Arduino ADC
- What is Arduino ADC?
- How to Use Arduino ADC
- Practical Applications of Arduino ADC
- Troubleshooting Common Issues with Arduino ADC
- Conclusion
- FAQ
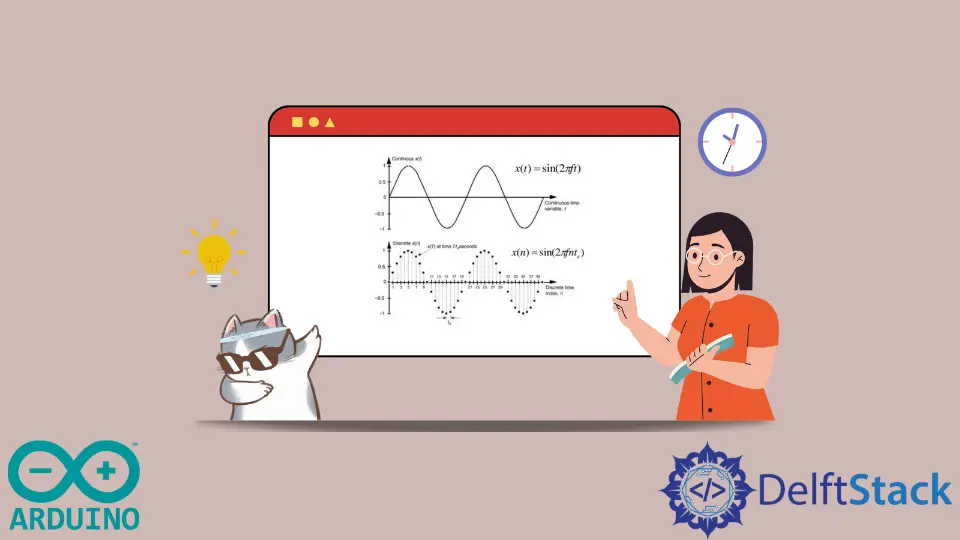
In the world of electronics and programming, the Arduino platform stands out as a versatile tool for hobbyists and professionals alike. One of its remarkable features is the ability to read analog signals through its analog pins and convert them into digital values. This process, known as Analog to Digital Conversion (ADC), is crucial for interfacing with various sensors and devices that output analog signals. Whether you’re measuring temperature, light intensity, or voltage levels, understanding how to effectively utilize Arduino’s ADC capabilities can significantly enhance your projects.
In this article, we’ll explore the fundamentals of Arduino ADC, providing insights, methods, and practical examples to help you master this essential skill.
What is Arduino ADC?
Arduino ADC refers to the built-in capability of Arduino boards to convert analog signals into digital data. Most Arduino boards, such as the Arduino Uno, come equipped with several analog input pins, typically denoted as A0 to A5. These pins can read voltage levels ranging from 0 to 5 volts and convert them into a digital representation. The ADC in Arduino uses a 10-bit resolution, meaning it can produce values from 0 to 1023, corresponding to the input voltage range. This allows for precise readings of varying analog signals, making it an invaluable tool for many electronic projects.
How to Use Arduino ADC
To effectively use the ADC feature in Arduino, you need to follow a simple process. First, connect your analog sensor or device to one of the analog input pins. Then, write a program that reads the analog value and processes it as needed. Below is a straightforward example of how to read an analog signal from a potentiometer and print the value to the Serial Monitor.
void setup() {
Serial.begin(9600);
}
void loop() {
int sensorValue = analogRead(A0);
Serial.println(sensorValue);
delay(500);
}
In this code, we start by initializing the Serial communication at a baud rate of 9600. In the loop function, we read the analog value from pin A0 using the analogRead()
function and store it in the variable sensorValue
. The value is then printed to the Serial Monitor every half a second. This simple setup allows you to visualize the changes in the analog signal as you adjust the potentiometer.
To further enhance your understanding of Arduino programming, consider exploring How to Program Arduino With C++, which provides insights into using C++ for Arduino projects.
Output:
512
510
515
520
This output represents the varying readings from the potentiometer, showcasing how the ADC effectively translates the analog signal into digital values.
Practical Applications of Arduino ADC
Understanding Arduino ADC opens up a world of possibilities for various applications. From measuring environmental conditions to controlling motors based on sensor input, the potential is vast. For instance, you can create a light-sensitive LED that brightens or dims based on the ambient light conditions. Here’s how you can implement this using a photoresistor.
const int photoResistorPin = A1;
const int ledPin = 9;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
int lightLevel = analogRead(photoResistorPin);
int ledBrightness = map(lightLevel, 0, 1023, 0, 255);
analogWrite(ledPin, ledBrightness);
delay(100);
}
In this example, we read the light level from a photoresistor connected to pin A1. The map()
function scales the analog value (0 to 1023) to a suitable range for PWM output (0 to 255) to control the brightness of an LED connected to pin 9. As the ambient light changes, the LED’s brightness adjusts accordingly, demonstrating a practical application of the Arduino ADC.
For more advanced applications, you might want to learn How to Make a Counter in Arduino, which can be useful in various projects that involve counting events or cycles.
Output:
LED Brightness: 128
LED Brightness: 255
LED Brightness: 63
This output illustrates how the LED brightness varies in response to changing light levels, showcasing the real-time capabilities of the Arduino ADC.
Troubleshooting Common Issues with Arduino ADC
While working with Arduino ADC, you may encounter some common issues. Here are a few troubleshooting tips to help you overcome these challenges and ensure accurate readings.
-
Noise in Readings: If you notice fluctuations in your readings, consider adding a capacitor between the analog input pin and ground to filter out noise.
-
Incorrect Connections: Double-check your wiring. Ensure that your sensor is correctly connected to the appropriate analog pin and that there are no loose connections.
-
Voltage Reference: By default, Arduino uses 5V as the reference voltage. If you’re working with sensors that output a different voltage range, you may need to adjust the reference voltage using the
analogReference()
function.
- Sampling Rate: The ADC samples the input signal at a certain rate. If your signal changes rapidly, consider using averaging techniques to smooth out the readings.
By addressing these issues, you can enhance the reliability and accuracy of your analog readings, ensuring your projects perform as intended. To further refine your skills in manipulating pins, check out How to Toggle Pin in Arduino, which explains pin manipulation techniques in detail.
Conclusion
In conclusion, mastering Arduino ADC is essential for anyone looking to delve into the world of electronics and programming. The ability to read and convert analog signals into digital data opens up numerous possibilities for innovative projects. Whether you are measuring environmental variables or creating interactive devices, understanding the principles of ADC will significantly enhance your skills. With the examples and troubleshooting tips provided, you’re now equipped to harness the full potential of Arduino’s analog capabilities. Dive in and start experimenting with your own projects today!
FAQ
-
What is the maximum voltage that Arduino ADC can read?
The maximum voltage that Arduino ADC can read is 5 volts. -
How many bits does Arduino ADC use for conversion?
Arduino ADC uses a 10-bit resolution for conversion, allowing for values from 0 to 1023. -
Can I change the reference voltage for ADC in Arduino?
Yes, you can change the reference voltage using theanalogReference()
function. -
What types of sensors can I use with Arduino ADC?
You can use various sensors, such as potentiometers, photoresistors, temperature sensors, and more. -
How can I improve the accuracy of my ADC readings?
To improve accuracy, you can filter noise, ensure proper connections, and use averaging techniques.