How to Implement the ngStyle Directive in Angular
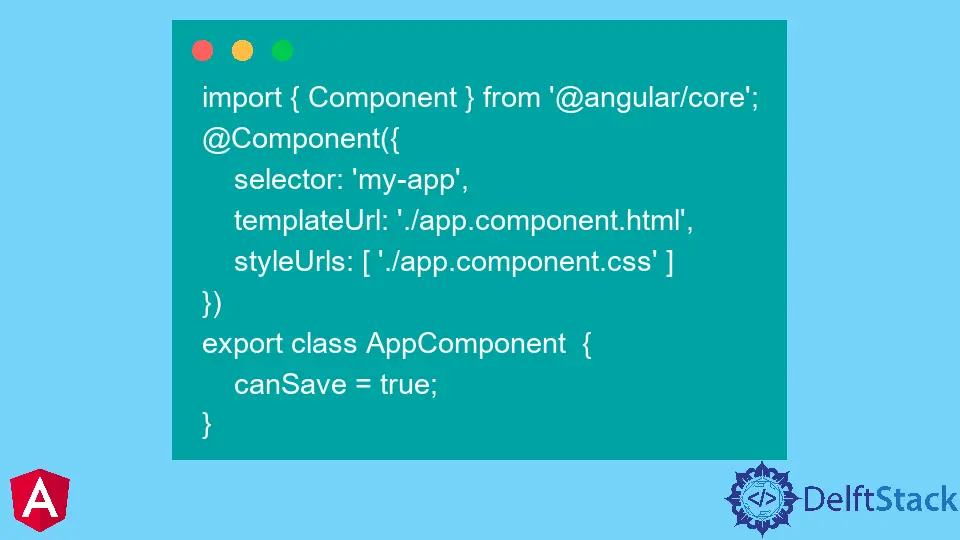
Angular style directive allows you to apply styles to your HTML elements. The ngStyle
directive is a variation of the style directive in Angular.
This article will discuss the usage of the ngStyle
directive in Angular.
the ngStyle
Directive in Angular
The ngStyle
directive applies styles to an HTML element using CSS selectors. This directive works with the Angular templating engine that allows you to have more control over how an HTML document should be rendered.
The ngStyle
directive is one of the most popular directives in Angular. It can be used for various purposes, such as applying custom CSS classes and styling form elements.
Syntax:
<element [ngStyle]="{'styleNames': styleExp}">...</element>
The value for the ngStyle
attribute directive can be any conditional binary or ternary expression. Furthermore, it handles any method call defined within the controller, which the controller can control according to the needs.
We can also use the ngStyle
directive to manage View and CSS styling based on the model value so that ngStyle
is run and the CSS styling is updated when the model value changes.
Steps to Implement the ngStyle
Directive in Angular
The ngStyle
directive is straightforward and can be applied to any element in the DOM. You need to follow a few steps before using it on your website.
-
Add
ngStyle
as a dependency in your Angular application module. -
Configure the styles with CSS classes or inline styles (CSS code).
-
Use the
ngStyle
directive in your HTML template. -
Use the
ngClass
attribute to specify which CSS classes should be applied to an element when it matches a given condition.
Let’s discuss an example using the steps mentioned above.
TypeScript code:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
canSave = true;
}
HTML code:
<h2>Example of ng-style in Angular</h2>
<button
[ngStyle] = "{
'backgroundColor' : canSave ? 'pink' : 'blue',
'color' : canSave ? 'red' : 'yellow',
'margin-left': '200px',
'padding': '30px',
'margin-top': '200px'
}"
>A simple button</button>
In the example, we created a simple button and gave margin, padding, font, and background color with the help of the ngStyle
directive.
Click here to check the demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook