How to String Comparison in Batch File
-
String Comparison Using the
if
andif-else
Commands in Batch File -
String Comparison Using the
for
Loop in Batch File
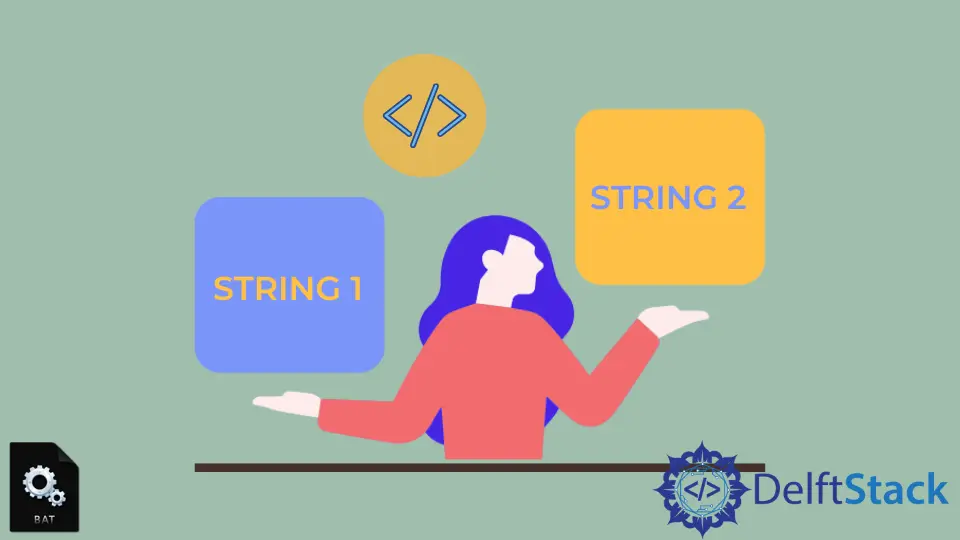
A string is an ordered collection of characters.
Strings can be compared using conditional commands in a Batch file, i.e., if
, if-else
, and for
commands. Strings may contain spaces and special characters, which can cause errors in the Batch file on normal execution.
Also, if the string contains a double quote, it can cause an error.
This tutorial will teach different ways of comparing strings in a Batch file using if
, if-else
, and for
commands.
String Comparison Using the if
and if-else
Commands in Batch File
The if
command performs conditional operations and logical comparisons between a set value and an expected value. It can check if a file exists, compare strings, and even check for errors.
String Comparison Using the if
Command
Let’s take an example to check whether the strings are equal. If they are equal, it will display the output with the echo
command.
@echo off
SetLocal
set string1="Hello World"
set string2="Hello World"
if %string1% == %string2% (echo "Both the strings are equal")
cmd /k
Output:
String Comparison Using the if-else
Command
@echo off
SetLocal
set string1="Hello World"
set string2="HELLO WORLD"
set string3="Hello World"
if %string1% == %string2% (echo string 1 and string 2 are equal echo the string is %string2%) else if %string1% == %string3% (echo string1 and string 3 are equal
echo the string is %string3%) else (echo all strings are different)
cmd /k
output:
Note:
- Don’t leave spaces between the brackets.
- When the strings or variables contain spaces or special characters, put them in double quotes.
With the if
command, you can use /I
for a case insensitive string comparison and NOT
to run the command if the condition is false.
You can also use comparison operators such as EQU
(equal), NEQ
(not equal), LSS
(less than), LEQ
(less than or equal to), GTR
(greater than or equal to), GEQ
(greater than or equal to) for comparing the values. But, these operators can not be used to compare strings.
Comparison of Strings Containing Double-Quotes Using the if-else
Command
If the string or the variables contains double quotes, enable delayed expansion with the SetLocal
command. Use !
instead of "
.
The code for the same is shown in the example below:
@echo off
SetLocal EnableDelayedExpansion
set string1="Hello "World""
set string2=""HELLO WORLD""
set string3="Hello World"
if !string1! == !string2! (echo "string 1 and string 2 are equal") else if !string1! == !string3! (echo "string1 and string 3 are equal") else (echo "All strings are different")
cmd /k
Output:
String Comparison Using the for
Loop in Batch File
The for
command is used to run a specified command for each file within a set of files.
The syntax of the for
command in a Batch file is shown.
for {%%|%}<variable> in (<set>) do <command> [<commandlineoptions>]
Let’s take an example where we have to compare the strings to show the Windows OS version:
@echo off
SetLocal EnableDelayedExpansion
for /f "usebackq tokens=1 delims=" %%I in ("C:\Users\Aastha Gas Harda\Desktop\testfile1.txt") do (
set string=%%I
echo %%I
if "%%~I" == "HelloWorld" (echo "match found") else (echo "match not found")
)
PAUSE
Output:
In the above example, a for
loop is used to compare the string value. If the string value is equal to HelloWorld
, it will display the output as match found
.
The delims
specify a delimiter set, and the tokens
specify which tokens from each line will be passed to the for
loop. The usebackq
must be used whenever we use quotes in the for
loop.
So, we have discussed different ways of comparing strings in Batch files. Other than comparing strings, these conditional commands can also be used to find specified values or text from text files or log files.