Equivalent of OnChange in Angular 2
-
Introduction to
ngOnChanges
in Angular 2 -
Use
ngOnChanges
as the Equivalent ofOnChange
in Angular 2 -
Use Cases for
ngOnChanges
in Angular 2 - Pitfalls and Best Practices
- Conclusion
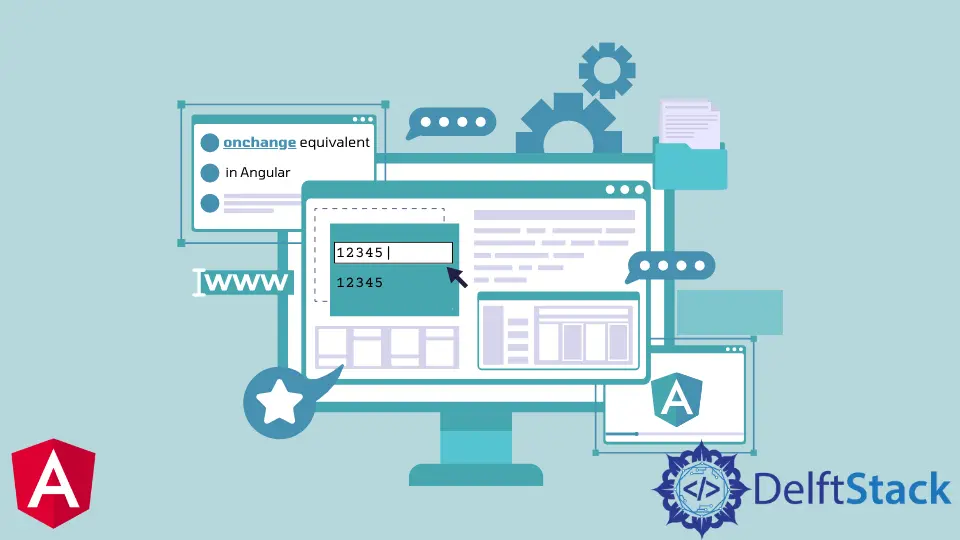
Angular event bindings are a great way to respond to any DOM event. They allow us to bind our code with the element’s native click or input events and execute them when they occur.
Angular 2 introduced a powerful lifecycle hook called ngOnChanges
, which plays a crucial role in managing component state and responding to changes in input properties. This lifecycle hook provides developers with a structured way to detect and handle changes in a component’s input bindings.
The OnChanges
can be used to monitor changes in input parameters in an Angular project, and we can use the OnChanges
equivalent in Angular 2 for input fields, button clicks, list items, or even scrolling events. In this article, we’ll delve into the details of ngOnChanges
, explore its functionality, and learn how to effectively use it in Angular 2 applications.
Introduction to ngOnChanges
in Angular 2
Angular 2.0 has two-way data binding. Any changes to the input field will be reflected immediately on the UI and vice versa.
ngOnChanges
is one of the lifecycle hooks provided by Angular 2. It is called whenever one or more input properties of a component change. This hook receives a SimpleChanges
object that contains the previous and current values of the input properties.
Syntax:
ngOnChanges(changes: SimpleChanges): void {
// Handle changes here
}
Here, changes
is an object where the keys are the names of the input properties, and the values are instances of SimpleChange
.
The argument SimpleChanges
is passed to the method ngOnChanges()
, which returns the new and previous input values following modifications.
When using the input user object’s data type, ngOnChanges()
is only invoked when the object’s link in the parent component changes. The ngOnChanges()
method will not execute if we merely alter the values of attributes of an input user object.
the SimpleChanges
Object
The SimpleChanges
object is a map of property names to instances of SimpleChange
. Each SimpleChange
instance provides information about the previous and current values of an input property.
A SimpleChange
object has two properties, the previousValue
and the currentValue
. The previousValue
property holds the previous value of the input property, and the currentValue
property contains the current value of the input property.
Use ngOnChanges
as the Equivalent of OnChange
in Angular 2
The OnChanges
event is a life cycle hook that executes when the value of the input changes. The ngModel
directive binds an input or textarea
element to a property on the current scope, and it replaces the HTML5 onchange
event, which is supported in Angular 2.
The ngModelChange
event is fired when a model updates and its value changes. It can be fired by passing an expression to the ngModel
directive or binding it to input.
Example Code - HTML:
<div>
<input [value]="first" (change)="changeFn($event)">
<p>{{ first }}</p>
<input [ngModel]="second" (ngModelChange)="modelChangeFn($event)">
<p>{{ second }}</p>
</div>
This code creates a simple UI with two input elements and paragraphs to display their corresponding values.
The first input element uses property binding to set its initial value, and the change
event triggers a function when the value changes. The second input element uses two-way binding with ngModel
, and the ngModelChange
event triggers another function when the value changes.
Example Code - TypeScript:
This Angular component, named AppComponent
, defines two properties (first
and second
) with initial values of '1'
and '2'
respectively. It also includes two functions: changeFn
, which updates first
with the value from a target element, and modelChangeFn
, which updates second
with a provided string.
These functions are bound to events in the component’s associated template, allowing for dynamic interaction between the UI and the component class.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
first = '1';
second = '2';
changeFn(abc) {
this.first = abc.target.value;
}
modelChangeFn(abc: string) {
this.second = abc;
}
}
Output:
changeFn()
will only be executed when the user blurs the input. On the other hand, when users enter, paste, or edit the value of an input, ModelChangeFn()
listens for the event and sets the object.
Click here to run the above code.
Use Cases for ngOnChanges
in Angular 2
Reacting to Input Changes
One of the most common use cases for ngOnChanges
is to respond to changes in input properties. This is especially useful when you need to perform specific actions based on the new values of the inputs.
For example, imagine a component that displays user information. If the user data changes, you might want to update the display accordingly.
ngOnChanges(changes: SimpleChanges): void {
if (changes.userData) {
this.updateDisplay(changes.userData.currentValue);
}
}
This code is within the ngOnChanges
lifecycle hook of an Angular component. It checks if there has been a change in the userData
input property, and if so, it calls a method (updateDisplay
) with the updated value.
This allows the component to react to changes in its input properties and update its display accordingly.
Synchronization With Child Components
ngOnChanges
can be used to keep child components in sync with their parent. By detecting changes in parent inputs, you can pass down updated values to child components as needed.
ngOnChanges(changes: SimpleChanges): void {
if (changes.data) {
this.childComponentInput = changes.data.currentValue;
}
}
This code is within the ngOnChanges
lifecycle hook of an Angular component. It checks if there has been a change in the data
input property, and if so, it assigns the new value to childComponentInput
.
This allows the component to keep childComponentInput
in sync with the data
input property.
Performing Calculations
You can use ngOnChanges
to recalculate values based on changes in input properties. This is particularly useful for components that depend on dynamic data.
ngOnChanges(changes: SimpleChanges): void {
if (changes.radius) {
this.calculateArea();
}
}
This code is within the ngOnChanges
lifecycle hook of an Angular component. It checks if there has been a change in the radius
input property, and if so, it triggers the calculation of the area.
Pitfalls and Best Practices
While ngOnChanges
is a powerful tool, it’s important to use it judiciously to avoid unnecessary computations and potential performance issues.
Avoid Complex Logic
It’s generally recommended to keep the logic within ngOnChanges
as simple as possible. Complex computations or operations that involve heavy processing should be delegated to other methods or services.
Avoid Side Effects
Try to limit the side effects of ngOnChanges
. Ideally, this lifecycle hook should be used for tasks directly related to input property changes.
Avoid performing unrelated tasks within this hook.
Be Mindful of Asynchronous Operations
Keep in mind that ngOnChanges
is synchronous and does not account for asynchronous operations. If you need to perform asynchronous tasks based on input changes, consider using other Angular features like RxJS
or async
pipes.
Conclusion
ngOnChanges
is a vital lifecycle hook in Angular 2 that enables developers to respond to changes in input properties in a structured and efficient manner. By understanding its usage and best practices, you can leverage this hook to build more dynamic and responsive Angular applications.
Remember to use ngOnChanges
judiciously, keeping the logic within it focused on handling input property changes. With careful implementation, you can enhance the reactivity and robustness of your Angular 2 components.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook