How to Create a Function in Batch Script
- Understanding Functions in Batch Script
- Creating a Simple Function
- Passing Parameters to a Function
- Returning Values from a Function
- Error Handling in Functions
- Conclusion
- FAQ
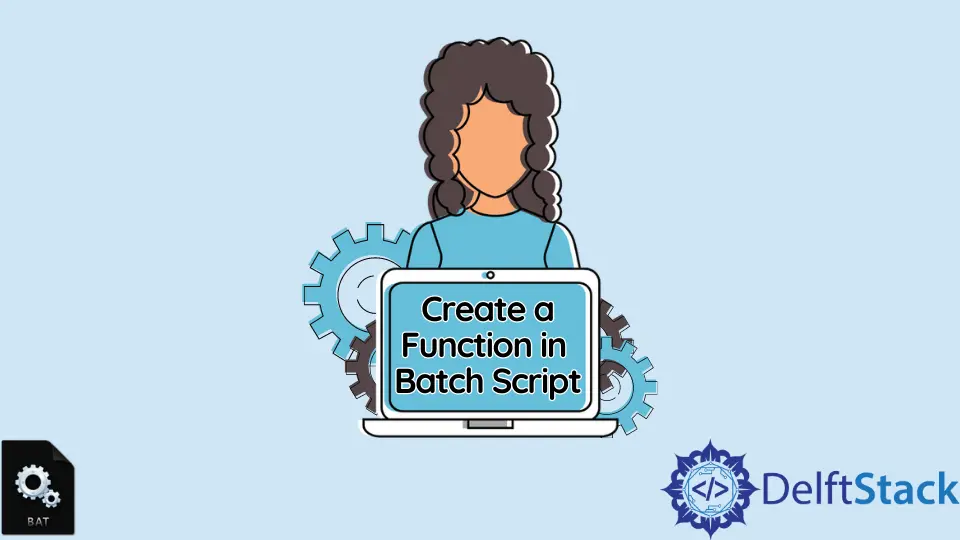
Creating functions in Batch Script can significantly enhance your scripting capabilities, allowing you to write cleaner, more efficient code. If you’re familiar with programming concepts, you know that functions help in organizing code, making it reusable and easier to manage.
In this tutorial, we will walk you through the steps to create a function in Batch Script. Whether you’re automating tasks or managing system configurations, understanding how to implement functions will boost your productivity. So, let’s dive in and explore the world of Batch Script functions!
Understanding Functions in Batch Script
Before we get into the nitty-gritty of creating functions, it’s essential to understand what a function is in the context of Batch Script. A function is a block of code designed to perform a specific task. This block can be called multiple times throughout your script, which not only saves time but also reduces the chances of errors.
In Batch Script, functions are typically defined using a label followed by the call
command to execute them. This structure allows for better organization of your code and makes it easier to debug.
Now, let’s look at how you can create and use functions in Batch Script effectively.
Creating a Simple Function
Creating a simple function in Batch Script involves defining a label for your function and then calling it. Here’s how you can do it:
@echo off
:myFunction
echo Hello, this is my function!
goto :eof
call :myFunction
Output:
Hello, this is my function!
In this example, we define a function called myFunction
using a label. The echo
command inside the function outputs a simple message. After defining the function, we use the call
command to execute it. The goto :eof
command is used to exit the function and return to the point where it was called. This structure allows you to reuse the function throughout your script.
Passing Parameters to a Function
Functions become even more powerful when you can pass parameters to them. This feature allows you to customize the behavior of your functions based on the input you provide. Here’s how to create a function that accepts parameters:
@echo off
:myFunction
setlocal
set name=%1
echo Hello, %name%! This is my function.
endlocal
goto :eof
call :myFunction John
Output:
Hello, John! This is my function.
In this example, we modify the myFunction
to accept a parameter called name
. The %1
represents the first argument passed to the function. We use setlocal
and endlocal
to create a local environment for the function, ensuring that any changes to variables do not affect the global environment. When we call the function with John
as an argument, it outputs a personalized greeting. This method enhances the flexibility of your functions.
Returning Values from a Function
Batch Script does not have a built-in return value mechanism like many other programming languages. However, you can simulate returning values by using environment variables. Here’s an example of how to return a value from a function:
@echo off
:myFunction
setlocal
set /a result=%1 + %2
endlocal & set result=%result%
goto :eof
call :myFunction 5 10
echo The result is %result%.
Output:
The result is 15.
In this code snippet, we create a function that adds two numbers. The set /a
command performs arithmetic operations. After calculating the result, we use endlocal & set
to pass the value back to the global environment. When we call the function with 5
and 10
, it calculates the sum and outputs the result. This approach allows you to effectively retrieve values from your functions.
Error Handling in Functions
Error handling is a crucial aspect of any scripting language, including Batch Script. You can implement basic error handling in your functions to manage unexpected situations. Here’s how to create a function that checks for valid input:
@echo off
:myFunction
if "%1"=="" (
echo Error: No input provided!
exit /b 1
)
echo You entered: %1
goto :eof
call :myFunction
call :myFunction Hello
Possible Output:
Error: No input provided!
You entered: Hello
In this example, the function checks if an argument is provided. If no input is detected, it outputs an error message and exits with a non-zero status. If an argument is provided, it simply echoes the input. This method allows your script to handle errors gracefully and provide feedback to the user.
Conclusion
Creating functions in Batch Script is a straightforward process that can significantly improve the efficiency and organization of your scripts. By mastering the techniques outlined in this tutorial, you can write cleaner code, manage complex tasks, and handle errors effectively. Functions are a powerful tool in your scripting arsenal, enabling you to automate tasks and streamline your workflow. Start implementing functions in your Batch Scripts today and experience the difference they can make!
FAQ
-
What is a function in Batch Script?
A function in Batch Script is a block of code that performs a specific task and can be reused throughout the script. -
How do I call a function in Batch Script?
You can call a function using thecall
command followed by the function’s label. -
Can I pass parameters to a function in Batch Script?
Yes, you can pass parameters to a function using command-line arguments, which can be accessed within the function using%1
,%2
, etc. -
How do I return a value from a function in Batch Script?
You can simulate returning a value by using environment variables to store the result and then access it outside the function.
- Is error handling possible in Batch Script functions?
Yes, you can implement basic error handling by checking input conditions and providing feedback to the user.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn