How to Implement Pagination in Angular
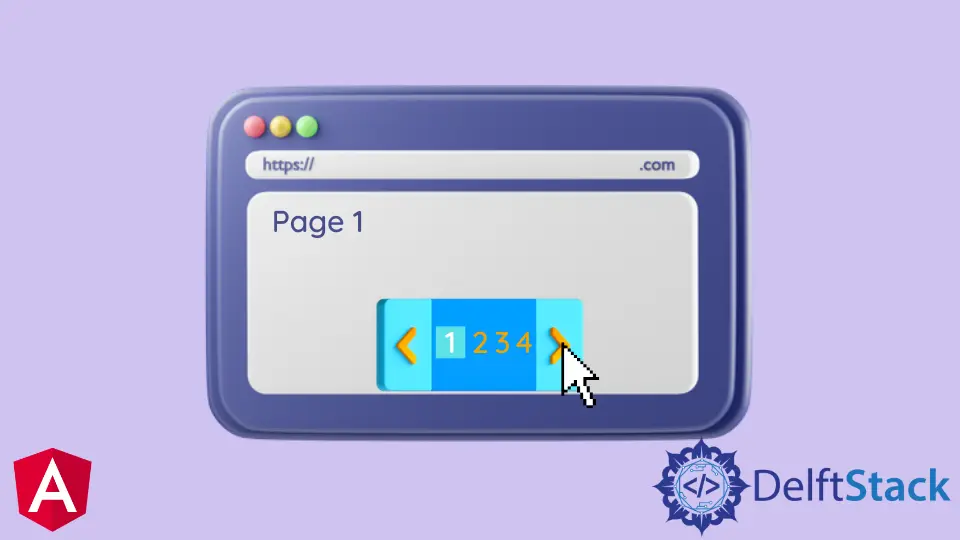
We will introduce pagination in Angular and which library is best to implement pagination, and how to use it.
Pagination in Angular
When we have a dataset of more than 1000 or 10,000 items, we cannot display all items on one page or at once because it takes a lot of time and memory to load a complete dataset. The best way to display many datasets is by using pagination in Angular.
Many libraries can be used for pagination in Angular. But in this tutorial, we will use ngx-pagination
, which is easy and simple to implement.
Installation of ngx-pagination
To install an ngx-pagination
library, we need to open the terminal in our project and execute the following command.
# angular CLI
npm install ngx-pagination
After our library is installed, we will import NgxPaginationModule
from ngx-pagination
in the app.module.ts
file. We will also import NgxPaginationModule
in @NgModule
.
So, our code in app.module.ts
will look like below.
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { NgxPaginationModule } from 'ngx-pagination';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
@NgModule({
imports: [ BrowserModule, FormsModule, NgxPaginationModule ],
declarations: [ AppComponent, HelloComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
We will create an array with some data and a number
variable that will store the number of the page we are on. Our code in app.component.ts
will look like below.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
pages: number = 1;
dataset: any[] = ['1','2','3','4','5','6','7','8','9','10'];
}
We will create a view to display our dataset. The app.component.html
will look like below.
# angular
<div>
<h2
*ngFor="
let data of dataset | paginate: { itemsPerPage: 2, currentPage: pages }
"
>
{{ data }}
</h2>
</div>
<pagination-controls (pageChange)="pages = $event"></pagination-controls>
Let’s write some CSS code to make it look better in app.component.css
.
# angular
div{
text-align: center;
padding: 10px;
}
h2{
border: 1px solid black;
padding: 10px;
}
Output:
It will work like this.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn