Regular Expressions in C
- Regular expressions in C
-
Construct a Regular Expression Using the
regcomp()
Method in C -
Compare Strings to a Compiled Regular Expression Using the
regexec()
Method in C
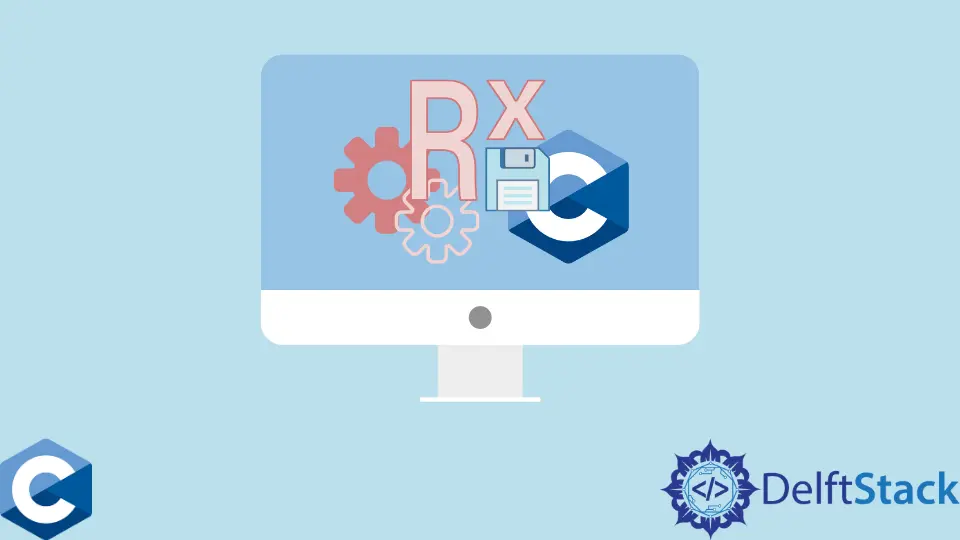
Regular expressions in C will be the topic of discussion in this article, along with an example.
Regular expressions in C
Regular expressions, consisting of a string of characters, may be used to discover search patterns. String matching and other similar applications make extensive use of it.
They are a standardized approach to matching pattern sequences with character sequences. C, C++, Java, and many other major programming languages use it.
After learning about regular expressions, let’s look at some POSIX-specific library patterns.
- Finding the letters or numbers enclosed by brackets is easy using
[]
. [:number:]
can be used to locate any number.[:word:]
can search for letters, digits, and underscores.[:lower:]
is used to locate the alphabet’s lowercase letters.
Construct a Regular Expression Using the regcomp()
Method in C
The regular expression regcomp()
method is used for compiling or constructing regular expressions. It requires the regex, expression, and flag.
The expression is a string type, regex is a reference to a memory region where the expression is matched and saved, and the flag is used to identify the kind of compilation.
It will return the value 0 if the compilation has been completed without error. And it will output Error code
if the expression cannot be compiled successfully.
Syntax:
regcomp(®ex, expression, flag)
Example:
-
Create a variable of the regex type, and give it a name like we did
r1
.regex_t r1;
-
Now, you need to build a variable that will hold the result of the regex generation process, as you can see below:
int regval;
-
Generate a regex by calling the function:
regval = regcomp(&r1, "[:word:]", 0);
-
Regular Expression compiled successfully.
will be shown after the compilation process.if (value == 0) { printf("Regular Expression compiled successfully."); }
-
Else, it will throw an exception with the message
An Error Occurred.
.else { printf("An Error Occurred."); }
Complete Source Code:
#include <regex.h>
#include <stdio.h>
int main() {
regex_t r1;
int regval;
regval = regcomp(&r1, "[:word:]", 0);
if (regval == 0) {
printf("Regular Expression compiled successfully.");
} else {
printf("An Error Occurred.");
}
return 0;
}
Output:
Regular Expression compiled successfully.
Compare Strings to a Compiled Regular Expression Using the regexec()
Method in C
It is possible to compare a string to a pattern using the regexec()
function. It considers some parameters: the first is a pattern that has been precompiled, a string that contains the pattern to be looked for, information about the location of matches, and flags that describe a change in the behavior of matches.
Syntax:
regexec(®ex, expression, 0, NULL, 0);
If there is a match, this will return the value 0. And if there is no match, it will return REG NOMATCH
.
Example:
-
To begin, we will create a function named
DisplayPattern
which will display the result. If the value is 0, it will showPattern found
; if it is not equal to 0, it will simply showPattern not found.
; else, it will show an error prompt with the messageAn error occurred.
.void DisplayPattern(int val) { if (val == 0) { printf("Pattern found.\n"); } else if (val == REG_NOMATCH) { printf("Pattern not found.\n"); } else { printf("An error occurred.\n"); } }
-
Now, we’ll create the
Main
function where we’ll create aregex_t
type variable namedr1
.regex_t r1;
-
Create three variables for the return type named
data1
,data2
,data3
.int data1; int data2; int data3;
-
Then, create a regEx and compare the pattern with the string in
reg
.data1 = regcomp(&r1, " I am a writing c language", 0); data1 = regexec(&r1, "Hi there its me Zeeshan", 0, NULL, 0);
-
Add a few more regEx as shown below, and compare the pattern with the string in
reg
.data2 = regcomp(&r1, "I am a writing c language", 0); data2 = regexec(&r1, " Lets do coding", 0, NULL, 0); data3 = regcomp(&r1, "My Name is Zeeshan Khan", 0); data3 = regexec(&r1, " My Name is Zeeshan Khan", 0, NULL, 0);
-
At last, display patterns using the
DisplayPattern
function that we created before.DisplayPattern(data1); DisplayPattern(data2); DisplayPattern(data3);
Complete Source Code:
#include <regex.h>
#include <stdio.h>
void DisplayPattern(int val) {
if (val == 0) {
printf("Pattern found.\n");
} else if (val == REG_NOMATCH) {
printf("Pattern not found.\n");
} else {
printf("An error occurred.\n");
}
}
int main() {
regex_t r1;
int data1;
int data2;
int data3;
data1 = regcomp(&r1, " I am a writing c language", 0);
data1 = regexec(&r1, "Hi there its me Zeeshan", 0, NULL, 0);
data2 = regcomp(&r1, "I am a writing c language", 0);
data2 = regexec(&r1, " Lets do coding", 0, NULL, 0);
data3 = regcomp(&r1, "My Name is Zeeshan Khan", 0);
data3 = regexec(&r1, " My Name is Zeeshan Khan", 0, NULL, 0);
DisplayPattern(data1);
DisplayPattern(data2);
DisplayPattern(data3);
return 0;
}
Output:
Pattern not found.
Pattern not found.
Pattern found.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn