Step Scope in Spring Batch
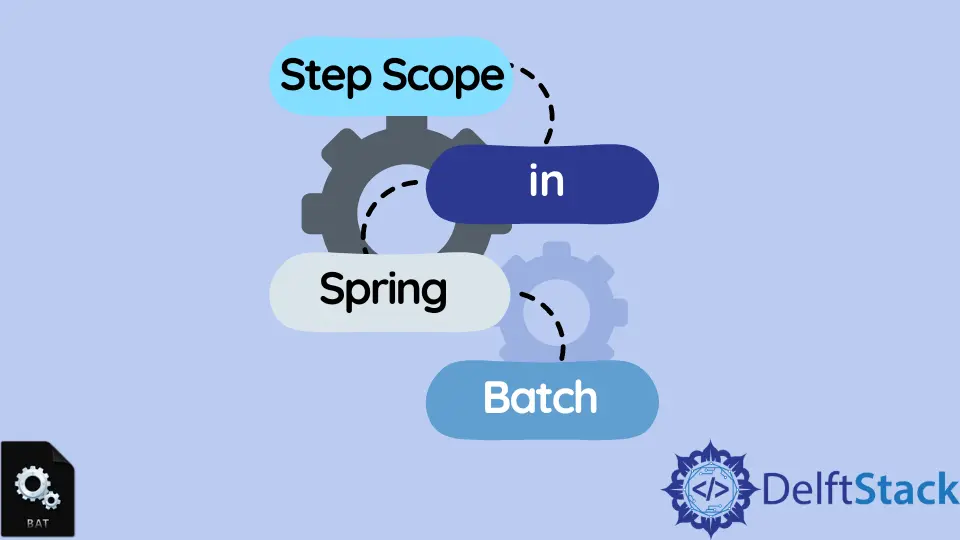
This tutorial teaches about working of Step Scope in Spring Batch. Spring Batch has three major high-level components: Application, Batch Core, and Batch Infrastructure.
Job and Step in Spring Batch
A job is an entity that encapsulates entire batch processing; i.e., it is at the top of a job hierarchy. Every job contains one or more steps.
It is a container for step instances where each step has one ItemReader
, ItemProcessor
and ItemWriter
. Some of the concepts a job includes are JobInstance
, JobParameters
, and JobExecution
.
A step is an independent domain object of a batch job that contains all the necessary information to define and control the batch process. Like Job, a Step has a StepExecution
that correlates with a JobExecution
as in a Job.
Spring defines two scopes, namely StepScope
and JobScope
.
Step Scope in Spring Batch
Beans that use late binding must always be declared with the step as scope. This can be done by setting scope="step"
, but the step scope cannot be used with the step bean.
If needed, the components in a step should be step-scoped instead. In Spring Batch, a Job is wired with either an XML config file or Java-based configuration, and the configuration is called JobConfiguration
.
The example shown below shows binding to step scope in XML and JAVA.
XML Configuration:
<bean id="flatFileItemReader" scope="step"
class="org.springframework.batch.item.file.FlatFileItemReader">
<property name="resource" value="#{jobParameters[input.file.name]}" />
</bean>
Java-based Configuration:
@StepScope
@Bean
public FlatFileItemReader flatFileItemReader(@Value("
#{jobParameters[input.file.name] } ") String name) {
return new FlatFileItemReaderBuilder<Foo>()
.name("flatFileItemReader")
.resource(new FileSystemResource(name))
...
}
The StepScope
object is useful in many ways. One of the reasons is that StepScope
helps define the beans’ lifespan.
By default, the bean scope is a singleton. When a bean is annotated with @singleton
, it will be created once in the beginning and destroyed at the end, but a StepScope
is unique to a specific step, not a singleton.
By specifying a component as a StepScope
, the spring container will start a new instance for each step. The lifespan of a step-scoped bean depends on the step’s lifespan.
In a step scoped bean, the beans will be created at the beginning and destroyed at the end of each step. The annotation is @StepScope
.
Another reason to use StepScope
is when steps are executed in parallel, as it will isolate the bean’s state. Through this, each thread in each step has its instance and does not modify the state managed by the other thread.
If not, multiple threads will change the beans’ state, making it inconsistent. Also, through StepScope
, information can be passed between steps through the stepExecutionContext
and stepExecutionListener
while performing the late binding of parameters.
Thus, StepScope
helps define the beans’ lifespan and allows us to pass data across steps. The following example shows the use of stepExecutionContext
in XML and JAVA configuration.
XML Configuration:
<bean id="flatFileItemReader" scope="step"
class="org.springframework.batch.item.file.FlatFileItemReader">
<property name="resource" value="#{stepExecutionContext['input.file.name']}" />
</bean>
Java-based Configuration:
@StepScope
@Bean
public FlatFileItemReader flatFileItemReader(
@Value("#{stepExecutionContext['input.file.name']}") String name) {
return new FlatFileItemReaderBuilder<Foo>()
.name("flatFileItemReader")
.resource(new FileSystemResource(name))...
}
We discussed how the StepScope
object works in Spring Batch, along with examples. To know more about Spring Batch, you can refer to the official documentation of the Spring Batch.