End of File (EOF) in C
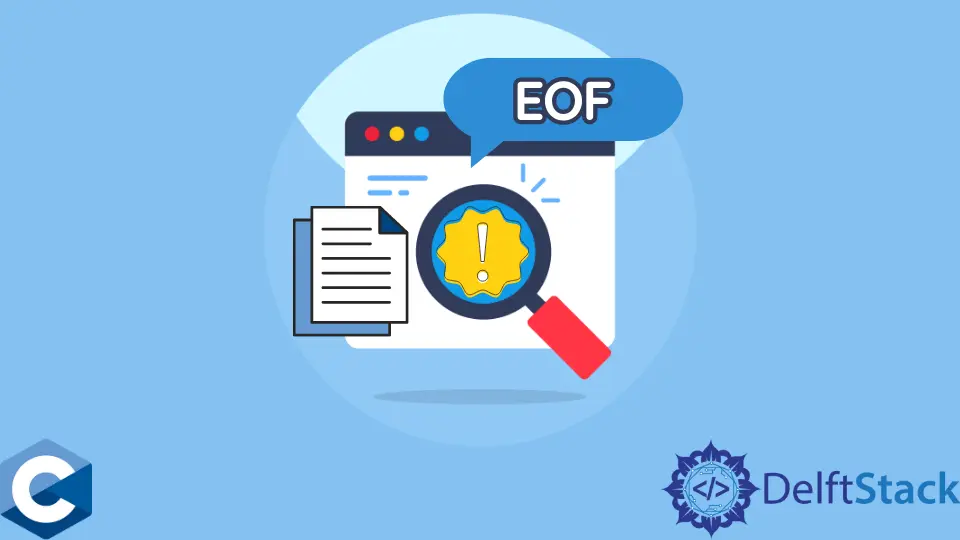
The topic of expressing EOF in C will be the focus of this article.
End Of File
(EOF) in C
The circumstance known as the end-of-file
occurs in an operating system of a computer when there’s no longer any data that can be read from a data source. The data source is often referred to as a file
or stream
.
The input has been completed when the End of the File (EOF)
message is shown.
After entering the content, we may terminate the text by pressing the Ctrl and Z keys simultaneously. This signals that the file has reached its conclusion, and there is nothing more to read.
When the end of the file is reached in C, the getc()
function returns EOF
. getc()
will also return end-of-file (EOF)
if it is unsuccessful.
Therefore, it is not sufficient to only compare the value provided by getc()
with EOF
to determine whether or not the file has reached its end. C offers the feof()
function to solve this issue.
This function will return a value greater than zero only if the end of the file has been reached; else, it will return 0
.
Operations to a File in C
The majority of programs are developed to save the information that is retrieved from the program. One of these methods is to save the information obtained in a file.
The following is a list of the various operations that may be carried out on a file.
Create a File
Using the fopen
program and specifying characteristics such as a
, a+
, w
, and w++
makes it possible to create a new file.
Open a File
The fopen
function and the appropriate access modes are used whenever a file is opened. The following are some file access modes that are most often used.
-
Pressing
a
will search the file. If the file is successfully opened, thefopen()
function loads it into memory and creates a pointer that links to the character now being read from it.If the file doesn’t already exist, a new one will be produced. Returns
Null
if unable to open a file.
-
The letter
w
searches the file. If the file already exists, the file’s contents will be rewritten.If the file doesn’t already exist, a new one will be produced. If the file cannot be opened,
Null
will be returned.
To carry out the activities that need to be done on the file, a specialized pointer known as the File pointer
is used, and its declaration looks like this:
FILE *fp;
Therefore, it is possible to open the file using:
fp = fopen("Shanii.txt", "w");
Here, Shanii.txt
is the file we want to be opened, and the second parameter is the file mode.
Reading a File
The functions fscanf
and fgets
are used to carry out the read operations on the files. Both of these methods performed the same actions as scanf
and gets
, but they considered an extra argument called the file pointer
.
You have the choice to read the file character by character or line by line, depending on how you wish to organize the information. The following is an example of the code needed to read a file.
FILE* fp;
fp = fopen("shanii.txt", "r");
fscanf(fp, "%s %s %s %d", str1, str2, str3, &year);
Write a File
The functions fprintf
and fputs
, which are used for reading files, may also be used to write files, and the process is quite similar to reading files. The command to use when writing to a file looks like this:
FILE *fp;
fp = fopen("shanii.txt", "w");
fprintf(fp, "%s %s %s %d", "I", "am", "Zeeshan", 2000);
Closing a File
You must always close a file after all of its actions have been completed successfully. You must use the fclose
function to close a file successfully.
The code for closing a file is shown here:
FILE *fp;
fp = fopen("fileName.txt", "w");
fprintf(fp, "%s %s %s %d", "I", "am", "Zeeshan", 2000);
fclose(fp);
Example of EOF in C
For example, look at the following C code to display the contents of a text file named shanii.txt
on the screen.
The value of getc()
is first compared to EOF
.
int charac = getc(filepointer);
while (charac != EOF) {
putchar(charac);
charac = getc(filepointer);
}
Then, a second check is performed using feof()
. This check ensures that the program only outputs Program has reached End-of-file
if the end of the file has been reached.
if (feof(filepointer)) {
printf("\n Program has reached End-of-file");
}
Else the program will display There was a problem
.
Source Code:
#include <stdio.h>
int main() {
FILE *filepointer = fopen("shanii.txt", "r");
int charac = getc(filepointer);
while (charac != EOF) {
putchar(charac);
charac = getc(filepointer);
}
if (feof(filepointer)) {
printf("\n Program has reached End-of-file");
} else {
printf("\n There was a problem");
}
fclose(filepointer);
getchar();
return 0;
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn