing processor time) since the program’s inception.
To calculate elapsed time using clock
, the general steps are as follows:
-
Record the current clock time before the code block starts.
-
Execute the code block.
-
Record the current clock time again after the code block finishes.
-
Calculate the time difference between the two recorded clock times.
Now, let’s see an example to illustrate these principles.
#include <stdio.h>
#include <time.h>
#define NUM 1000000
#define NUM2 10000000
void loopFunc(size_t num) {
int tmp = 0;
for (int i = 0; i < num; ++i) {
tmp += 1;
}
}
int main() {
clock_t start_time, end_time;
// Measure time for code block 1
start_time = clock();
loopFunc(NUM);
end_time = clock();
double elapsed_time1 = ((double)(end_time - start_time)) / CLOCKS_PER_SEC;
printf("loopFunc(%d) time spent: %0.8f sec\n", NUM, elapsed_time1);
// Measure time for code block 2
start_time = clock();
loopFunc(NUM2);
end_time = clock();
double elapsed_time2 = ((double)(end_time - start_time)) / CLOCKS_PER_SEC;
printf("loopFunc(%d) time spent: %0.8f sec\n", NUM2, elapsed_time2);
return 0;
}
The loopFunc
is a simple function used for demonstration purposes. Replace it with the code block you want to measure.
In the main
function, we declare the start_time
and end_time
variables of type clock_t
to store the clock time before and after each code block execution. The clock
function is used to record the current clock time.
After executing the code block, we record the end time again. The elapsed time is calculated by subtracting the start time from the end time and converting it to seconds using CLOCKS_PER_SEC
.
The result is printed to the console.
Output:
loopFunc(1000000) time spent: 0.01000000 sec
loopFunc(10000000) time spent: 0.11000000 sec
The output presents the elapsed time for executing the specified code blocks, offering valuable insights into the CPU time consumption of your C program.
Conclusion
Measuring elapsed time within a code block in C is a significant aspect of performance optimization and profiling.
In this article, we explored three distinct methods for achieving this: utilizing the gettimeofday
function, the clock_gettime
function, and the clock
function. Each method provides a unique approach to time measurement, offering varying levels of precision and suitability for different use cases.
The gettimeofday
function, with its microsecond precision, is widely supported and suitable for general-purpose timing.
On the other hand, the clock_gettime
function offers high-resolution timing information and is particularly valuable for POSIX-compliant systems. Lastly, the clock
function provides a straightforward way to measure CPU time consumption.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook