How to Use the getaddrinfo Function in C
-
Use the
getaddrinfo
Function to Host Name to IP Address in C -
Use the
getnameinfo
Function to IP Address to Host Name in C
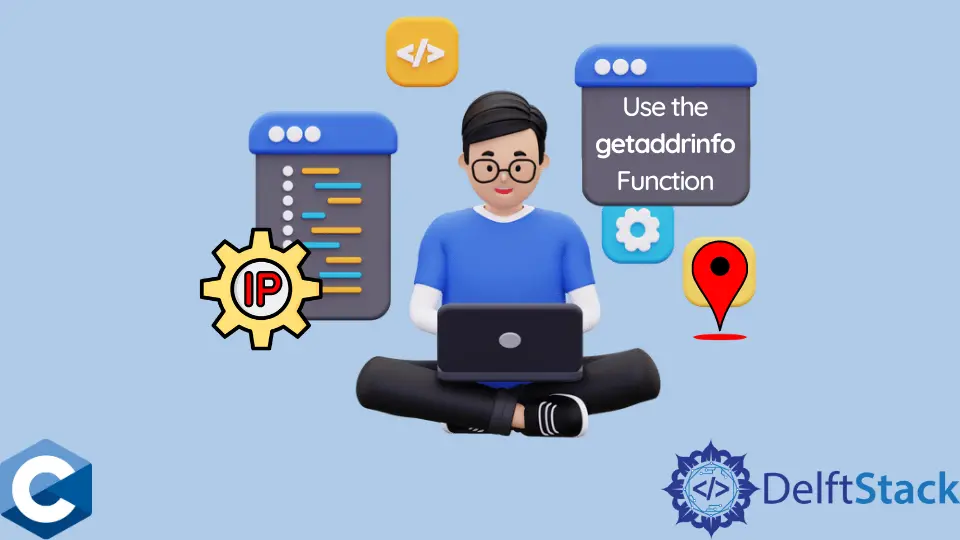
This article will demonstrate multiple methods about how to use the getaddrinfo
function in C.
Use the getaddrinfo
Function to Host Name to IP Address in C
getaddrinfo
is part of the UNIX networking programming facilities, and it can convert network host information to the IP address and conversely. getaddrinfo
is also a POSIX compliant function call, and it can do conversion regardless of the underlying protocols.
getaddrinfo
takes four arguments,
- The first can be the pointer to either the hostname or IPv4/IPv6 formatted address string.
- The second argument can be a service name or a port number represented with the decimal integer.
- The next two arguments are pointers to
addrinfo
structures. The first one ishints
that specifies requirements to filter the retrieved socket structures, while the second one is the pointer, where the function will dynamically allocate a linked list ofaddrinfo
structs.
Notice that, hints
structure should be set with zeros and then should be its members assigned. ai_family
member indicates the address family, e.g., IPv4 or IPv6 as AF_INET and AF_INET6, respectively. In this case, we are not interested in service name conversion and specify NULL
as the second argument to the function. Finally, we call getnameinfo
to translate the given sockaddr
structures to the printable form.
#include <netdb.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
int main(int argc, char const *argv[]) {
struct addrinfo hints;
struct addrinfo *res, *tmp;
char host[256];
memset(&hints, 0, sizeof(struct addrinfo));
hints.ai_family = AF_INET;
if (argc != 2) {
fprintf(stderr, "%s string\n", argv[0]);
exit(EXIT_FAILURE);
}
int ret = getaddrinfo(argv[1], NULL, &hints, &res);
if (ret != 0) {
fprintf(stderr, "getaddrinfo: %s\n", gai_strerror(ret));
exit(EXIT_FAILURE);
}
for (tmp = res; tmp != NULL; tmp = tmp->ai_next) {
getnameinfo(tmp->ai_addr, tmp->ai_addrlen, host, sizeof(host), NULL, 0,
NI_NUMERICHOST);
puts(host);
}
freeaddrinfo(res);
exit(EXIT_SUCCESS);
}
Sample Command:
./program localhost
Output:
127.0.0.1
127.0.0.1
127.0.0.1
Use the getnameinfo
Function to IP Address to Host Name in C
The getnameinfo
function is used in conjunction with getaddrinfo
in this case, and it retrieves the hostnames for corresponding IP addresses. Notice that we process the user input from the first command-line argument and pass it as the getaddrinfo
argument to retrieve the socketaddr
structs. Finally, each structure can be translated to the hostname string. Since the getaddrinfo
allocates dynamic memory to store the linked list in the fourth argument, this pointer should be freed by the user with the freeaddrinfo
function call.
#include <netdb.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
int main(int argc, char const *argv[]) {
struct addrinfo hints;
struct addrinfo *res, *tmp;
char host[256];
memset(&hints, 0, sizeof(struct addrinfo));
hints.ai_family = AF_INET;
if (argc != 2) {
fprintf(stderr, "%s string\n", argv[0]);
exit(EXIT_FAILURE);
}
int ret = getaddrinfo(argv[1], NULL, &hints, &res);
if (ret != 0) {
fprintf(stderr, "getaddrinfo: %s\n", gai_strerror(ret));
exit(EXIT_FAILURE);
}
for (tmp = res; tmp != NULL; tmp = tmp->ai_next) {
getnameinfo(tmp->ai_addr, tmp->ai_addrlen, host, sizeof(host), NULL, 0, 0);
}
freeaddrinfo(res);
exit(EXIT_SUCCESS);
}
Sample Command:
./program 127.0.0.1
Output:
localhost
localhost
localhost
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook