How to Use of extern C in C++
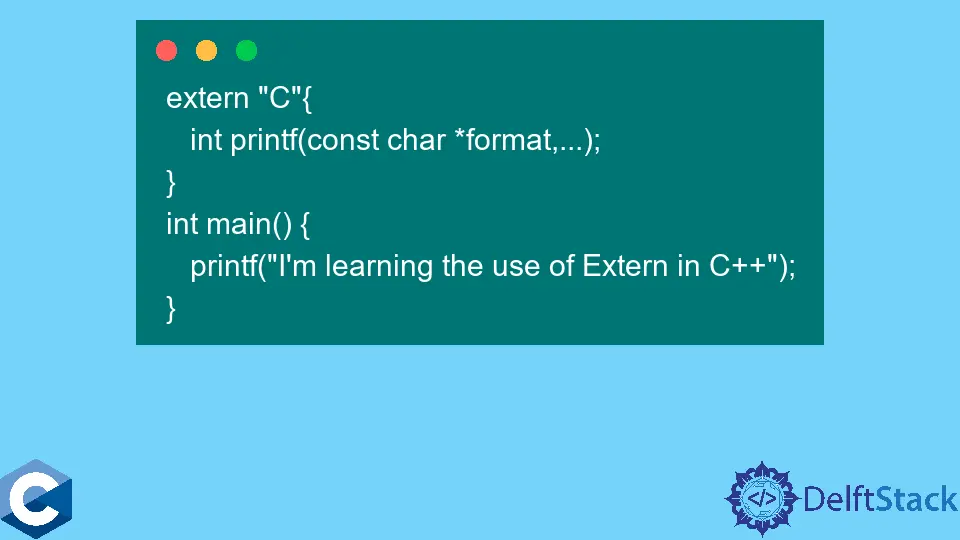
This tutorial talks about the name mangling in C++, the prerequisite for understanding the impact of extern "C"
in C++. Further, it educates about extern "C"
in C++ programming.
Use of extern "C"
in C++
We use the extern
keyword to define global variables, also known as external variables, and defined outside the method (function). We can use these variables throughout a program and modify the value using functions.
When the extern
keyword is used in the C++ file, a specific function name in C++ has a C linkage. In this situation, the compiler will not mangle the function name.
What is the name mangling in C++ programming?
It is a prerequisite for understanding the extern
keyword in C++. So, let’s learn the name mangling first.
The C++ programming supports the function overloading feature. We can have multiple functions with the same name but with a different number of arguments and their data type.
Here, the return
data type is not considered. The concern is how the overloaded functions are distinguished in the object code.
The function names are changed in object code by adding details about the respective arguments. The technique used here to add arguments’ information is Name Mangling.
Remember, there is no standardized technique in C++ programming for name mangling. That’s the reason various compilers use various techniques.
The following is the practical demonstration of name mangling in C++ programming. The code below has three overloaded functions named calculate
and one main
method.
Example Code:
int calculate(int number) { return number * number; }
double calculate(double number) { return number * number; }
float calculate(float number) { return number * number; }
int main(void) {
int number1 = calculate(3);
double number2 = calculate(3.38);
float number3 = calculate(3.00);
}
The C++ compiler changes the above code to differentiate between overloaded functions.
Example Code:
int __calculate_i(int number) { return number * number; }
double __calculate_d(double number) { return number * number; }
float __calculate_f(float number) { return number * number; }
int __main_v(void) {
int number1 = __calculate_i(3);
double number2 = __calculate_d(3.38);
float number3 = __calculate_f(3.00);
}
C programming does not support the function overloading. So, we need to ensure that the function names are not changed when we link the C code to a C++ file.
The code snippet below generates an error because the compiler changes the printf()
function’s name and can’t find the definition of the changed/updated printf()
function.
Example Code:
int printf(const char *format, ...);
int main() { printf("I'm learning the use of Extern in C++"); }
We use the extern
keyword in C++ programming to eliminate this issue. Whenever the C++ compiler finds the code inside the extern "C" {}
block, it makes sure that the name of the function remains un-mangled.
It means the function’s name will not be changed, which will let the program execute successfully. Remember, different compilers may generate different outputs for these code blocks.
Example Code:
extern "C" {
int printf(const char *format, ...);
}
int main() { printf("I'm learning the use of Extern in C++"); }
Output:
I'm learning the use of Extern in C++
So, the extern "C"
is a linkage specification that must be within the scope of the namespace
. The extern "C"
would ignore the class members.
We must use this keyword whenever we want to link the C code in a C++ file.