How to Restrict Custom Directives in AngularJS
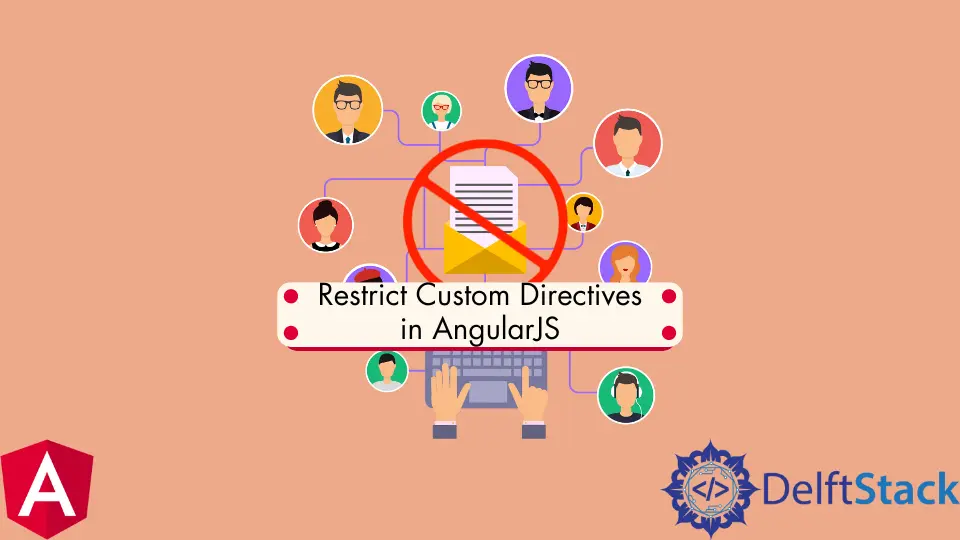
Directives are a special type of AngularJS component used to extend the functionality of an HTML element or create new features with a rich behavior that is not available using pure HTML.
AngularJS includes pre-built directives like ngBind
, ngModel
, and ngClass
. The Angular framework uses these directives to instruct the browser on new HTML tags.
HTML tags and comments and CSS classes and properties are examples of directives.
Restriction directive limits the access to the DOM element from external HTML codes by adding an attribute to it.
This article will discuss how to limit custom directives in AngularJS.
AngularJS Directive Restrict A
vs. E
The restrict
attribute tells Angular how to create a new directive that is only one letter long. It can have four values: A
, C
, E
, M
, or a combination of those values, such as EA, ME, etc.
Each one is significant in its way.
The restricted values and their meaning are listed below.
E
denotes that it can only be used as an element.A
denotes that it can only be used as an attribute.C
is only valid as a class attribute.M
only applies to comments.
Suppose the directive’s name is hello
. The following are the types and their usage.
A = <div hello></div>
C = <div class="hello"></div>
E = <hello data="Demo"></hello>
M = <!--directive:hello -->
When developing a component in charge of the template, use an element. This is a common scenario when you’re constructing a Domain-Specific Language for aspects of your template.
When adding new functionality to an existing element, use an attribute.
You can apply multiple directives to the same DOM node when leveraging an attribute rather than an element.
This is especially useful for form controls, where you can use additional properties to highlight, disable, or add labels without having to enclose the component of a lot of tags.
Example (JavaScript):
var app = angular.module('myapp', []);
app.controller('MainCtrl', function($scope) {
$scope.name = 'World';
});
app.directive('helloWorld',function(){
return {
replace:true,
restrict: 'E',
template: '<h2>Hello World</h2>'
}
});
Example (HTML):
<!DOCTYPE html>
<html ng-app="myapp">
<head>
<meta charset="utf-8" />
<script>document.write('<base href="' + document.location + '" />');</script>
<script data-require="angular.js@1.2.x" src="http://code.angularjs.org/1.2.7/angular.js" data-semver="1.2.7"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<hello-world></hello-world>
<div hello-world></div>
<div class="hello-world"></div>
</body>
</html>
Click here to check the live demonstration of the code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook