The dup2 Function in C
- What is the dup2 Function?
- Using dup2 for Standard Output Redirection
- Using dup2 for Standard Input Redirection
- Conclusion
- FAQ
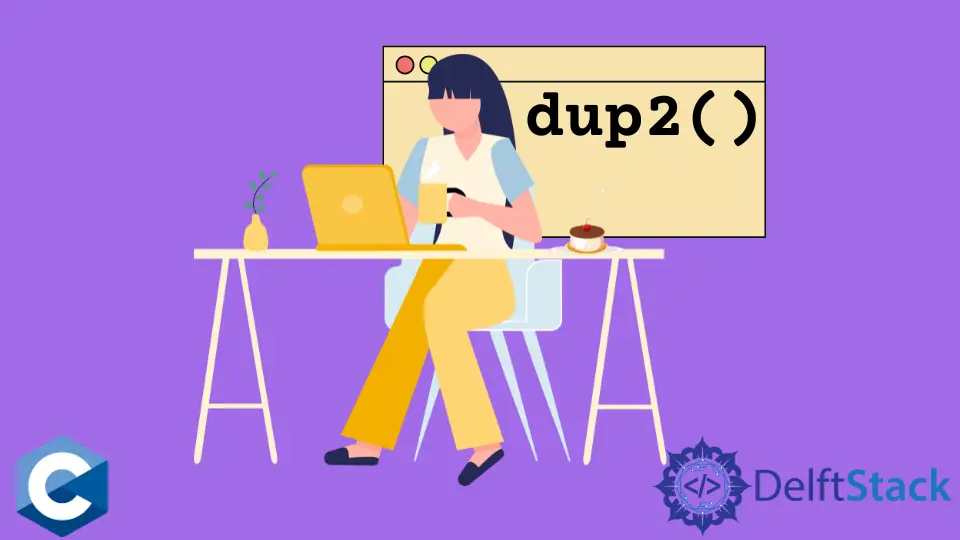
When working with system-level programming in C, the dup2 function is an essential tool for managing file descriptors. This function allows you to duplicate one file descriptor to another, effectively redirecting input and output streams within your program. Understanding how to use dup2 can significantly enhance your ability to control data flow in your applications, especially when you’re dealing with processes and pipes.
In this article, we will delve into what the dup2 function is, how it works, and provide practical examples to illustrate its utility. By the end, you’ll be equipped with the knowledge to implement dup2 effectively in your C programs.
What is the dup2 Function?
The dup2 function is part of the POSIX standard and is used for duplicating file descriptors in C. The function prototype is as follows:
int dup2(int oldfd, int newfd);
Here, oldfd
is the file descriptor you want to duplicate, and newfd
is the file descriptor you want to overwrite or create. If newfd
is already open, it will be closed before being reused. The function returns the new file descriptor on success or -1 on failure, setting errno
to indicate the error.
Using dup2 is particularly useful in scenarios where you want to redirect standard input, output, or error streams, such as redirecting output to a file or using pipes between processes.
Using dup2 for Standard Output Redirection
One common use case for dup2 is redirecting the standard output (stdout) to a file. This can be particularly useful for logging or saving output from a program that typically goes to the console. Here’s a simple example demonstrating how to redirect stdout to a file using dup2.
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
int main() {
int file = open("output.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (file < 0) {
perror("open");
return 1;
}
dup2(file, STDOUT_FILENO);
close(file);
printf("This will be written to the file instead of the console.\n");
return 0;
}
Output:
This will be written to the file instead of the console.
In this example, we first open a file named output.txt
for writing. The open
function returns a file descriptor, which we then pass to dup2
. By using dup2
, we replace the standard output file descriptor with our new file descriptor. After the call to dup2
, any output sent to stdout will go to output.txt
instead of the console. Finally, we close the original file descriptor to free up resources.
Using dup2 for Standard Input Redirection
Another common application of dup2 is redirecting standard input (stdin). This can be particularly useful when you want a program to read input from a file instead of the keyboard. Here’s an example that illustrates how to achieve this.
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
int main() {
int file = open("input.txt", O_RDONLY);
if (file < 0) {
perror("open");
return 1;
}
dup2(file, STDIN_FILENO);
close(file);
char buffer[100];
fgets(buffer, sizeof(buffer), stdin);
printf("Read from file: %s", buffer);
return 0;
}
Output:
Read from file: (content of input.txt)
In this example, we open a file named input.txt
for reading. We then use dup2
to redirect stdin to this file. After the redirection, any calls to fgets
that read from stdin will actually read from input.txt
. This allows for seamless integration of file input into programs that are designed to read from standard input.
Conclusion
The dup2 function is a powerful tool in C programming, particularly for managing file descriptors and redirecting input and output streams. Whether you’re redirecting standard output to a file or reading input from a file instead of the keyboard, dup2 offers a straightforward solution. By mastering this function, you can enhance your ability to control data flow in your applications, making your programs more versatile and efficient. Remember that proper error handling and resource management are key when using functions like dup2, so always check return values and close file descriptors when you’re done.
FAQ
-
What is the purpose of the dup2 function in C?
dup2 is used to duplicate a file descriptor, allowing you to redirect input and output streams in your programs. -
Can dup2 be used for both input and output redirection?
Yes, dup2 can redirect both standard input and standard output by duplicating the respective file descriptors. -
What happens if the new file descriptor is already open when using dup2?
If the new file descriptor is already open, dup2 will close it before duplicating the old file descriptor to it. -
How do I check for errors when using dup2?
You can check for errors by examining the return value of dup2. If it returns -1, you can use the errno variable to determine the cause of the error. -
Is dup2 a standard C function?
dup2 is part of the POSIX standard and is commonly used in Unix-like operating systems.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook