How to Select a Value on a Dropdown List in Angular
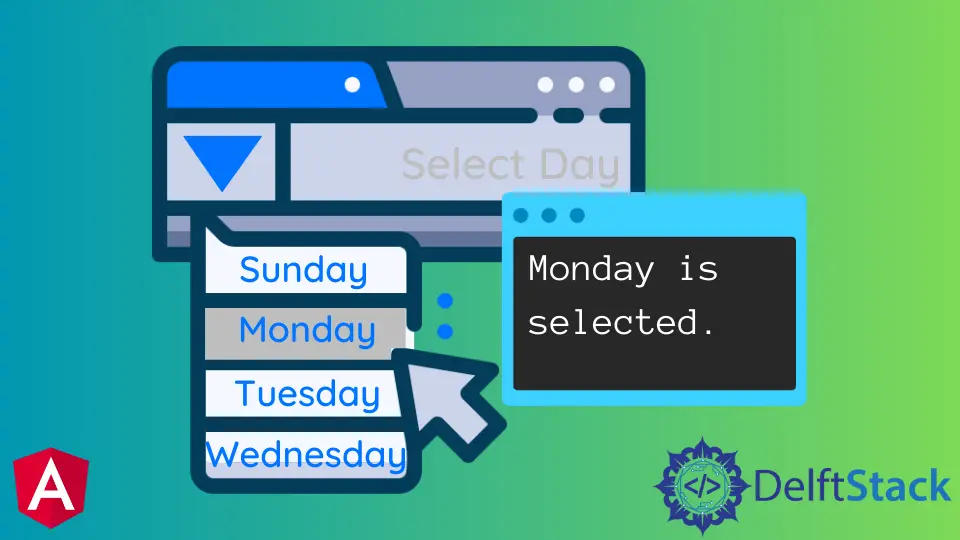
In Angular 2, the ngOptions
directive is used to create a dropdown list, the ngFor
directive to iterate through dropdown values, and the ngif
to select a value.
This article will demonstrate how to select the dropdown list in Angular.
Steps to Select a Value on a Dropdown List in Angular 2
Selecting a value from a dropdown list is one of the most common tasks in a web application.
The following steps are needed to select a value on the dropdown list in Angular 2.
-
Open the Code Editor.
-
Create a dropdown list.
-
Add a button to open the dropdown list to add items.
-
Add an empty
div
element with anng-for
directive to display all items in a table format. -
Add a button that will call functions to close and save changes made in this view when clicked on.
-
Add
ngif
directive to select the right option.
Let’s write an example to apply the steps mentioned above.
TypeScript code (App.component.ts
):
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
selectedOption = 0;
actions = [{ id: 0, name: 'Select Country' },
{ id: 1, name: 'Netherland' },
{ id: 2, name: 'England' },
{ id: 3, name: 'Scotland' },
{ id: 4, name: 'Germany' },
{ id: 5, name: 'Canada' },
{ id: 6, name: 'Mexico' },
{ id: 7, name: 'Spain' }]
}
A selector and template are the two fields of the @Component
metadata object. The selector field specifies a selector for an HTML element that represents the component.
The template instructs Angular on how to display the view of this component. You can either add a URL to an HTML file or put HTML here.
We used the URL to point to the HTML template in this example. After that, we wrote the options to display in the output terminal.
TypeScript code:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
The @NgModule
defines the metadata for the module. BrowserModule
keeps track of important app service providers.
It also includes standard directives like ngIf
and ngFor
, which appear and may be used in any module’s component templates right away. The ngModel
directive requires the FormsModule
module.
The declarations list specifies the app element and the root element, which is at the beginning of the app component hierarchy. The declarations array contains a list of the modules, commands, and pipes.
HTML code (App.component.html
):
<h2>Example of how to select the value in the dropdown list in Angular 2</h2>
<select id="select-menu" [(ngModel)]="selectedOption" class="bx--text-input" required name="actionSelection" >
<option *ngFor="let action of actions" [value]="action.id">{{action.name}}</option>
</select>
<div>
<div *ngIf="selectedOption==1">
<div>Netherland is selected</div>
</div>
<div *ngIf="selectedOption==2">
<div>England is selected</div>
</div>
<div *ngIf="selectedOption==3">
<div>Scotland is selected</div>
</div>
<div *ngIf="selectedOption==4">
<div>Germany is selected</div>
</div>
<div *ngIf="selectedOption==5">
<div>Canada is selected</div>
</div>
<div *ngIf="selectedOption==6">
<div>Mexico is selected</div>
</div>
<div *ngIf="selectedOption==7">
<div>Spain is selected</div>
</div>
</div>
In this code, we used two directives, ngfor
and ngif
.
The ngfor
directive is used to create a list of items. This can be a simple array or an object with some properties converted into an array.
The ngfor
directive is typically used to iterate over an array and render different DOM elements for each item in the list. Here, the purpose of ngfor
is to create a list of countries.
Secondly, we used ngif
, a directive in Angular that creates an if-else
statement. It can also be used with ngElse
, ngSwitch
, and ngShow/ngHide
.
The directive only renders the HTML code when the expression evaluates to true. If it evaluates to false, it will not render anything.
Here, ngif
only will display the selected country.
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook