在 Angular 的下拉列表中选择一个值
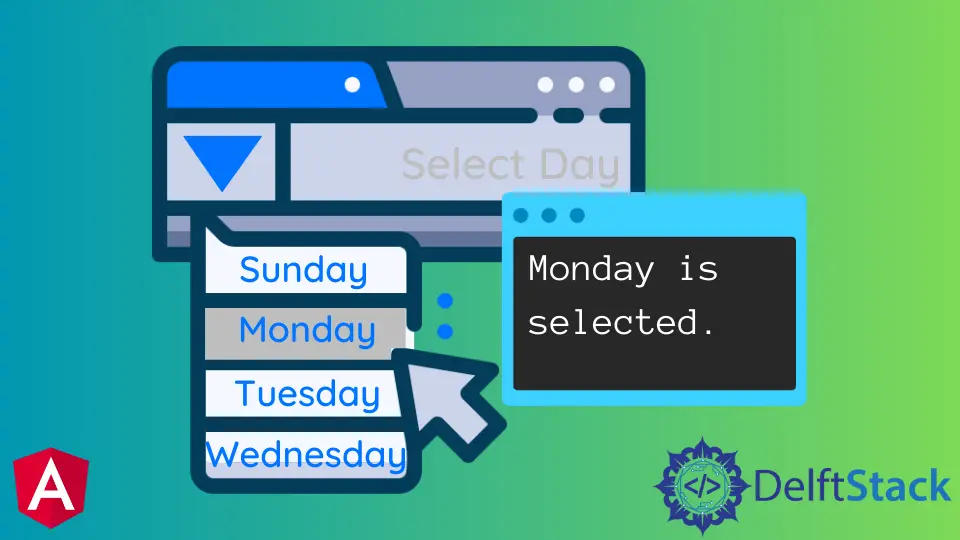
在 Angular 2 中,ngOptions
指令用于创建下拉列表,ngFor
指令用于迭代下拉值,ngif
用于选择一个值。本文将演示如何在 Angular 中选择下拉列表。
在 Angular 2 中的下拉列表中选择值的步骤
从下拉列表中选择一个值是 Web 应用程序中最常见的任务之一。
在 Angular 2 的下拉列表中选择一个值需要以下步骤。
-
打开代码编辑器。
-
创建一个下拉列表。
-
添加按钮以打开下拉列表以添加项目。
-
添加一个带有
ng-for
指令的空div
元素,以表格格式显示所有项目。 -
添加一个按钮,该按钮将调用函数以在单击时关闭并保存在此视图中所做的更改。
-
添加
ngif
指令以选择正确的选项。
让我们编写一个示例来应用上述步骤。
TypeScript 代码(App.component.ts
):
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
selectedOption = 0;
actions = [{ id: 0, name: 'Select Country' },
{ id: 1, name: 'Netherland' },
{ id: 2, name: 'England' },
{ id: 3, name: 'Scotland' },
{ id: 4, name: 'Germany' },
{ id: 5, name: 'Canada' },
{ id: 6, name: 'Mexico' },
{ id: 7, name: 'Spain' }]
}
选择器和模板是 @Component
元数据对象的两个字段。选择器字段为代表组件的 HTML 元素指定选择器。
该模板指示 Angular 如何显示该组件的视图。你可以将 URL 添加到 HTML 文件或将 HTML 放在这里。
在此示例中,我们使用 URL 指向 HTML 模板。之后,我们编写了要在输出终端中显示的选项。
TypeScript 代码:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
@NgModule
定义了模块的元数据。BrowserModule
跟踪重要的应用服务提供商。
它还包括标准指令,如 ngIf
和 ngFor
,它们会立即出现并可以在任何模块的组件模板中使用。ngModel
指令需要 FormsModule
模块。
声明列表指定应用程序元素和根元素,它位于应用程序组件层次结构的开头。声明数组包含模块、命令和管道的列表。
HTML 代码(App.component.html
):
<h2>Example of how to select the value in the dropdown list in Angular 2</h2>
<select id="select-menu" [(ngModel)]="selectedOption" class="bx--text-input" required name="actionSelection" >
<option *ngFor="let action of actions" [value]="action.id">{{action.name}}</option>
</select>
<div>
<div *ngIf="selectedOption==1">
<div>Netherland is selected</div>
</div>
<div *ngIf="selectedOption==2">
<div>England is selected</div>
</div>
<div *ngIf="selectedOption==3">
<div>Scotland is selected</div>
</div>
<div *ngIf="selectedOption==4">
<div>Germany is selected</div>
</div>
<div *ngIf="selectedOption==5">
<div>Canada is selected</div>
</div>
<div *ngIf="selectedOption==6">
<div>Mexico is selected</div>
</div>
<div *ngIf="selectedOption==7">
<div>Spain is selected</div>
</div>
</div>
在这段代码中,我们使用了两个指令,ngfor
和 ngif
。
ngfor
指令用于创建项目列表。这可以是一个简单的数组,也可以是一个将某些属性转换为数组的对象。
ngfor
指令通常用于遍历数组并为列表中的每个项目呈现不同的 DOM 元素。在这里,ngfor
的目的是创建一个国家列表。
其次,我们使用了 ngif
,这是 Angular 中创建 if-else
语句的指令。它也可以与 ngElse
、ngSwitch
和 ngShow/ngHide
一起使用。
该指令仅在表达式计算结果为 true 时呈现 HTML 代码。如果计算结果为 false,则不会渲染任何内容。
在这里,ngif
只会显示所选国家。
点击这里查看上面代码的演示。
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook