How to Format Specifiers in C
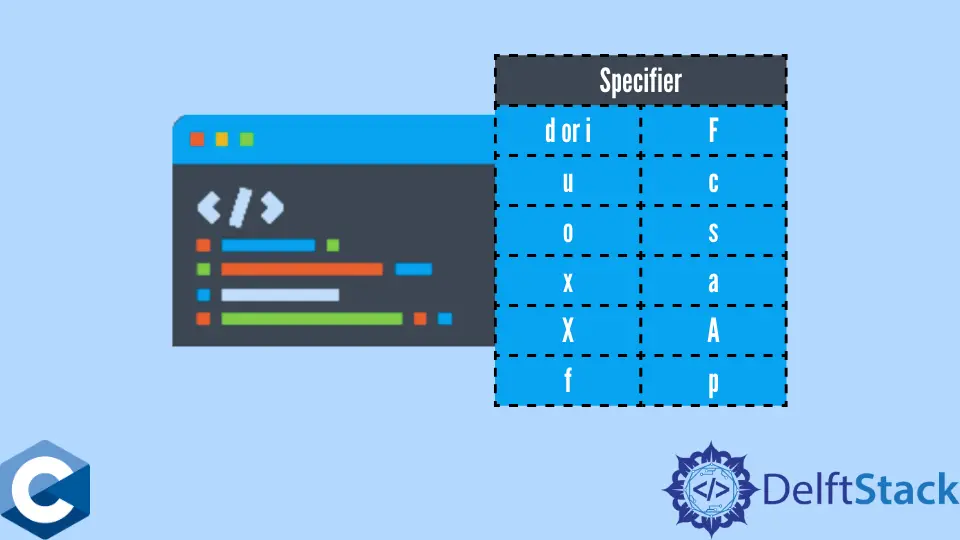
This article is about using the printf
function in C language and the format specifier used in this function. The article will discuss, in detail, the syntax of format specifiers and how they are used for different data types.
Let us look at the syntax of the printf
function first.
Format Specifiers in the printf()
Function in C
The syntax of printf()
function is:
int printf(const char* format, ...);
This function writes the string stored in the format
variable on the standard output console. If this string includes format specifiers,i.e., some sub-strings starting with the %
symbol to specify some variables, these are replaced with the values specified after the string.
Parameters:
format
The text to be written to output is stored in this C string. It can optionally include embedded format specifiers, replaced by the values supplied in the next extra arguments and formatted as needed.
A format specifier follows the below pattern:
%[flags][width][.precision][length]specifier
The square brackets []
indicate that these specifiers are optional. For example, printf("%lu",4294967295)
means print 4294967295
in unsigned long format.
Here, l
is a length specifier for long int
, and u
is a specifier for unsigned
numbers. The rest of the optional arguments are ignored.
The specifier
at the end of this pattern is the most significant part since it defines the type of data to be printed. The list of different specifiers and their descriptions is shown in the table below:
Specifier | Description |
---|---|
d or i |
signed decimal integer |
u |
unsigned decimal integer |
o |
unsigned octal number |
x |
unsigned hexadecimal number |
X |
unsigned hexadecimal in uppercase |
f |
decimal floating point in lowercase |
F |
decimal floating point in uppercase |
c |
character |
s |
string |
a |
hexadecimal floating-point in lowercase |
A |
hexadecimal floating-point in uppercase |
p |
pointer address |
The remaining sub-specifiers before the specifier are all optional, but their purpose is defined below.
Flags:
Flags | Description |
---|---|
- |
for left justifying the field. |
+ |
Forcefully place a plus sign before positive numbers |
# |
used for octal and hexadecimal specifiers (o,x,X) for placing 0, 0x or 0X respectively. |
0 |
adds 0 to the left of the value. |
Width:
width | Description |
---|---|
(number) |
specifies the minimum number of characters to be printed. |
* |
The width is supplied as an additional integer value argument preceding the argument to be formatted rather than in the format string. |
Precision:
Precision | Description |
---|---|
.number |
Specifies the minimum number of digits to be printed. If the number has fewer digits, then these are padded with zeros on the left. |
.* |
The precision is supplied as an additional integer value argument preceding the argument to be formatted rather than in the format string. |
Length:
Length | Description |
---|---|
hh |
Specifies printf to assume an int-sized integer argument promoted from a char for integer types. |
h |
Specifies printf to assume an int-sized integer argument promoted from a short for integer types. |
l |
Specifies printf to assume a long-sized integer argument for integer types. This is ignored for floating-point types. When using varargs , float arguments are automatically promoted to double. |
ll |
Specifies printf to assume a long large-sized integer argument for integer types. |
L |
Specifies printf to assume a long double argument for floating-point types. |
z |
For integer types, specifies printf to assume a size_t -sized integer argument. |
j |
For integer types, specifies printf to assume an intmax_t -sized integer argument. |
t |
For integer types, specifies printf to assume a ptrdiff_t -sized integer argument. |
...
The function may expect a sequence of additional arguments, each holding a value to replace a format specifier in the format string, depending on the format string (or a pointer to a storage location, for n).
The number of these parameters should, at least, be equal to the number of values indicated in the format specifiers. The function ignores any additional arguments.
Return Value:
The total amount of characters written is returned if the operation is successful. The error indication (ferror
) is set, and a negative integer is returned if a writing error occurs.
Errno
is set to EILSEQ
, and a negative integer is given if a multibyte character encoding error occurs while writing wide characters.
Example:
#include <stdio.h>
int main() {
printf("Some Character values: %c %c \n", 'b', 66);
printf("Some Decimal Value: %d %ld\n", 1234, 670000L);
printf("Numbers preceeding with blank spaces: %10d \n", 1234);
printf("Numbers preceeding with zeros: %010d \n", 1235);
printf("Different number systems: %d %x %o %#x %#o \n", 100, 100, 100, 100,
100);
return 0;
}
Output:
Some Character values: b B
Some Decimal Value: 1234 670000
Numbers preceeding with blank spaces: 1234
Numbers preceeding with zeros: 0000001235
Different number systems: 100 64 144 0x64 0144
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn