Session Storage in Angular
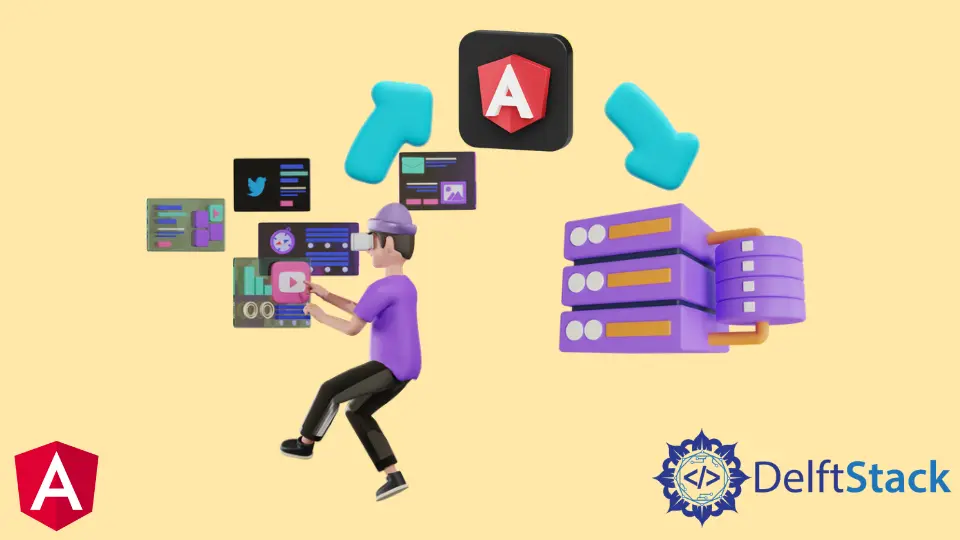
We will learn about sessionStorage
and how to save, get, delete specific, or delete all data in sessionStorage
in Angular.
Session Storage in Angular
sessionStorage
keeps a separate storage area for every given origin available for the duration of the page session. sessionStorage
is flushed when the tab or window is closed.
Saving Data Using sessionStorage
To store data in sessionStorage
, we will create a function saveData()
inside the app.component.ts
file.
# angular
saveData(){
}
Inside the saveData
function we will use setItem
to store name in the sessionStorage
.
# angular
saveData() {
sessionStorage.setItem('name', 'Rana Hasnain');
}
Our function to store data in sessionStorage
is complete.
We need to edit our app.component.html
file and add a button with the click
event to the saveData()
function. So, our code in app.component.html
will look like below.
# angular
<hello name="{{ name }}"></hello>
<button (click)="saveData()">Save Data in Session Storage</button>
Output:
When we click on the button, it will save data in the sessionStorage
with the key
of the name
.
Output:
Get Data From the sessionStorage
We will discuss how to get the stored data in the sessionStorage
and display it.
We will create a getData()
function in app.component.ts
.
# angular
getData(){
}
Inside the getData()
function we will use getItem
to get our desired data based on key
from the sessionStorage
.
# angular
getData(){
return sessionStorage.getItem('name');
}
We are now done with our function, and we need to display the data we got from the getData()
function.
We will edit app.component.html
and replace the name
attribute of the hello
tag. So our code in app.component.html
will look like below.
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()">Save Data in Session Storage</button>
Output:
Deleting Specific Data From the sessionStorage
We will discuss deleting specific data based on the key
from the sessionStorage
on button click.
First, we will create the removeData()
function in the app.component.ts
.
# angular
removeData(){
}
We will use the removeItem
to remove specific data from the sessionStorage
based on key
. We will also add some extra data in the sessionStorage
, which we can remove for this example.
Our code in app.component.ts
will look like below.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
title = 'Session Storage in Angular 12 By Husnain';
name = 'Angular ' + VERSION.major;
saveData() {
sessionStorage.setItem('name', 'Rana Hasnain');
sessionStorage.setItem('location', 'Pakistan');
}
getData() {
return sessionStorage.getItem('name');
}
removeData() {
sessionStorage.removeItem('location');
}
}
In the above code, we have added extra data with the key
location in the sessionStorage
, removed on button click.
We need to create a button in app.component.html
, which will call our removeData()
function. So app.component.html
will look like this.
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()" class="btn">Save Data in Session Storage</button>
<button (click)="removeData()" class="btn">Remove Location</button>
We will also style buttons to look better and add the following code in the app.component.css
.
# angular
p {
font-family: Lato;
}
.btn{
margin-right: 15px;
padding: 10px 15px;
background-color: blueviolet;
color: white;
border: none;
border-radius: 5px;
}
Output:
We will click on the Save Data
button to save the sessionStorage
location. After that, we will click on the Remove Location
button to remove location data from sessionStorage
.
Delete All Data From the sessionStorage
We will discuss deleting all data from the sessionStorage
in Angular.
We will create the deleteData()
function in the app.component.ts
.
# angular
deleteData(){
}
We will use clear
to delete all data from sessionStorage
. So our code will look like below.
# angular
deleteData(){
sessionStorage.clear();
}
We need to create a button in app.component.html
to call the deleteData()
function. So our code will look like below.
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()" class="btn">Save Data in Session Storage</button>
<button (click)="removeData()" class="btn">Remove Location</button>
<button (click)="deleteData()" class="btn">Clear All</button>
Output:
Final result:
Our files will look like below in the end.
app.component.ts
:
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
title = 'Session Storage in Angular 12 By Husnain';
name = 'Angular ' + VERSION.major;
saveData() {
sessionStorage.setItem('name', 'Rana Hasnain');
sessionStorage.setItem('location', 'Pakistan');
}
getData() {
return sessionStorage.getItem('name');
}
removeData() {
sessionStorage.removeItem('location');
}
deleteData() {
sessionStorage.clear();
}
}
app.component.html
:
# angular
<hello name="{{ getData() }}"></hello>
<button (click)="saveData()" class="btn">Save Data in Session Storage</button>
<button (click)="removeData()" class="btn">Remove Location</button>
<button (click)="deleteData()" class="btn">Clear All</button>
app.component.css
:
# angular
p {
font-family: Lato;
}
.btn {
margin-right: 15px;
padding: 10px 15px;
background-color: blueviolet;
color: white;
border: none;
border-radius: 5px;
}
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn