How to Implement Error Handling in Batch Script
- Understanding Error Levels in Batch Scripts
- Using Conditional Statements for Error Handling
- Logging Errors for Future Reference
- Creating Custom Error Messages
- Conclusion
- FAQ
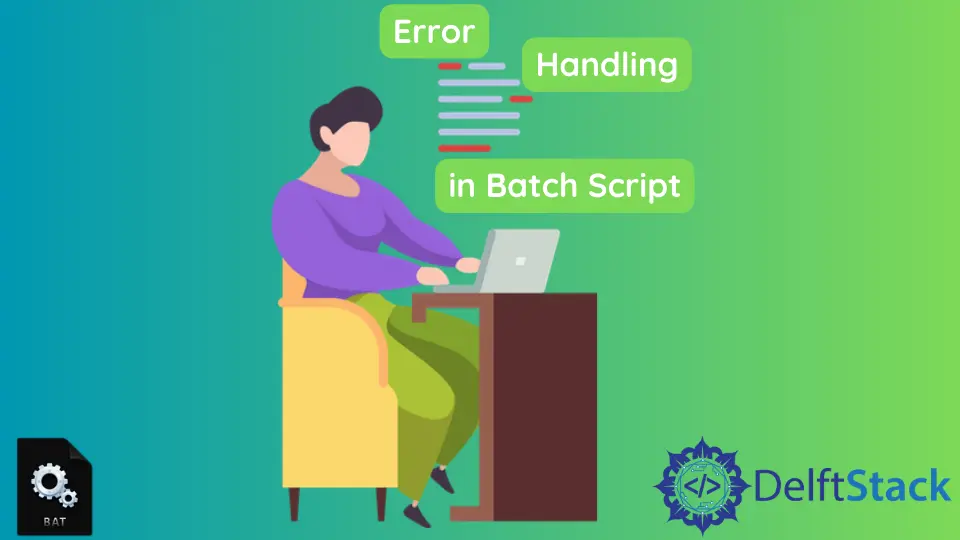
In the world of scripting, error handling is a crucial aspect that often gets overlooked. When working with Batch Scripts, especially in environments like Git, understanding how to effectively manage errors can save you time and prevent potential disasters.
This tutorial will delve into the essential techniques for implementing error handling in Batch Scripts. We will explore how to gracefully handle errors, log them for future reference, and ensure your scripts run smoothly even when unexpected issues arise. By the end of this guide, you’ll be well-equipped to tackle error handling in your Batch Scripts, enhancing their reliability and robustness.
Understanding Error Levels in Batch Scripts
Before we dive into practical implementations, it’s essential to understand how error levels work in Batch Scripts. Each command executed in a Batch file returns an exit code, known as an error level. The exit code signifies whether the command was successful or if an error occurred. By convention, an exit code of 0
indicates success, while any non-zero value indicates an error.
You can access the error level using the built-in variable ERRORLEVEL
. This variable allows you to check the result of the last command executed. Here’s a simple example:
@echo off
echo Running a command...
some_command_here
if ERRORLEVEL 1 (
echo An error occurred!
) else (
echo Command completed successfully.
)
In this script, we run a command and check its result using ERRORLEVEL
. If the command fails (returns a non-zero exit code), we print an error message. This method is simple yet effective for basic error handling.
Using Conditional Statements for Error Handling
Conditional statements are a powerful tool for managing errors in Batch Scripts. By using IF
, you can create specific responses based on the error level. This way, you can tailor your error handling to the needs of your script.
Here’s an example demonstrating this approach:
@echo off
echo Starting process...
git clone https://github.com/example/repo.git
if ERRORLEVEL 1 (
echo Failed to clone repository. Please check the URL and your network connection.
) else (
echo Repository cloned successfully.
)
In this script, we attempt to clone a Git repository. If the git clone
command fails, we provide a user-friendly error message, guiding the user to check the repository URL and their network connection. This method not only informs the user of the failure but also gives them actionable steps to resolve the issue.
The flexibility of conditional statements allows you to handle various error scenarios effectively, making your scripts more resilient.
Logging Errors for Future Reference
Logging errors is an excellent practice that can help you troubleshoot issues later. By capturing error messages and relevant information, you can analyze them to improve your scripts over time. In Batch Scripts, you can redirect error output to a log file.
Here’s how you can implement error logging:
@echo off
set LOGFILE=error_log.txt
echo Starting process... >> %LOGFILE%
git clone https://github.com/example/repo.git >> %LOGFILE% 2>&1
if ERRORLEVEL 1 (
echo An error occurred. Check %LOGFILE% for details.
) else (
echo Repository cloned successfully.
)
In this example, we redirect both standard output and error output to a log file named error_log.txt
. If the git clone
command fails, we inform the user to check the log file for more details. This approach allows you to keep a record of errors that can be invaluable for debugging and improving your scripts.
Logging errors not only aids in troubleshooting but also helps in maintaining a history of issues encountered, making it easier to track recurring problems.
Creating Custom Error Messages
Sometimes, the default error messages provided by commands may not be informative enough for users. In such cases, you can create custom error messages that are more descriptive and helpful. This approach enhances user experience and provides clarity on what went wrong.
Here’s an example of how to implement custom error messages:
@echo off
echo Starting process...
git clone https://github.com/example/repo.git
if ERRORLEVEL 1 (
echo Error: Unable to clone the repository.
echo Possible reasons:
echo 1. The repository URL is incorrect.
echo 2. You do not have permission to access this repository.
echo 3. Network issues may be preventing the connection.
) else (
echo Repository cloned successfully.
)
In this script, if the git clone
command fails, we provide a detailed explanation of possible reasons for the failure. This not only informs the user of the error but also equips them with knowledge to troubleshoot effectively.
Custom error messages can significantly improve the usability of your scripts, making them more intuitive and user-friendly.
Conclusion
Implementing error handling in Batch Scripts is essential for creating robust and reliable scripts, especially when dealing with Git commands. By understanding error levels, using conditional statements, logging errors, and creating custom messages, you can enhance the user experience and make your scripts more resilient. These practices not only help in troubleshooting but also contribute to the overall quality of your coding efforts. As you continue to refine your Batch Scripts, keep these error handling techniques in mind to ensure smooth and efficient operations.
FAQ
-
What is the purpose of error handling in Batch Scripts?
Error handling helps manage unexpected issues that may arise during script execution, ensuring the script runs smoothly and provides useful feedback. -
How can I check the exit code of a command in Batch Scripts?
You can check the exit code using theERRORLEVEL
variable immediately after executing a command. -
Can I log errors in Batch Scripts?
Yes, you can redirect error output to a log file using the>>
operator and2>&1
to capture both standard and error outputs. -
What are custom error messages, and why are they useful?
Custom error messages provide more descriptive feedback to users, helping them understand what went wrong and how to fix it. -
Is error handling necessary in every script?
While not mandatory, implementing error handling is highly recommended as it improves the reliability and user-friendliness of scripts.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn