How to Fix Arduino Exit Status 1 Error
- Understanding the Exit Status 1 Error
- Check Your Board and Port Settings
- Verify Library Installation
- Check for Syntax Errors
- Clean Up Your Sketch
- Conclusion
- FAQ
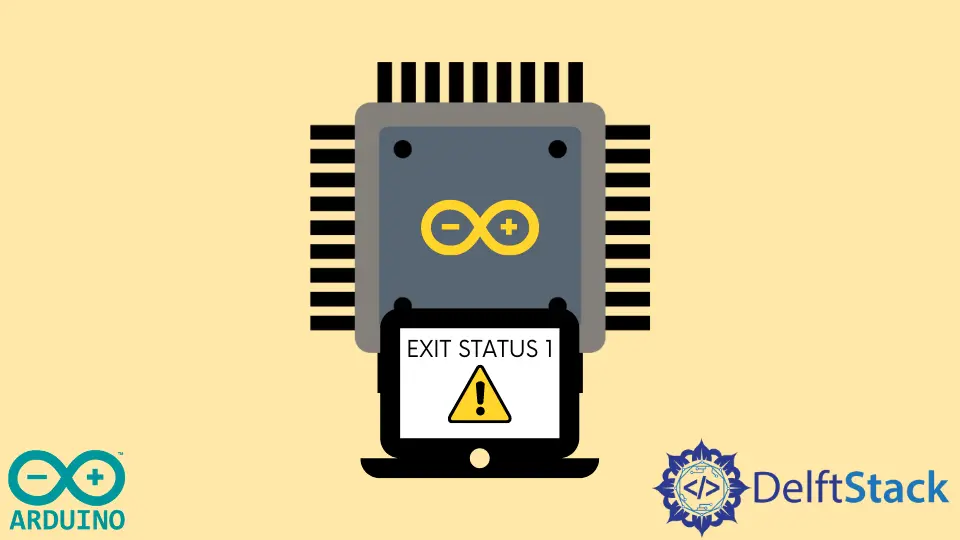
When working with Arduino, encountering the “exit status 1” error can be frustrating, especially for beginners. This compilation error usually indicates that something went wrong during the upload process, but pinpointing the exact cause can be tricky.
In this tutorial, we will dive into the common reasons behind this error and provide effective solutions to help you troubleshoot and resolve it. Whether you’re dealing with missing libraries, incorrect board settings, or syntax errors in your code, we’ve got you covered. Let’s get started on fixing that exit status 1 error and getting your Arduino project back on track!
Understanding the Exit Status 1 Error
Before we jump into the solutions, it’s essential to understand what the exit status 1 error means. This error usually occurs during the compilation phase of your Arduino sketch. It indicates that the compiler encountered an issue that prevented it from successfully creating the executable file for your Arduino board. The reasons can vary widely, from simple syntax mistakes in your code to missing libraries or incorrect board configurations. Being aware of these potential pitfalls can save you time and frustration as you troubleshoot your project.
For those interested in refining their skills, understanding how to program Arduino with C++ can enhance the way you approach coding with Arduino.
Check Your Board and Port Settings
One of the most common causes of the exit status 1 error is incorrect board or port settings in the Arduino IDE. If your IDE is not configured to recognize the board you are using, it will lead to compilation issues. Here’s how to check and correct these settings:
- Open the Arduino IDE.
- Navigate to the “Tools” menu.
- Select the correct board from the “Board” submenu.
- Ensure the correct port is selected under the “Port” submenu.
After adjusting these settings, try uploading your sketch again to see if the error persists.
Verify Library Installation
Another frequent culprit behind the exit status 1 error is missing or improperly installed libraries. If your code relies on specific libraries that are not installed or are outdated, the compilation will fail. Here’s how to verify and install the necessary libraries:
- Go to the “Sketch” menu.
- Select “Include Library,” then click on “Manage Libraries.”
- Use the search bar to find the libraries you need.
- Install or update the libraries as necessary.
After ensuring all required libraries are installed and updated, try compiling your sketch again. If you need to clean up libraries that are no longer in use, learn how to delete Arduino libraries from Arduino IDE.
Check for Syntax Errors
Syntax errors in your code can also trigger the exit status 1 error. Even a small typo can cause the compiler to fail. To identify and fix these errors, follow these steps:
- Carefully review your code for any typos or incorrect syntax.
- Pay attention to missing semicolons, mismatched brackets, and incorrect variable names.
- Use the Arduino IDE’s built-in error messages to help locate the problem.
Here’s a simple example of a common syntax error and how to correct it:
void setup() {
Serial.begin(9600)
}
void loop() {
Serial.println("Hello, World!");
}
In the code above, the missing semicolon after Serial.begin(9600)
will cause a compilation error.
Output:
error: expected ';' before '}' token
To fix it, simply add the missing semicolon:
void setup() {
Serial.begin(9600);
}
void loop() {
Serial.println("Hello, World!");
}
Now, the code should compile without any issues.
Clean Up Your Sketch
Sometimes, the exit status 1 error can be caused by leftover code or unused variables in your sketch. Cleaning up your code can help eliminate potential issues that might be causing the error. Here’s how to do it:
- Remove any unused variables or functions.
- Comment out or delete code segments that are not necessary for your current project.
- Organize your code for better readability, making it easier to spot errors.
By streamlining your sketch, you not only improve its performance but also reduce the chances of encountering the exit status 1 error.
Conclusion
In summary, the Arduino exit status 1 error can stem from various issues, including incorrect board settings, missing libraries, syntax errors, and code clutter. By following the troubleshooting steps outlined in this article, you can effectively diagnose and resolve the error, allowing you to continue with your Arduino projects seamlessly. Remember, patience is key when debugging, and with practice, you’ll become more adept at identifying and fixing these common issues. Happy coding!
FAQ
-
what does exit status 1 mean in Arduino?
Exit status 1 indicates that there was a compilation error in your Arduino sketch, preventing it from uploading successfully. -
how can I check if my libraries are properly installed?
You can check your libraries by going to the “Sketch” menu, selecting “Include Library,” and then clicking on “Manage Libraries” to see installed libraries. -
how do I fix syntax errors in my Arduino code?
Carefully review your code for typos, missing semicolons, or mismatched brackets, and correct any mistakes you find. -
can cleaning up my code help fix the exit status 1 error?
Yes, removing unused variables and organizing your code can help eliminate potential issues that may cause the error. -
what should I do if the error persists after trying all solutions?
If the error continues, consider seeking help from online forums or communities dedicated to Arduino programming, where you can share your code and get feedback.