Usa la funzione getaddrinfo in C
-
Usa la funzione
getaddrinfo
per ospitare il nome nell’indirizzo IP in C -
Utilizzare la funzione
getnameinfo
per l’indirizzo IP al nome host in C
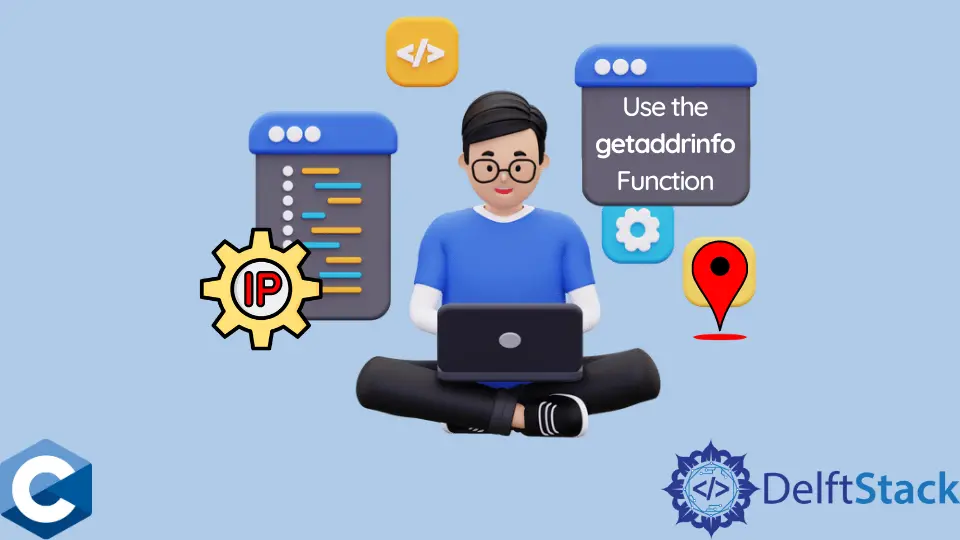
Questo articolo mostrerà diversi metodi su come utilizzare la funzione getaddrinfo
in C.
Usa la funzione getaddrinfo
per ospitare il nome nell’indirizzo IP in C
getaddrinfo
fa parte delle strutture di programmazione di rete UNIX e può convertire le informazioni dell’host di rete nell’indirizzo IP e viceversa. getaddrinfo
è anche una chiamata di funzione conforme a POSIX e può eseguire conversioni indipendentemente dai protocolli sottostanti.
getaddrinfo
accetta quattro argomenti,
- Il primo può essere il puntatore al nome host o alla stringa dell’indirizzo formattato IPv4 / IPv6.
- Il secondo argomento può essere un nome di servizio o un numero di porta rappresentato con il numero intero decimale.
- I prossimi due argomenti sono puntatori a strutture
addrinfo
. Il primo èhints
che specifica i requisiti per filtrare le strutture socket recuperate, mentre il secondo è il puntatore, dove la funzione allocherà dinamicamente una lista collegato di struttureaddrinfo
.
Si noti che la struttura dei hints
dovrebbe essere impostata con zero e quindi dovrebbero essere assegnati i suoi membri. Il membro ai_family
indica la famiglia di indirizzi, ad es. IPv4 o IPv6 come AF_INET e AF_INET6, rispettivamente. In questo caso, non siamo interessati alla conversione del nome del servizio e specifichiamo NULL
come secondo argomento della funzione. Infine, chiamiamo getnameinfo
per tradurre le strutture sockaddr
date nella forma stampabile.
#include <netdb.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
int main(int argc, char const *argv[]) {
struct addrinfo hints;
struct addrinfo *res, *tmp;
char host[256];
memset(&hints, 0, sizeof(struct addrinfo));
hints.ai_family = AF_INET;
if (argc != 2) {
fprintf(stderr, "%s string\n", argv[0]);
exit(EXIT_FAILURE);
}
int ret = getaddrinfo(argv[1], NULL, &hints, &res);
if (ret != 0) {
fprintf(stderr, "getaddrinfo: %s\n", gai_strerror(ret));
exit(EXIT_FAILURE);
}
for (tmp = res; tmp != NULL; tmp = tmp->ai_next) {
getnameinfo(tmp->ai_addr, tmp->ai_addrlen, host, sizeof(host), NULL, 0,
NI_NUMERICHOST);
puts(host);
}
freeaddrinfo(res);
exit(EXIT_SUCCESS);
}
Comando di esempio:
./program localhost
Produzione:
127.0.0.1
127.0.0.1
127.0.0.1
Utilizzare la funzione getnameinfo
per l’indirizzo IP al nome host in C
La funzione getnameinfo
è usata insieme a getaddrinfo
in questo caso, e recupera i nomi host per gli indirizzi IP corrispondenti. Si noti che elaboriamo l’input dell’utente dal primo argomento della linea di comando e lo passiamo come argomento getaddrinfo
per recuperare le strutture socketaddr
. Infine, ogni struttura può essere tradotta nella stringa del nome host. Poiché getaddrinfo
alloca memoria dinamica per memorizzare la lista collegata nel quarto argomento, questo puntatore dovrebbe essere liberato dall’utente con la chiamata alla funzione freeaddrinfo
.
#include <netdb.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
int main(int argc, char const *argv[]) {
struct addrinfo hints;
struct addrinfo *res, *tmp;
char host[256];
memset(&hints, 0, sizeof(struct addrinfo));
hints.ai_family = AF_INET;
if (argc != 2) {
fprintf(stderr, "%s string\n", argv[0]);
exit(EXIT_FAILURE);
}
int ret = getaddrinfo(argv[1], NULL, &hints, &res);
if (ret != 0) {
fprintf(stderr, "getaddrinfo: %s\n", gai_strerror(ret));
exit(EXIT_FAILURE);
}
for (tmp = res; tmp != NULL; tmp = tmp->ai_next) {
getnameinfo(tmp->ai_addr, tmp->ai_addrlen, host, sizeof(host), NULL, 0, 0);
}
freeaddrinfo(res);
exit(EXIT_SUCCESS);
}
Comando di esempio:
./program 127.0.0.1
Produzione:
localhost
localhost
localhost
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook