How to Throw an Exception in C
- an Overview of Exception
- Handle an Exception in C Programming Language
-
the
setjmp()
Function to Handle Errors in C -
Implement Error Handling With
errno
in C -
Use
perror()
andstrerror()
to Print the Error Messages - Handling Dividing by Zero Errors
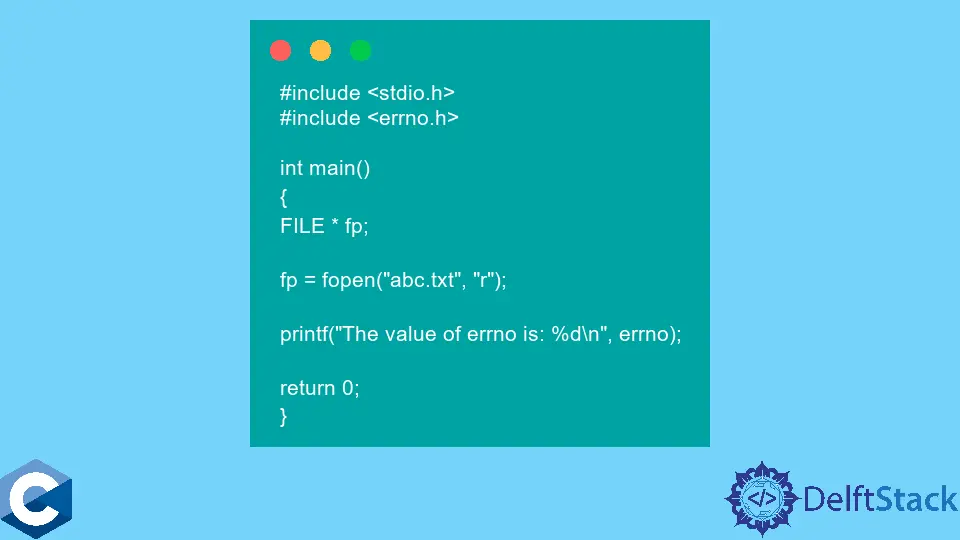
This article explains how to deal with an exception or error in the C programming language.
an Overview of Exception
An attempt to divide by zero is an example of a situation that might trigger an exception, which is a reaction to an extraordinary scenario that can occur while a program is being executed.
Control may be passed from one section of a program to another with the help of an exception.
Handle an Exception in C Programming Language
Even though C does not provide direct support for error handling (also known as exception handling), there are methods in which error handling may be accomplished in C. To avoid problems in the first place, a programmer must take precautions and examine the values returned by the functions.
In the event of an error, many calls to C functions return the value -1
or NULL
. Hence, performing a rapid test on these return values may be accomplished quickly and efficiently using, for example, an if
statement.
the setjmp()
Function to Handle Errors in C
Simulating the exception handling functionality of other programming languages is possible using the setjmp()
function. The first time setjmp()
is called, a reference point is stored to the current point in the program’s execution.
This reference point will remain valid so long as the function that contains setjmp()
does not return or exit. When you make a call to longjmp()
, the execution will return to the point where the corresponding setjmp()
call occurred.
setjmp()
’s parameter is jmp buf
, which is a type that may contain an execution context, and it returns 0
on the very first occasion that it is executed (when it sets the return point). The value supplied to longjmp()
is then returned when the program is performed for a second time, when longjmp()
is called.
A jmp buf
previously supplied to setjmp()
is required as an input for longjmp()
, along with a value that should be passed to setjmp()
as the return value.
When failures occur in deeply nested function calls, it would be tiresome to verify return values back to the place you desire to return to. This is where setjmp()
and longjmp()
comes in handy the most.
Source Code:
#include <setjmp.h>
#include <stdio.h>
#include <stdlib.h>
int main(void) {
int value;
jmp_buf environment;
value =
setjmp(environment); // value is set to 0 the first time this is called
if (value != 0) {
printf("You have just executed a longjmp call, and the return value is %d.",
value); // now, value is 1, passed from longjmp()
exit(0);
}
puts("Performing longjmp call\n");
longjmp(environment, 1);
return (0);
}
Output:
Performing longjmp call
You have just executed a longjmp call, and the return value is 1
Implement Error Handling With errno
in C
When a function call is executed in C, a variable known as errno
receives an automatic assignment of a code (value) that may be used to determine the problem encountered. This code can be used to pinpoint where the error occurred.
It is a global variable that tells you what kind of error happened when you called any function, and it is specified in the errno.h
header file. Errors of various kinds are denoted by distinct codes or values for the errno
variable.
The following is a list of many distinct errno
values, each corresponding to a specific meaning.
errno Value |
Error Message |
---|---|
1 | Operation not permitted |
2 | No such file or directory |
3 | No such process |
4 | Interrupted system call |
5 | I/O error |
6 | No such device or address |
7 | Argument list too long |
8 | Exec format error |
9 | Bad file number |
10 | No child processes |
11 | Try again |
12 | Out of memory |
13 | Permission denied |
Source Code:
#include <errno.h>
#include <stdio.h>
int main() {
FILE* fp;
fp = fopen("abc.txt", "r");
printf("The value of errno is: %d\n", errno);
return 0;
}
Output:
The value of errno is: 2
In this case, the errno
is set to 2, which indicates that there is neither a file nor a directory with that name.
Use perror()
and strerror()
to Print the Error Messages
The sorts of errors that were encountered are indicated by the errno
value that was obtained above.
If it is necessary to display the error description, then two functions may be used to show a text message connected to the error no
.
The perror()
displays the string you provide, inserts a space, then the colon, and finally, it describes the errno
value. The strerror()
refers to the description of the current value of errno
.
Source Code:
#include <errno.h>
#include <stdio.h>
#include <string.h>
int main() {
FILE *fp;
fp = fopen(" abc.txt ", "r");
printf("The value of errno is: %d\n", errno);
printf("The error message is: %s\n", strerror(errno));
perror("perror Message");
return 0;
}
Output:
The value of errno is: 2
The error message is: No such file or directory
Message from perror: No such file or directory
Handling Dividing by Zero Errors
When dealing with errors or exceptions, this is by far the most common issue that is brought up for discussion.
Frequently, we will present mathematical assertions such as:
c = a / b;
But we often forget that the value of b
is likely to be zero
in some circumstances.
The division of an integer by zero
is not allowed in mathematics. People often believe that the solution can never be reached, meaning the solution is infinite.
Unfortunately, this is not the case. In this particular scenario, the correct response is not specified
. Using the principles of managing exceptions and errors in C, the following is a program that will resolve this issue.
Source Code:
#include <stdio.h>
#include <stdlib.h>
int main() {
int dividend;
int divisor;
int quotient;
printf("Enter the value for dividend: ");
scanf("%d", ÷nd);
printf("Enter the value for divisor: ");
scanf("%d", &divisor);
if (divisor == 0) {
fprintf(stderr, "\nDivision by zero is not possible!\n");
exit(-1);
}
quotient = dividend / divisor;
fprintf(stderr, "The value of quotient: %d\n", quotient);
exit(0);
return 0;
}
Output:
Input a zero
value.
Enter the value for dividend: 100
Enter the value for divisor: 0
Division by zero is not possible!
Input a non-zero
value.
Enter the value for dividend: 100
Enter the value for divisor: 5
The value of quotient: 20
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn